Cryptocurrency App using ReactJS
Last Updated :
19 Jul, 2023
In this article, we are going to build a cryptocurrency app that lists all the available cryptos in the market. In this application user can see the current price, market cap, available supply of all the cryptocurrencies. The user can also search for an individual crypto coin and get information about it. We will convert the currency into INR by passing it in our API request.
Let us see how the final application will look like.
Approach: Our app contains two sections, one for searching the name of the cryptocurrency and the other for displaying all the available cryptocurrencies in the market. Initially, we will fetch all the data from the API and store it inside a state variable. Then we will map through all the data and display it in a table. Whenever a user searches for some specific crypto, we will filter that and display only the matching results.
Prerequisites: The pre-requisites for this project are:
Creating a React application and Module Installation:
Step 1: Create a react application by typing the following command in the terminal:
npx create-react-app crypto-app
Step 2: Now, go to the project folder i.e crypto-app by running the following command:
cd crypto-app
Step 3: Install Axios which is an npm package. It is a promise-based HTTP client for the browser and node.js.
npm install axios
Project Structure: It will look like this.
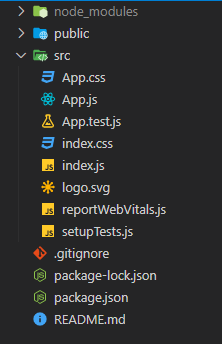
The updated dependencies in package.json file will look like:
"dependencies": {
"@testing-library/jest-dom": "^5.16.5",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"axios": "^1.4.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example: Write the following code in respective files
- App.js: This file contains the logic and structure of the project along with API call using axios
- App.css: This file contains the design of the project.
Javascript
import "./App.css" ;
import Axios from "axios" ;
import { useEffect, useState } from "react" ;
function App() {
const [search, setSearch] = useState( "" );
const [crypto, setCrypto] = useState([]);
useEffect(() => {
Axios.get(
`https:
).then((res) => {
setCrypto(res.data.coins);
});
}, []);
return (
<div className= "App" >
<h1>All Cryptocurrencies</h1>
<input
type= "text"
placeholder= "Search..."
onChange={(e) => {
setSearch(e.target.value);
}}
/>
<table>
<thead>
<tr>
<td>Rank</td>
<td>Name</td>
<td>Symbol</td>
<td>Market Cap</td>
<td>Price</td>
<td>Available Supply</td>
<td>Volume(24hrs)</td>
</tr>
</thead>
{ }
<tbody>
{ }
{crypto
.filter((val) => {
return val.name.toLowerCase().includes(search.toLowerCase());
})
.map((val, id) => {
return (
<>
<tr id={id}>
<td className= "rank" >{val.rank}</td>
<td className= "logo" >
<a href={val.websiteUrl}>
<img src={val.icon} alt= "logo" width= "30px" />
</a>
<p>{val.name}</p>
</td>
<td className= "symbol" >{val.symbol}</td>
<td>₹{val.marketCap}</td>
<td>₹{val.price.toFixed(2)}</td>
<td>{val.availableSupply}</td>
<td>{val.volume.toFixed(0)}</td>
</tr>
</>
);
})}
</tbody>
</table>
</div>
);
}
export default App;
|
CSS
.App {
min-height : 100 vh;
height : auto ;
display : flex;
flex- direction : column;
align-items: center ;
gap: 15px ;
padding : 40px ;
font-size : 16px ;
background-image : linear-gradient(to top , #dfe9f3 0% , white 100% );
}
h 1 {
color : forestgreen;
}
input {
padding-left : 5px ;
font-size : 20px ;
width : 250px ;
height : 30px ;
}
table {
width : 1000px ;
border-collapse : separate ;
border-spacing : 0 1em ;
}
thead {
background-color : rgb ( 44 , 44 , 44 );
color : white ;
text-align : center ;
}
tbody>tr {
text-align : right ;
}
.rank {
text-align : center ;
font-weight : bold ;
}
.logo {
display : flex;
justify- content : flex-start;
padding-left : 10% ;
gap: 10px ;
}
.symbol {
text-align : center ;
}
|
Steps to Run Application:
Step 1: Run the application using the following command from the root directory of the project:
npm start
Step 2: Now open your browser and go to http://localhost:3000/, you will see the output:
Output:
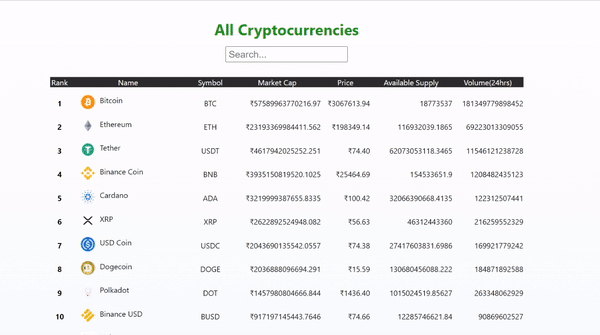
Share your thoughts in the comments
Please Login to comment...