How to create Dividend Payout Ratio Calculator Card using JavaScript & Tailwind CSS ?
Last Updated :
12 Mar, 2024
A Dividend Payout Ratio Calculator is a web-based tool that helps users calculate the percentage of a company’s earnings that are paid out as dividends to shareholders. The dividend payout ratio is a financial metric that measures the proportion of earnings that are distributed to shareholders as dividends. It is calculated by dividing the total dividends paid by the company by its net income. The calculator is styled using Tailwind CSS, a utility-first CSS framework, which ensures a responsive and visually appealing design.
Approach to create Dividend Payout Ratio Calculator Card:
- Begin with the basic HTML structure, including the
<!DOCTYPE html>
declaration, <html>
, <head>
, and <body>
tags. Import Tailwind CSS for styling by adding a <script>
tag in the <head>
section. - Create a container
<div>
with Tailwind CSS classes for styling. Inside this container, include elements for the app title, input fields for the dividends paid and net income, a button for calculating the dividend payout ratio, and a <div>
for displaying the result. - Use JavaScript to handle the logic of calculating the dividend payout ratio based on the input values. Add an event listener to the calculate button. When the button is clicked, the script should retrieve the values from the input fields, perform the calculation, and display the result. If any field is empty, display an error message.
- Utilize Tailwind CSS classes to style the app container, input fields, button, and result
<div>
. Customize the colors, fonts, and layout to create an appealing visual design for the Dividend Payout Ratio Calculator. - Ensure that the Dividend Payout Ratio Calculator is responsive and works well on different screen sizes. Use Tailwind CSS classes for responsive design, such as
sm:
, md:
, and lg:
, to adjust the layout and styling based on the screen size.
Example: Implementation to design DPR calculator.
HTML<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Dividend Payout Ratio Calculator</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body class="bg-gray-100">
<div class="flex justify-center items-center h-screen">
<div class="max-w-md w-full bg-white p-8 rounded-lg
shadow-lg border-2 border-green-500">
<h1 class="text-3xl font-bold text-center mb-8">
Dividend Payout Ratio Calculator
</h1>
<div class="mb-4">
<label for="dividends" class="block text-gray-700">
Dividends Paid:
</label>
<input type="number"
id="dividends"
name="dividends"
class="w-full border border-gray-300 rounded-md px-3
py-2 focus:outline-none focus:border-blue-500"
placeholder="Enter total dividends paid">
</div>
<div class="mb-4">
<label for="netIncome"
class="block text-gray-700">
Net Income:
</label>
<input type="number" id="netIncome" name="netIncome"
class="w-full border border-gray-300 rounded-md px-3
py-2 focus:outline-none focus:border-blue-500"
placeholder="Enter net income">
</div>
<div class="mb-8">
<button id="calculateButton"
class="w-full bg-blue-500 text-white rounded-md
py-2 px-4 hover:bg-blue-600 focus:outline-none">
Calculate Dividend Payout Ratio
</button>
</div>
<div id="payoutRatioResult"
class="text-lg font-semibold text-center"></div>
<div id="errorMessage"
class="text-red-500 text-sm mt-4 hidden">
Please fill in all fields.
</div>
</div>
</div>
<script>
const calculateButton = document.getElementById('calculateButton');
calculateButton.addEventListener('click', () => {
const dividends = parseFloat(document.getElementById('dividends')
.value);
const netIncome = parseFloat(document.getElementById('netIncome')
.value);
if (!dividends || !netIncome) {
document.getElementById('errorMessage')
.classList
.remove('hidden');
return;
}
const payoutRatio = (dividends / netIncome * 100).toFixed(2);
document.getElementById('payoutRatioResult').textContent =
`Dividend Payout Ratio: ${payoutRatio}%`;
document.getElementById('errorMessage').classList.add('hidden');
});
</script>
</body>
</html>
Output:
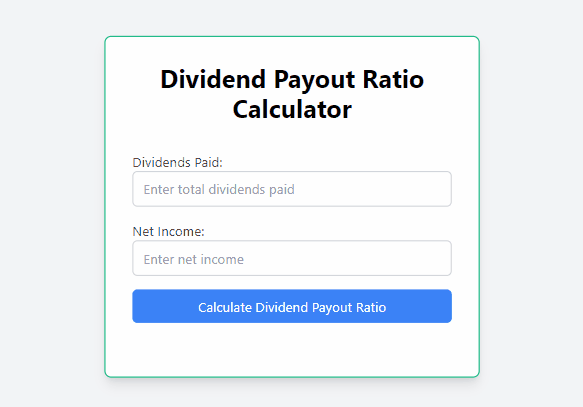
Share your thoughts in the comments
Please Login to comment...