How To Create And Use .env Files In Python
Last Updated :
23 Feb, 2024
In Python, a .env file is commonly used to store configuration settings, API keys, and other sensitive information. It is a plain text file with key-value pairs, and the python-dotenv library is often used to load these variables into the environment. In this article, we will explore the detailed process of creating and using .env Files in Python.
What is .Env Files In Python?
A .env file in Python is a simple text file used to store configuration settings, environment variables, and other key-value pairs related to a Python project. These files typically contain sensitive information such as API keys, database credentials, or configuration settings. The contents of a .env file are not meant to be hardcoded in the source code but are instead loaded into the project’s environment during runtime. The python-dotenv library is commonly employed to read the contents of the .env file and set the environment variables, making it a convenient way to manage project configurations securely.
Installation
How To Create And Use .Env Files In Python?
Below is the complete procedure to create and use .Env files in Python:
Step 1: Create the .env file
In your project directory, create a file named “.env” at the root level to store configuration settings and sensitive information.
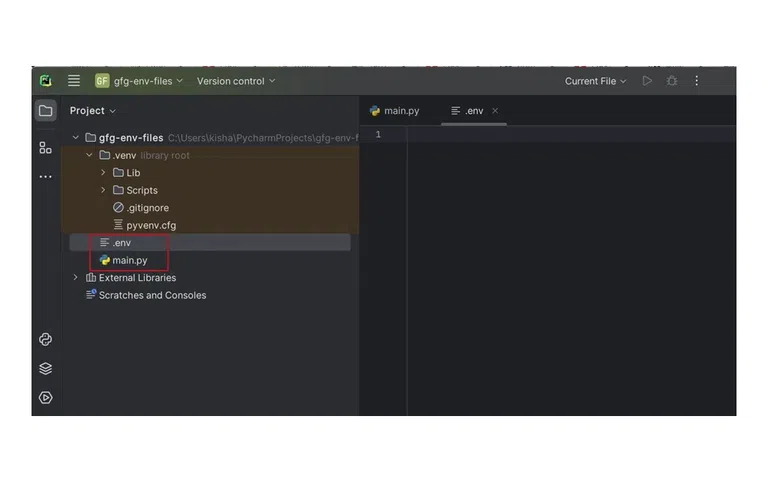
Step 2: Install the Module
Install the python-dotenv library by running the following command in your terminal or integrated terminal within your Python IDE (e.g., PyCharm):
pip install python-dotenv
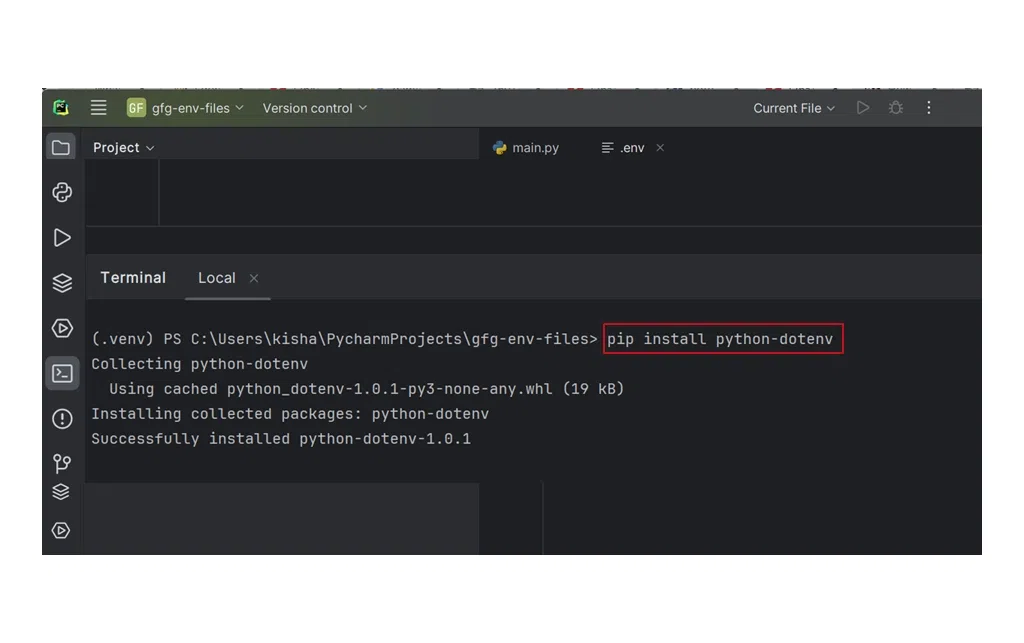
Step 3: Store Sensitive Data in .env File
In your .env file, define key-value pairs for your configuration settings. For example:
MY_KEY = "kishankaushik12353"
This file will store sensitive information and configuration settings.
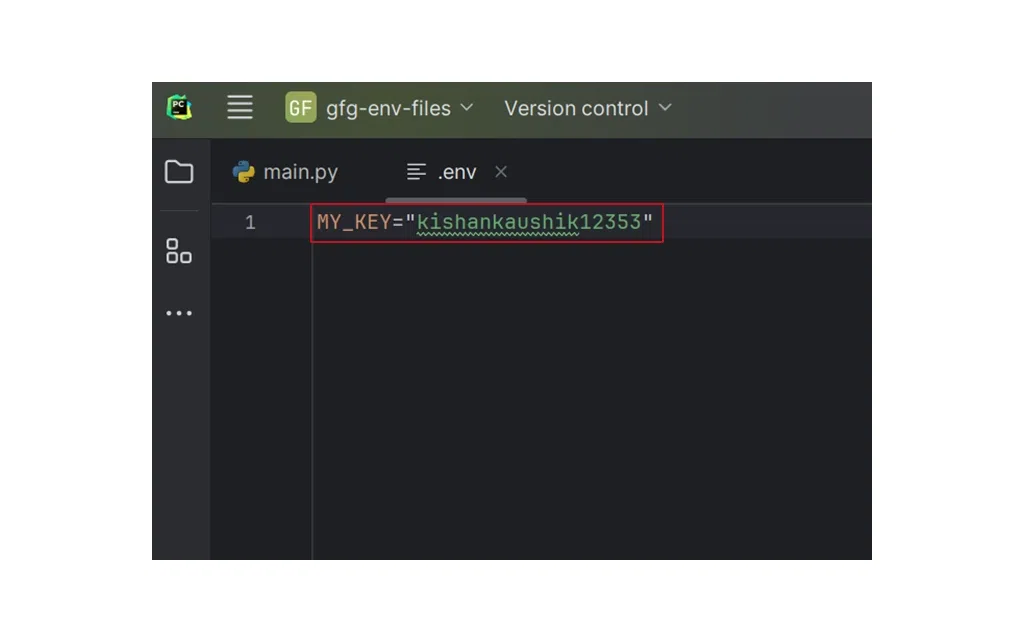
Step 4: Access the .env file
In your Python code, import the dotenv module, and use load_dotenv() to load variables from the .env file. Access the values using os.getenv(“KEY”) for each key-value pair defined in the .env file.
Python3
import os
from dotenv import load_dotenv, dotenv_values
load_dotenv()
print (os.getenv( "MY_KEY" ))
|
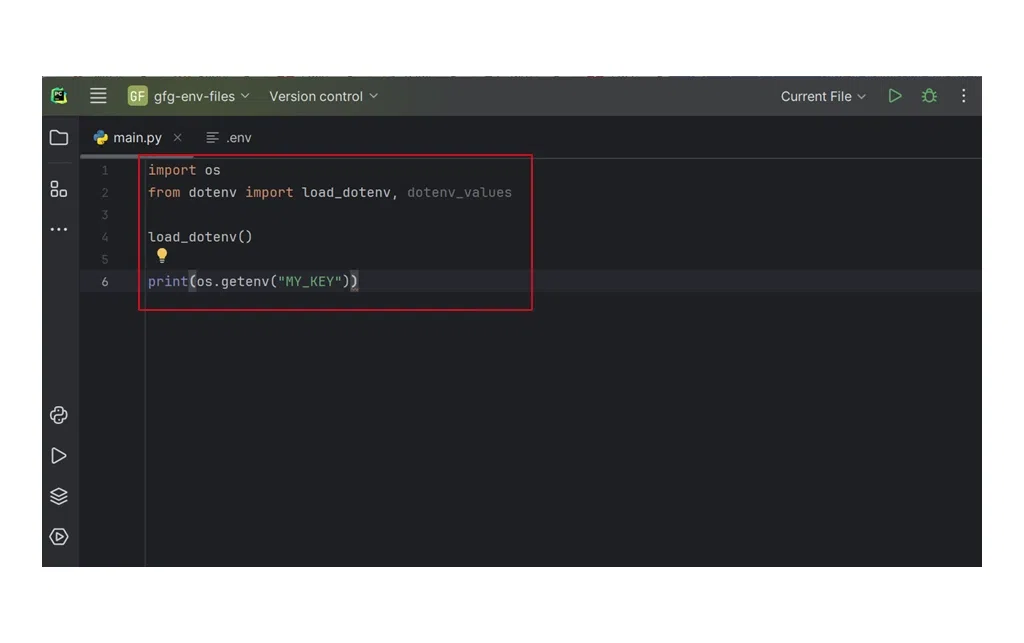
Step 5: Compile and Run the Code
Compile and run the Python code. The program will utilize the loaded environment variables from the .env file, and the output will display the value associated with the specified key, such as “MY_KEY,” demonstrating successful integration with the .env file.
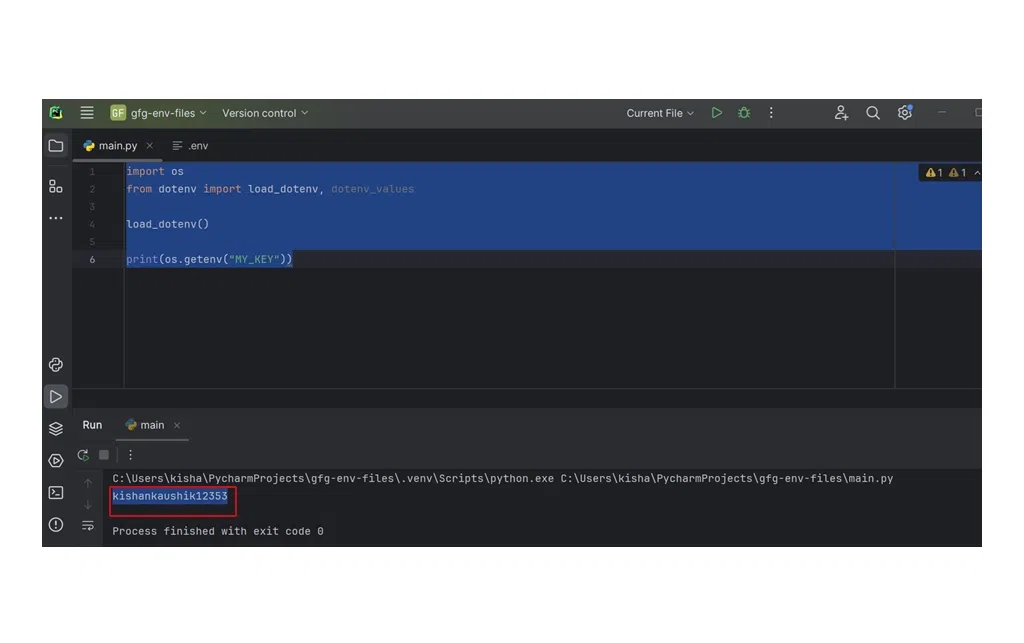
Conclusion
In conclusion, utilizing .env files in Python provides a secure and organized way to manage sensitive information and configuration settings. By using the python-dotenv library, developers can easily load variables into the project’s environment, enhancing flexibility across different environments. This practice ensures the separation of sensitive data from the codebase and enables a systematic approach for managing configurations in development, testing, and production. Incorporating .env files is a best practice for maintaining security and scalability in Python projects.
Share your thoughts in the comments
Please Login to comment...