Word and Letter Counter using React
Last Updated :
07 Mar, 2024
The word and letter counter is an application that counts the number of letters and words entered in text and give the number (count) of each in output. Each element present in the text will be considered a letter, and a group of letters with a space will be considered a word. Using this approach, we implement the counter.
Preview of Final Output:
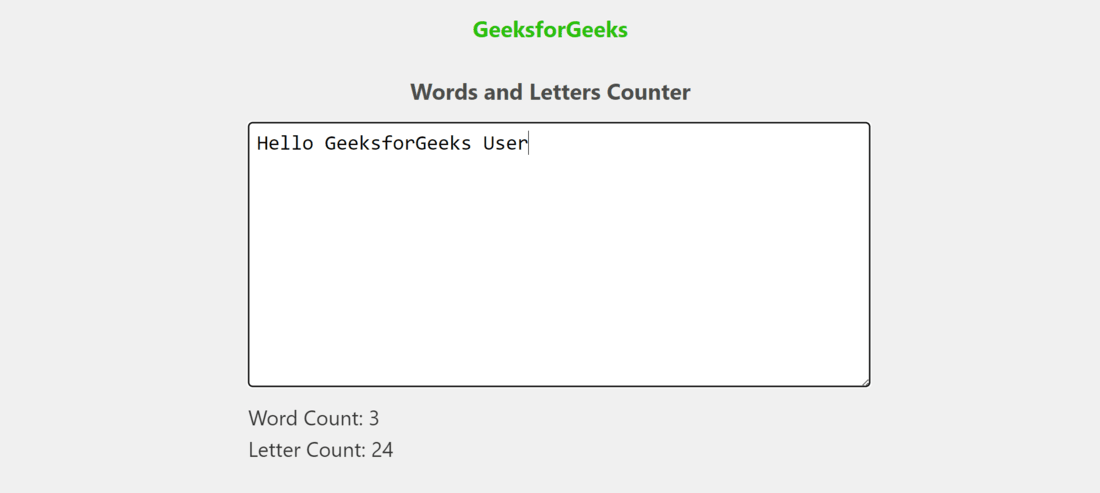
Final Output
Prerequisites and Technologies:
Approach:
The Words and Letters Counter project utilizes the capabilities of React JS to create an interactive web application. The focus is on providing users with an easy-to-use interface where they can effortlessly enter text. As users type or paste text into the designated area, the application displays two metrics in real time: the number of words and letters. The design is clean and minimalistic, ensuring that the main functionality remains prominent. Additionally, reactive state management allows for updates without requiring refreshing.
Functionalities:
- An input field where users can type or paste text.
- Real time updates of both word count and letter count as users enter their text.
Steps to create Project
Step 1: Create a new React JS project using the following command
npx create-react-app word-letter-counter
Step 2: Change to the project directory
cd word-letter-counter
Project Structure
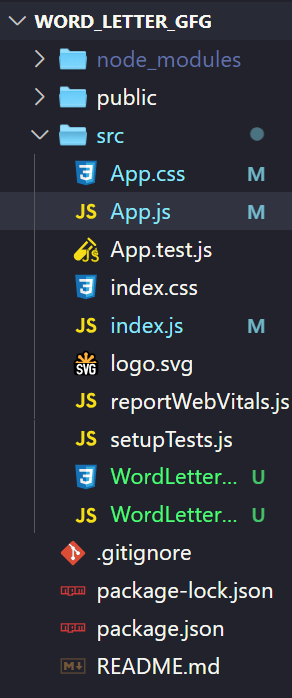
project structure
Example: Write the following code in respective files
- App.js: This serves as the entry point, for your React application. It takes care of rendering the structure of your web page. Includes the WordLetterCounter component.
- App.css: In this file you’ll find the styles that define the structure of your web page. This includes things like the page title and the container that wraps around the WordLetterCounter component.
- WordLetterCounter.js: This particular component is responsible for handling both word and letter counting functionality.
- WordLetterCounter.css: Within this file you’ll find all the styles to the WordLetterCounter component. It determines how things, like textarea input field and text displaying word and letter counts will appear on your web page.
Javascript
import React from "react" ;
import WordLetterCounter from "./WordLetterCounter" ;
import "./App.css" ;
function App() {
return (
<div className= "App" >
<h1 id= "top" >
GeeksforGeeks
</h1>
<h1>
Words and Letters
Counter
</h1>
<WordLetterCounter />
</div>
);
}
export default App;
|
Javascript
import React, { useState } from "react" ;
import "./WordLetterCounter.css" ;
function WordLetterCounter() {
const [text, setText] =
useState( "" );
const wordCount = text
.split(/\s+/)
.filter(Boolean).length;
const letterCount = text.length;
const handleTextChange = (e) => {
setText(e.target.value);
};
return (
<div>
<textarea
placeholder=
"Type your text here..."
onChange={
handleTextChange
}
value={text}
rows={5}
cols={50}
/>
<p>
Word Count:
{wordCount}
</p>
<p>
Letter Count:{ " " }
{letterCount}
</p>
</div>
);
}
export default WordLetterCounter;
|
CSS
.App {
display : flex;
flex- direction : column;
align-items: center ;
background-color : #f0f0f0 ;
height : 100 vh;
}
# top {
font-size : 27px ;
margin : 20px 0 ;
color : #2bc00d ;
}
h 1 {
font-size : 27px ;
margin : 20px 0 ;
color : #4c4e4b ;
}
|
CSS
.container {
display : flex;
flex- direction : column;
align-items: center ;
text-align : center ;
font-family : Arial , sans-serif ;
margin : 20px ;
}
textarea {
width : 100% ;
padding : 10px ;
height : 300px ;
margin-bottom : 10px ;
border : 1px solid #ccc ;
border-radius: 5px ;
font-size : 25px ;
}
p {
font-size : 25px ;
margin : 5px 0 ;
color : #333 ;
}
|
Steps to run the project
1. Type the following command in terminal.
npm start
2. Open web-browser and type the following URL
http://localhost:3000/
Output(GIF):
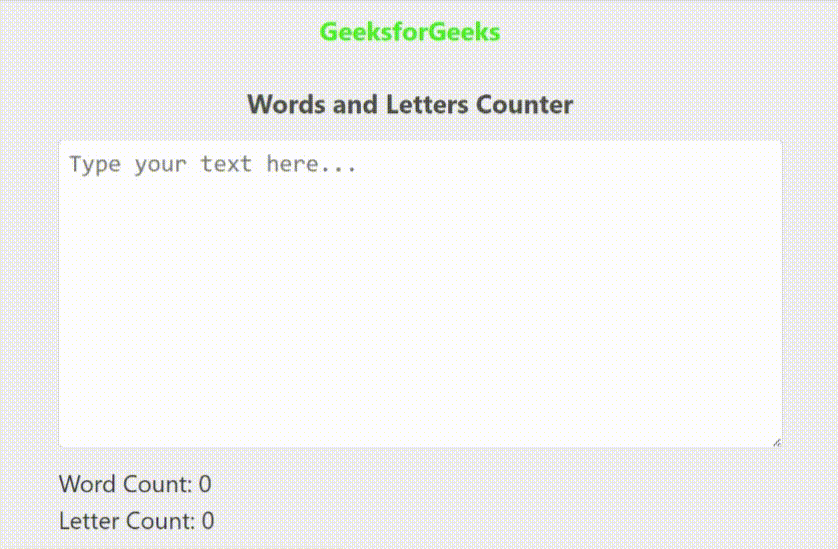
Output(GIF)
Share your thoughts in the comments
Please Login to comment...