How to Show and Hide Password in React Native ?
Last Updated :
12 Sep, 2023
In this article, we’ll see how we can add password show and hide features in React Native applications.
In mobile apps, users often need to toggle password visibility for better user experience and accuracy. React Native simplifies this with the `SecureTextEntry` prop in the `TextInput` component, enabling easy implementation of password show/hide functionality.
Prerequisites:
Approach:
- Create a React Native component with a password input field and a toggle button/icon.
- Maintain a state variable to track the visibility of the password.
- Use the
SecureTextEntry
prop of the TextInput
component to toggle the visibility of the password.
- Implement an event handler for the toggle button/icon to update the state variable.
- Toggle the value of the
SecureTextEntry
prop based on the state variable to show or hide the password.
Step 1: Set Up the Development Environment​
Install Expo CLI globally by running this command:
npm install -g expo-cli​
Step 2: Create React Native Application With Expo CLI
Create a new react native project with Expo CLI by using this command:
expo init showhide-passowrd
Step 3: ​Navigate to project directory by using this command:
cd showhide-password
Project Structure:
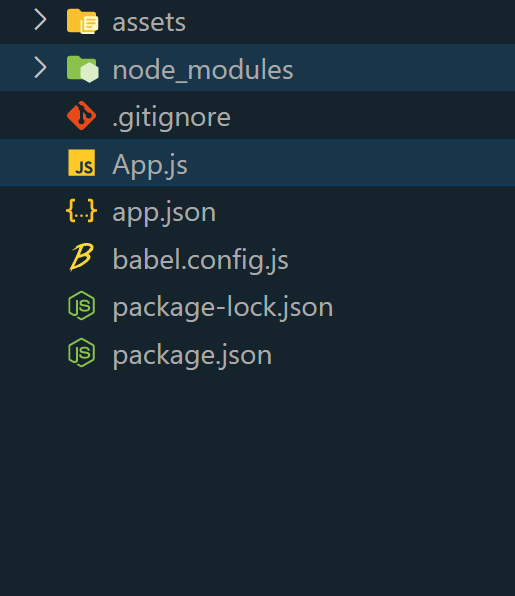
Step 4: Install required dependency
- Install the @expo/vector-icons package with the command:
npm install @expo/vector-icons
Example:
Javascript
import React, { useState } from 'react' ;
import { TextInput, View, StyleSheet, Text,
SafeAreaView } from 'react-native' ;
import { MaterialCommunityIcons } from '@expo/vector-icons' ;
const App = () => {
const [password, setPassword] = useState( '' );
const [showPassword, setShowPassword] = useState( false );
const toggleShowPassword = () => {
setShowPassword(!showPassword);
};
return (
<SafeAreaView style={styles.mainContainer}>
<Text style={styles.heading}>
Geeksforgeeks || Password Show and Hide
</Text>
<View style={styles.container}>
<TextInput
secureTextEntry={!showPassword}
value={password}
onChangeText={setPassword}
style={styles.input}
placeholder= "Enter Password"
placeholderTextColor= "#aaa"
/>
<MaterialCommunityIcons
name={showPassword ? 'eye-off' : 'eye' }
size={24}
color= "#aaa"
style={styles.icon}
onPress={toggleShowPassword}
/>
</View>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
mainContainer: {
marginTop: 70,
margin: 40,
},
container: {
flexDirection: 'row' ,
alignItems: 'center' ,
justifyContent: 'center' ,
backgroundColor: '#f3f3f3' ,
borderRadius: 8,
paddingHorizontal: 14,
},
input: {
flex: 1,
color: '#333' ,
paddingVertical: 10,
paddingRight: 10,
fontSize: 16,
},
icon: {
marginLeft: 10,
},
heading: {
alignItems: 'center' ,
fontSize: 20,
color: 'green' ,
marginBottom: 20,
},
});
export default App;
|
Steps to run: To run the react native application, open the Terminal/CMD and enter the following command:
npx expo start
To run on Android:
npx react-native run-android
To run on ios:
npx react-native run-ios
Output:
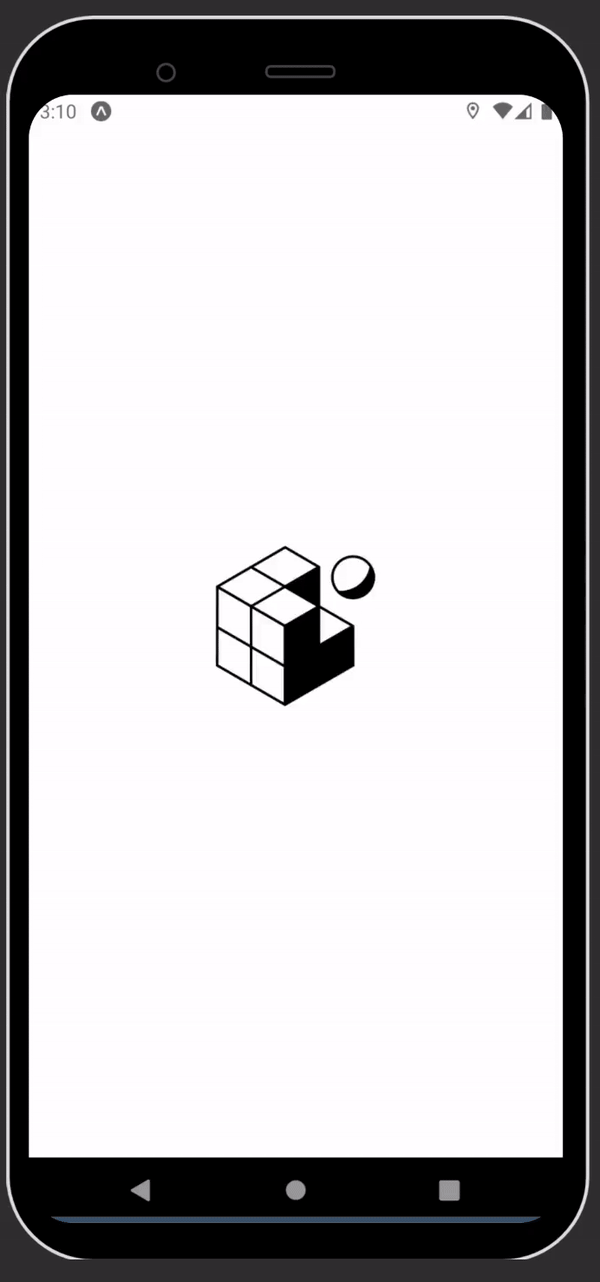
How to Show and Hide Password in React Native
Share your thoughts in the comments
Please Login to comment...