How to check element exists or not in jQuery ?
Last Updated :
14 Dec, 2023
In this article, we are going to learn how to check element exists or not in jQuery, Using jQuery, we can determine whether an HTML element, such as <p>, <div>, etc., is present in the HTML page or not. There are two ways to check whether an element in the HTML document exists or not using jQuery.
Using the length property
Using the length property in jQuery. If the element exists, then the length property will return the total count of the matched elements with the specified selector. Otherwise, it will return 0 as a result which will be considered as a false value, thus the element is not present in the document.
Syntax:
$(element_selector).length;
Example: The below example will explain the use of the length property to check whether the element is present in the document or not.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< title >Check whether an element exists or not using jQuery</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
font-size: 2.5rem;
}
div {
font-weight: bold;
font-size: 1.5rem;
}
button {
cursor: pointer;
margin-top: 2rem;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< div id = "div1" >This is div with id div1</ div >
< button id = "div-1-check-btn" >
Click to check if div 1 exists
</ button >
< button id = "div-2-check-btn" >
Click to check if div 2 exists
</ button >
< script type = "text/javascript" >
$(document).ready(function () {
$("#div-1-check-btn").click(function () {
if ($("#div1").length) {
alert("Div 1 exists");
} else {
alert("Div 1 does not exist");
}
});
$("#div-2-check-btn").click(function () {
if ($("#div2").length) {
alert("Div 2 exists");
} else {
alert("Div 2 does not exist");
}
});
});
</ script >
</ body >
</ html >
|
Output:
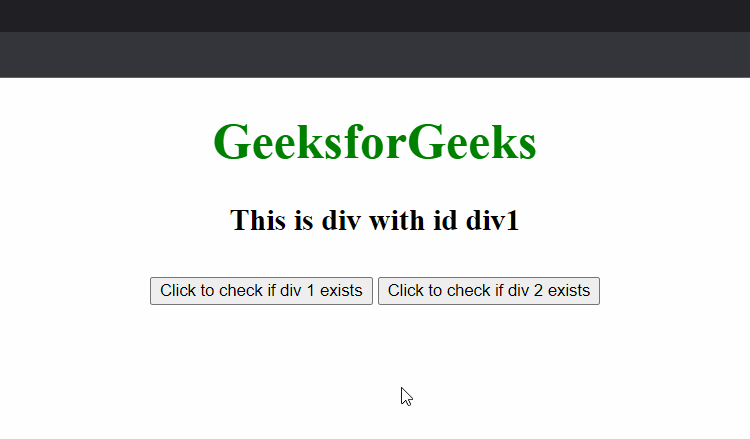
Using the jQuery plugin with methods
This approach is quite similar to the first approach due to the fact that it also uses the length property in jQuery but the difference is that a user-defined method exists() is created from the plugin using the same logic of truthy and falsy values of length as discussed in the previous technique.
Syntax:
jQuery.fn.function_name = function(){};
Example: The below example will explain the use of the jQuery plugin to check the existence of an element.
HTML
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
< title >Check whether an element exists or not using jQuery</ title >
< style >
body {
text-align: center;
}
h1 {
color: green;
font-size: 2.5rem;
}
div {
font-weight: bold;
font-size: 1.5rem;
}
button {
cursor: pointer;
margin-top: 2rem;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< div id = "div2" >This is div with id div2</ div >
< button id = "div-1-check-btn" >
Click to check if div 1 exists
</ button >
< button id = "div-2-check-btn" >
Click to check if div 2 exists
</ button >
< script type = "text/javascript" >
$(document).ready(function () {
jQuery.fn.exists = function () {
return this.length > 0;
};
$("#div-1-check-btn").click(function () {
if ($("#div1").exists()) {
alert("Div 1 exists");
} else {
alert("Div 1 does not exist");
}
});
$("#div-2-check-btn").click(function () {
if ($("#div2").exists()) {
alert("Div 2 exists");
} else {
alert("Div 2 does not exist");
}
});
});
</ script >
</ body >
</ html >
|
Output:
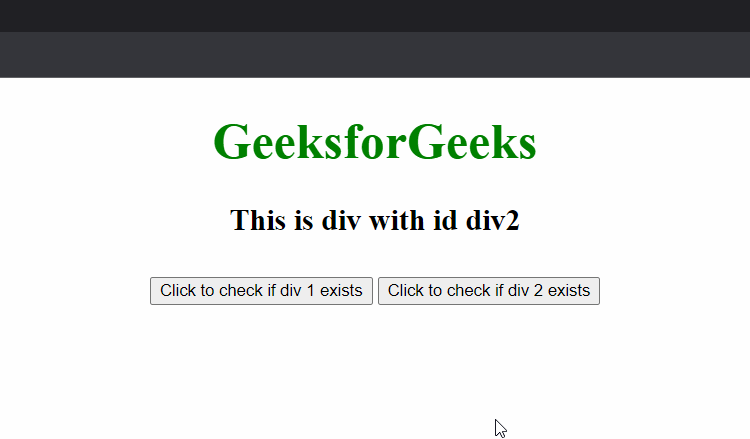
Share your thoughts in the comments
Please Login to comment...