How to Change Background Color using HTML Rounded Slider ?
Last Updated :
01 Apr, 2024
To Change the Background Color using an HTML rounded slider that dynamically changes the webpage’s background as the slider thumb is moved along its track, giving a visual indication of the selected color. The approach provides a convenient method for customizing the visual appearance of the webpage in real-time.
Approach
- The HTML document defines a slider that changes the webpage’s background color when the slider thumb is moved.
- The slider input element is defined with the ID “myRange”. It has a minimum value of 0, a maximum value of 100, and an initial value of 50. After getting the input, the function “changeBackgroundColor()” is called.
- The JavaScript function retrieves the value of a range slider with the id “myRange”.
- It then constructs an HSL color string based on the slider’s value, with full saturation and 50% lightness.
- Finally, it sets the background color of the entire web page’s body to the generated color string. This function dynamically changes the webpage’s background color as the user adjusts the slider.
Example: Implementation of changing background color using HTML rounded slider.
HTML
<!DOCTYPE html>
<html>
<head>
<meta name="viewport"
content="width=device-width,
initial-scale=1">
<title>Custom Rounded Range Slider</title>
<style>
body {
background-color: yellow;
}
h1 {
text-align: center;
}
.slidecontainer {
width: 100%;
}
.custom-slider-range:hover {
opacity: 1.2;
}
.custom-slider-range {
-webkit-appearance: none;
appearance: none;
width: 100%;
height: 25px;
background: linear-gradient(to right,
red, yellow, green);
outline: none;
opacity: 0.8;
transition: opacity 0.4s;
border-radius: 15px;
}
.custom-slider-range::-moz-range-thumb {
width: 25px;
height: 25px;
background: #fff;
border: 2px solid #000;
border-radius: 50%;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Change Background Color
using HTML Rounded Slider</h1>
<div class="slidecontainer">
<input type="range" min="1"
max="100"
value="50"
class="custom-slider-range"
id="myRange"
oninput="changeBackgroundColor()">
</div>
<script>
function changeBackgroundColor() {
let slider = document.getElementById("myRange");
let value = slider.value;
let color = 'hsl(' + value + ', 100%, 50%)';
document.body.style.background = color;
}
</script>
</body>
</html>
Output:
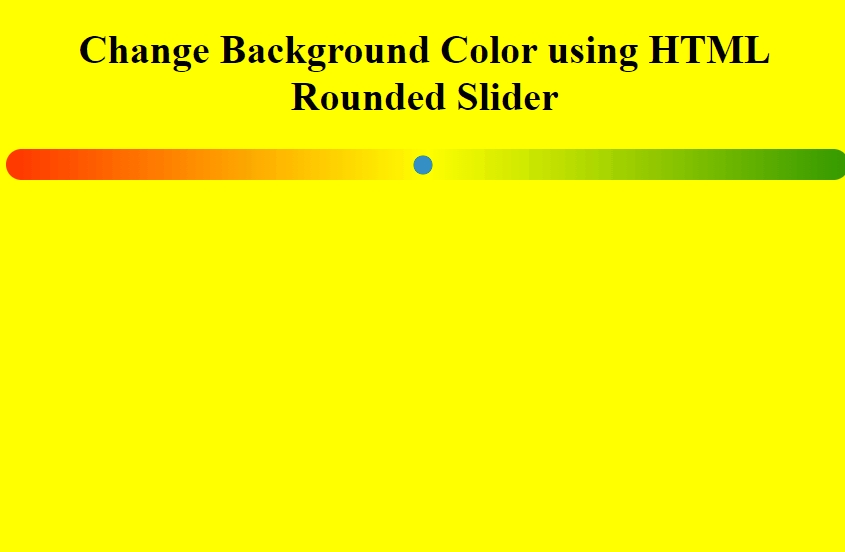
Output
Share your thoughts in the comments
Please Login to comment...