BMI Calculator Using React
Last Updated :
12 Jul, 2023
In this article, we will create a BMI Calculator application using the ReactJS framework. A BMI calculator finds the relation between height and weight of a person. It gives a numerical value based on which the individual is categorised into underweight, normal weight, overweight and obese.
The formula to calculate BMI is:
BMI = Weight(kg)/(Height(m))^2
The category of BMI is as follows:
- Underweight: BMI < 18.5
- Normal Weight: BMI between 18.5 and 25
- Overweight: BMI between 25 and 29.9
- Obese: BMI > 30
Let’s have a look at what our final project will look like:
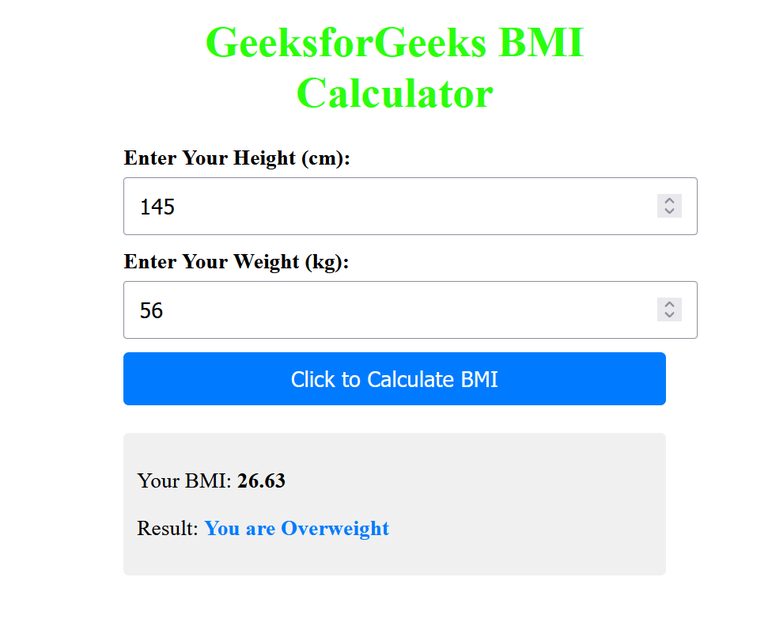
Technologies Used/Pre-requisites:
Approach:
This application is basically developed through functional components and also manages the state accordingly. The application takes input from the end user like height in cms, weight in kgs and calculates the BMI score, and also gives the message to the user whether he/she is underweight, normal, or obese. The logic of this calculation and giving the message is implemented using JSX. It takes height and weight as the input and calculates the BMI score and gives the message about the body language. We have done all the code in the App.js file, which can be easily understandable. A simple approach is been applied to develop this application.
Steps to create the application:
Step 1: Set up React project using the below command in VSCode IDE.
npx create-react-app <<name of project>>
Step 2: Navigate to the newly created project folder by executing the below command.
cd <<Name_of_project>>
Step 3: Insert the below code in the App.js and styles/App.css files mentioned in the above directory structure.
The dependencies in package.json will look like this:
{
"name": "bmi_cal",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.16.5",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Project Structure:
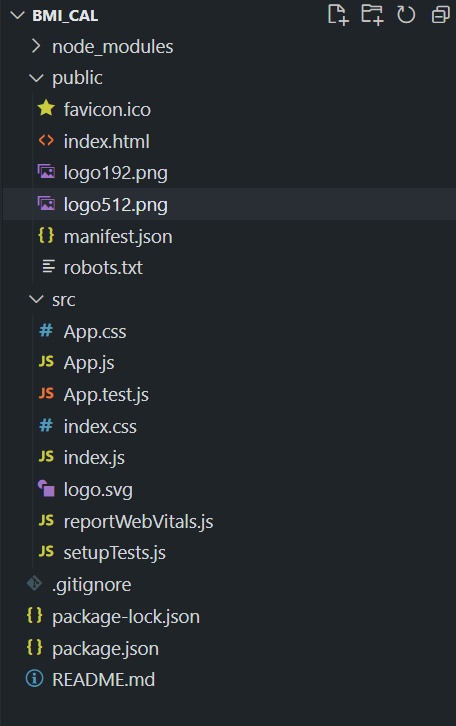
Example: Write following code in respective files(The name of the files is mentioned in the first line of each code block)
- index.html: This is an automatically created file in the public folder we just have to import the icon pack in its <head> tag.
- App.js: App.js file code is the main logic of the BMI Calculator application, where the hook (useState) is used to manage the state of the height, weight, BMI value, and BMI text. After clicking on the “Click to Calculate BMI” button the BMI value based on user input is been calculated, and depending on this BMI value, a message is also provided to the user as feedback.
- App.css: This is the styling file, which makes the application more attractive, we have provided some styling attributes like coloring the text, buttons, etc. Overall, all the styling is been managed through this file.
Javascript
import React, { useState } from 'react' ;
import './App.css' ;
function BmiCalculator() {
const [heightValue, setHeightValue] = useState( '' );
const [weightValue, setWeightValue] = useState( '' );
const [bmiValue, setBmiValue] = useState( '' );
const [bmiMessage, setBmiMessage] = useState( '' );
const calculateBmi = () => {
if (heightValue && weightValue) {
const heightInMeters = heightValue / 100;
const bmi = (weightValue / (heightInMeters * heightInMeters)).toFixed(2);
setBmiValue(bmi);
let message = '' ;
if (bmi < 18.5) {
message = 'You are Underweight' ;
} else if (bmi >= 18.5 && bmi < 25) {
message = 'You are Normal weight' ;
} else if (bmi >= 25 && bmi < 30) {
message = 'You are Overweight' ;
} else {
message = 'You are Obese' ;
}
setBmiMessage(message);
} else {
setBmiValue( '' );
setBmiMessage( '' );
}
};
return (
<div className= "container" >
<h1>GeeksforGeeks BMI Calculator</h1>
<div className= "input-container" >
<label htmlFor= "height" >Enter Your Height (cm):</label>
<input
type= "number"
id= "height"
value={heightValue}
onChange={(e) => setHeightValue(e.target.value)}
/>
</div>
<div className= "input-container" >
<label htmlFor= "weight" >Enter Your Weight (kg):</label>
<input
type= "number"
id= "weight"
value={weightValue}
onChange={(e) => setWeightValue(e.target.value)}
/>
</div>
<button className= "calculate-btn" onClick={calculateBmi}>
Click to Calculate BMI
</button>
{bmiValue && bmiMessage && (
<div className= "result" >
<p>
Your BMI: <span className= "bmi-value" >{bmiValue}</span>
</p>
<p>
Result: <span className= "bmi-message" >{bmiMessage}</span>
</p>
</div>
)}
</div>
);
}
export default BmiCalculator;
|
CSS
.container {
max-width : 400px ;
margin : 0 auto ;
padding : 20px ;
}
h 1 {
color : #1eff00 f 3 ;
text-align : center ;
margin-bottom : 20px ;
}
.input-container {
margin-bottom : 10px ;
}
label {
display : block ;
font-weight : bold ;
margin-bottom : 5px ;
}
input[type= 'number' ] {
width : 100% ;
padding : 10px ;
font-size : 16px ;
}
.calculate-btn {
display : block ;
width : 100% ;
padding : 10px ;
background-color : #007bff ;
color : #fff ;
font-size : 16px ;
border : none ;
border-radius: 4px ;
cursor : pointer ;
}
.result {
margin-top : 20px ;
padding : 10px ;
background-color : #f0f0f0 ;
border-radius: 4px ;
}
.bmi-value {
font-weight : bold ;
}
.bmi-message {
color : #007bff ;
font-weight : bold ;
}
|
Steps to run the application:
1. Type the following command in the terminal from your VS Code IDE.
npm start
2. Open the web browser and type the following URL in the address bar, to see the live application.
http://localhost:3000/
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...