How to create an image map in JavaScript ?
Last Updated :
27 Aug, 2021
An image map is nothing but an image that is broken into various hotspots and each hotspot will take you to a different file. Hotspots are nothing but clickable areas which we create on an image by using the <area> tag. This type of map is called a client-side image map as the map is embedded in the HTML itself.
Approach: Below is the step-by-step implementation of How to create an image map in JavaScript.
Step 1: The first step is to insert an image using the <img> tag. Here we will be using an additional attribute “usemap“. The value of the usemap must start with ‘#’ tag followed by the name of the map as written below.
Syntax:
<img src="map1.jpg" alt="Cocktails"
usemap="#mymap" width="600" height="400">
Step 2: We then create an image map using the tag <map>. This creates a map linked to the image using the required name attribute. The name attribute value must be the same as given in usemap attribute of <img> tag.
Syntax:
<map name="mymap">
Step 3: The different clickable areas are created using the tag <area>. We must be defining the shape of the area. The different shapes are rectangle, circle, and polygon. The coordinates of the area must also be given and href is the link that will be opened when the user clicks the area.
Syntax:
<area shape="rect" coords="x,y,x,y" href="">
<area shape="circle" coords="x,y,r" href="">
<area shape="poly" coords="" href="">
Step 4: Now for finding the coordinate of an image.
- Rectangle image will have the coordinates x1,y1,x2,y2 where x1,y1 are the coordinates of the top left corner and x2,y2 are the coordinates of the bottom right as shown in the image.
- Circle image will have the coordinates x,y,r where x,y are the coordinates of the center of the circle and r is the radius of the circle.
- Polygon image will have the coordinates x1,y1,x2,y2,x3,y3,x4,y4,,……. where x,y defines the coordinates of one of the corners of the image as shown in the image.
Example 1: In this example, we will simply create the image map of a rectangle and circle images.
HTML
<!DOCTYPE html>
< html >
< body >
< img
src =
alt = "Cocktails"
usemap = "#mymap"
width = "400"
height = "500" />
< map name = "mymap" >
< area
shape = "rect"
coords = "167,22,380,113"
alt = "Write"
onmouseover = "myFunction('Write for Us!!')"
onmouseout = "myFunction('')" />
< area
shape = "rect"
coords = "148,423,382,500"
alt = "Practice"
onmouseover = "myFunction('Practice and Learn')"
onmouseout = "myFunction('')" />
< area
shape = "circle"
coords = "115,260,40"
alt = "IDE"
onmouseover = "myFunction('IDE')"
onmouseout = "myFunction('')" />
</ map >
< h1 id = "info" ></ h1 >
< script >
function myFunction(name) {
document.getElementById("info").innerHTML = name;
}
</ script >
</ body >
</ html >
|
Output:
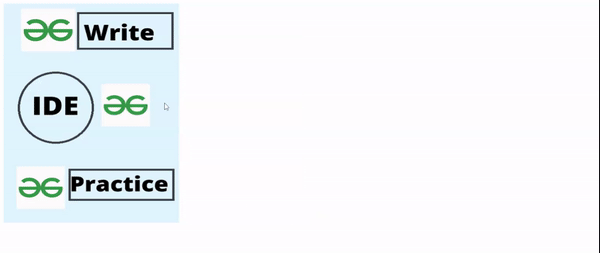
Example 2: In this example, we are considering the yellow-colored portion of the image as a polygon.
HTML
<!DOCTYPE html>
< html >
< body >
< img
src =
alt = "Polygon"
usemap = "#map1"
width = "600"
height = "433" />
< map name = "map1" >
< area
shape = "poly"
coords="152,244,160,180,251,133,368,123,495,117,551,185,
467,278,396,303,311,298,230,292,169,268"
alt = "Polygon"
onmouseover="myFunction('A Computer Science portal for geeks.
It contains well written, well thought and well
explained computer science and programming
articles')"
onmouseout = "myFunction('')"
/>
</ map >
< h1 id = "info" ></ h1 >
< script >
function myFunction(name) {
document.getElementById("info").innerHTML = name;
}
</ script >
</ body >
</ html >
|
Output:
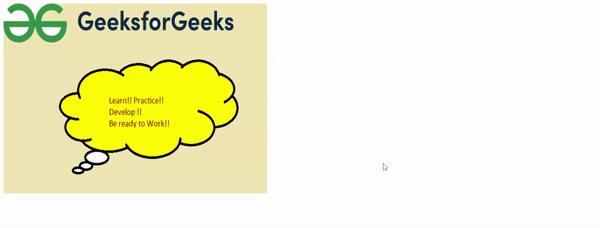
Share your thoughts in the comments
Please Login to comment...