How to Change Brightness using HTML Range Slider ?
Last Updated :
18 Mar, 2024
HTML Range Slider is the value adjusting component that can also be used to dynamically adjust and change the brightness of various elements like Image. we will explore two different approaches to Changing brightness using an HTML range slider.
These are the following approaches:
Using CSS filter Property
In this approach, we are using the CSS filter property with the brightness function to dynamically adjust the brightness of an image based on the input value of a range slider. The JavaScript code listens for input changes on the slider and updates the filter property to control the image brightness.
Syntax:
img.style.filter = `brightness(${bVal}%)`;
Example: The below example uses the CSS filter Property to Change brightness using an HTML range slider.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Example 1</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
.bContainer {
width: 80%;
text-align: center;
}
.imgContainer {
margin-bottom: 50px;
width: 50%;
margin: 0 auto;
}
img {
max-width: 100%;
height: auto;
filter: brightness(100%);
margin-bottom: 60px;
}
input[type="range"] {
width: 50%;
margin: 0 auto;
}
</style>
</head>
<body>
<div class="bContainer">
<h1>Using CSS filter Property</h1>
<div class="imgContainer">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240315180241/Kotlin-Libraries.webp"
alt="Example Image"
id="image">
</div>
<input type="range" min="0"
max="200" value="100"
class="slider"
id="brightnessSlider">
</div>
<script>
const bSlider =
document.getElementById('brightnessSlider');
const img = document.getElementById('image');
bSlider.addEventListener('input', function () {
const bVal = this.value;
img.style.filter = `brightness(${bVal}%)`;
});
</script>
</body>
</html>
Output:
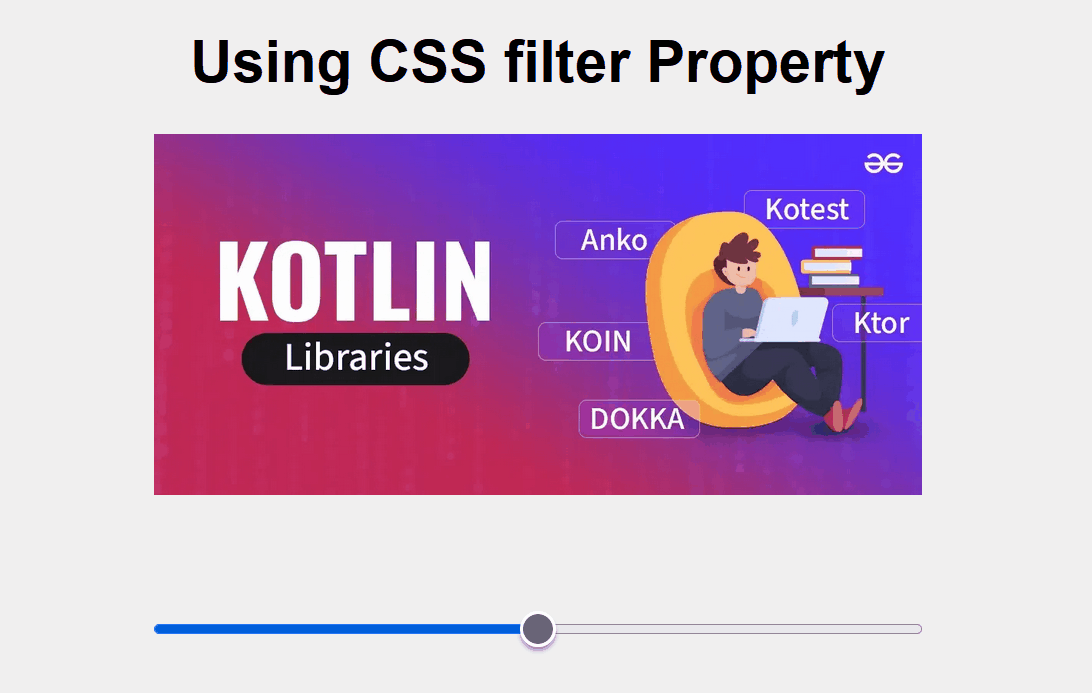
Using SVG Filter Effects
In this approach, we are using SVG Filter Effects with the <feColorMatrix> element to apply a brightness filter to an image. The <input type=”range”> is used to dynamically adjust the brightness level by updating the filter’s matrix values based on the slider input.
Syntax:
<svg width="100%" height="100%">
<filter>
</svg>
Example: The below example uses the SVG Filter Effects to Change brightness using an HTML range slider.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width
,initial-scale=1.0">
<title>Example 2</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
.bContainer {
width: 80%;
text-align: center;
}
.imgContainer {
margin-bottom: 20px;
}
img {
max-width: 100%;
height: auto;
}
input[type="range"] {
width: 50%;
margin: 0 auto;
}
h1 {
margin-bottom: 20px;
}
</style>
</head>
<body>
<div class="bContainer">
<h1>Using SVG Filter Effects</h1>
<div class="imgContainer">
<svg width="100%" height="100%">
<defs>
<filter id="brightnessFilter">
<feColorMatrix type="matrix"
values="1 0 0 0 0
0 1 0 0 0
0 0 1 0 0
0 0 0 1 0" />
</filter>
</defs>
<image xlink:href="
https://media.geeksforgeeks.org/wp-content/uploads/20240315172801/7-Best-AI-SEO-Writing-Tools-in-2024.webp"
width="100%" height="100%"
filter="url(#brightnessFilter)" />
</svg>
</div>
<input type="range" min="0" max="200"
value="100" class="slider"
id="brightnessSlider">
</div>
<script>
const bSlider = document.getElementById('brightnessSlider');
const filter = document.getElementById('brightnessFilter');
bSlider.addEventListener('input',
function () {
const bVal = this.value / 100;
filter.children[0].setAttribute('values',
`${bVal} 0 0 0 0
0 ${bVal} 0 0 0
0 0 ${bVal} 0 0
0 0 0 1 0`);
});
</script>
</body>
</html>
Output:
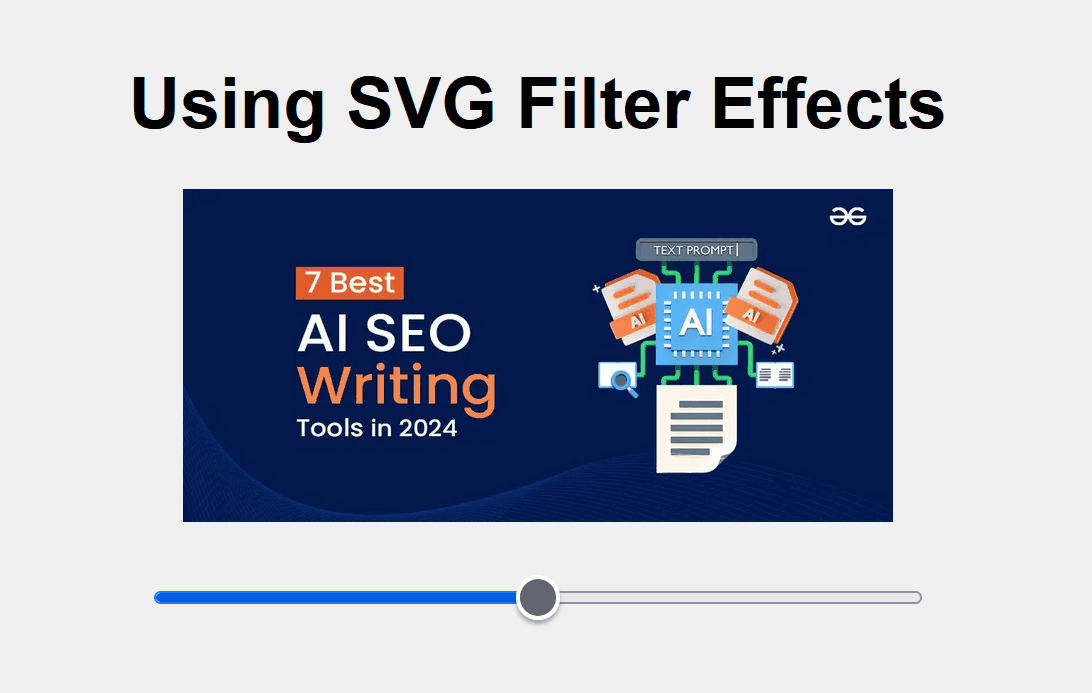
Share your thoughts in the comments
Please Login to comment...