How to create PDF files in Flask
Last Updated :
29 Sep, 2023
Whether it’s generating a report, exporting data, or creating catalogs, Python and the Flask web framework provide powerful tools to accomplish this task. In this article, we’ll explore how to generate both PDF files using Python and Flask.
Creating PDF files in Flask
Step 1: Installation
We are using Flask to create CSV output. Please refer:- Install Flask and Install Python for proper installation.
Step 2: Create a Virtual Environment
In this step, we will first create a virtual environment for our project. Please refer to create virtual environment for further information.
Step 3: Create Folders
Now, we will create a folder in the given format in the given picture below. We will create a static folder, and a templates folder, and inside the templates folder, create an index.html file. We will also create an app.py file where we will write our Python code.
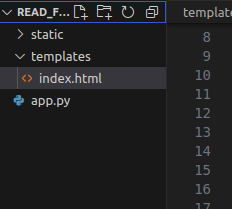
Step 4: Write HTML Code
In this step, we will create a file index.html and paste this code inside that file. In this code, we have created an HTML form, and when a person the book details. It is saved inside a pdf file.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >Generate PDF</ title >
</ head >
< body >
< h1 >Generate and Download PDF</ h1 >
< form method = "POST" action = "/generate-pdf" >
< label for = "title" >Title:</ label >
< input type = "text" id = "title" name = "title" required>< br >
< label for = "author" >Author:</ label >
< input type = "text" id = "author" name = "author" required>< br >
< label for = "publication_year" >Publication Year:</ label >
< input type = "number" id = "publication_year" name = "publication_year" required>< br >
< input type = "submit" value = "Add Book" >
</ form >
< p >< a href = "/generate-pdf" download>Download Book Catalog (PDF)</ a ></ p >
</ body >
</ html >
|
Step 5: Write Python Code
In this step, we will write our Flask logic in the form of Python code.
Import All Required Libraries: Import all required libraries and module like Flask, render_template, request, Response, send_file and other as shown in the code itself.
Python3
from flask import Flask, render_template, request, Response, send_file
from io import BytesIO
from reportlab.pdfgen import canvas
app = Flask(__name__)
user_data = []
|
Function for Default Route: In this function, when a user visits the root URL of the web application, Flask will execute the index() function, which, in turn, renders the ‘index.html’ template and sends the resulting HTML page as the response to the user’s web browser. Code implementation is given below in index() function.
Python3
@app .route( '/' )
def index():
return render_template( 'index.html' )
|
Function to Take Input Data from User: This function code handles requests to ‘/generate-pdf’, allows users to submit form data to collect book information, generates a PDF catalog from that data, and provides the PDF for download. Code is given below in generate_pdf() function.
Python3
@app .route( '/generate-pdf' , methods = [ 'GET' , 'POST' ])
def generate_pdf():
if request.method = = 'POST' :
title = request.form.get( 'title' )
author = request.form.get( 'author' )
publication_year = request.form.get( 'publication_year' )
if title and author and publication_year:
user_data.append({
'title' : title,
'author' : author,
'publication_year' : publication_year
})
pdf_file = generate_pdf_file()
return send_file(pdf_file, as_attachment = True , download_name = 'book_catalog.pdf' )
|
Function to Genrate PDF: This function code defines a function to create a PDF catalog of books using the ReportLab library and sets up a web application (presumably for serving this catalog). Code implementation is given below inside generate_pdf_file() function.
Python3
def generate_pdf_file():
buffer = BytesIO()
p = canvas.Canvas( buffer )
p.drawString( 100 , 750 , "Book Catalog" )
y = 700
for book in user_data:
p.drawString( 100 , y, f "Title: {book['title']}" )
p.drawString( 100 , y - 20 , f "Author: {book['author']}" )
p.drawString( 100 , y - 40 , f "Year: {book['publication_year']}" )
y - = 60
p.showPage()
p.save()
buffer .seek( 0 )
return buffer
if __name__ = = '__main__' :
app.run(debug = True )
|
Full Implementation of app.py file Python code
Python3
from flask import Flask, render_template, request, Response, send_file
from io import BytesIO
from reportlab.pdfgen import canvas
app = Flask(__name__)
user_data = []
@app .route( '/' )
def index():
return render_template( 'index.html' )
@app .route( '/generate-pdf' , methods = [ 'GET' , 'POST' ])
def generate_pdf():
if request.method = = 'POST' :
title = request.form.get( 'title' )
author = request.form.get( 'author' )
publication_year = request.form.get( 'publication_year' )
if title and author and publication_year:
user_data.append({
'title' : title,
'author' : author,
'publication_year' : publication_year
})
pdf_file = generate_pdf_file()
return send_file(pdf_file, as_attachment = True , download_name = 'book_catalog.pdf' )
def generate_pdf_file():
buffer = BytesIO()
p = canvas.Canvas( buffer )
p.drawString( 100 , 750 , "Book Catalog" )
y = 700
for book in user_data:
p.drawString( 100 , y, f "Title: {book['title']}" )
p.drawString( 100 , y - 20 , f "Author: {book['author']}" )
p.drawString( 100 , y - 40 , f "Year: {book['publication_year']}" )
y - = 60
p.showPage()
p.save()
buffer .seek( 0 )
return buffer
if __name__ = = '__main__' :
app.run(debug = True )
|
Step 6: Run the File
In this step, just run the file and you will see this form. Just fill this form and a click on Add Book button. Data will be added and then click on Download Book Catalog button to download the file in PDF format.
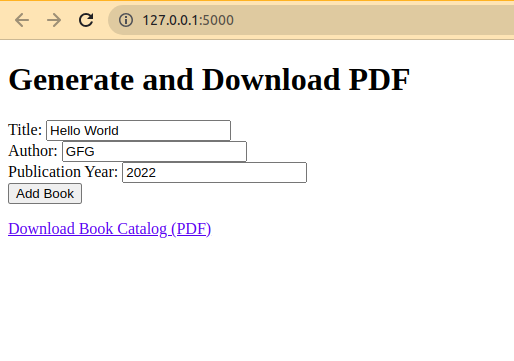
PDF File Output
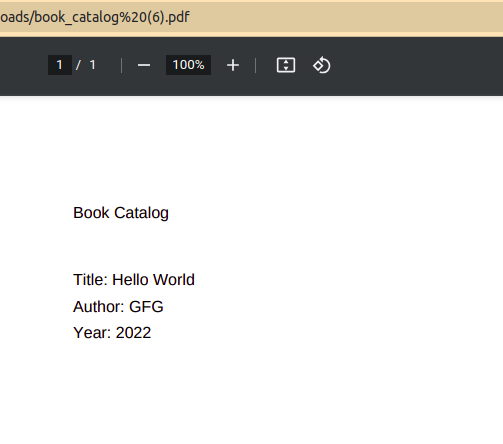
Share your thoughts in the comments
Please Login to comment...