How to call a function on click event in Angular2 ?
Last Updated :
15 Nov, 2023
A Function is a set of statements that takes input, does some specific computation, and produces output. An on click event occurs when the user clicks on an element. In this article, we will see How to call a function on click event in Angular2, along with understanding the basic implementation through the examples.
Setting the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
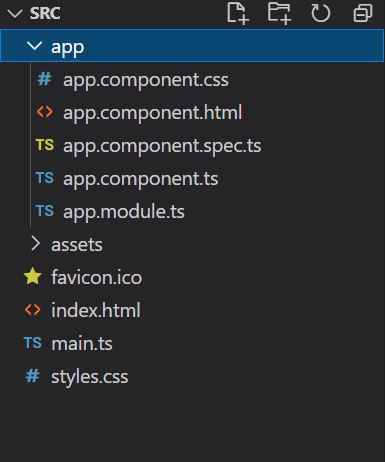
Example 1: In this example, we will call an API, and then iterate over the result, and display the data from the API in a card
HTML
< head >
< link href =
rel = "stylesheet" >
</ head >
< div style = "width: 70%;" >
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to call a function on
click event in Angular2 ?
</ h2 >
< button class = "btn btn-primary"
(click)="display()">
Call API
</ button >
< div class = "card" >
< div class = "card-header" >
< b class = "text-center" >
Data Obtained from API below
</ b >
</ div >
< div class = "card-body" >
< div * ngFor = "let item of gfg|keyvalue" >
{{item.value}}
</ div >
</ div >
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
import { HttpClient }
from '@angular/common/http' ;
import { TmplAstHoverDeferredTrigger }
from '@angular/compiler' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any
constructor(private http: HttpClient) { }
display() {
(result) => {
this .gfg = result
}
)
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
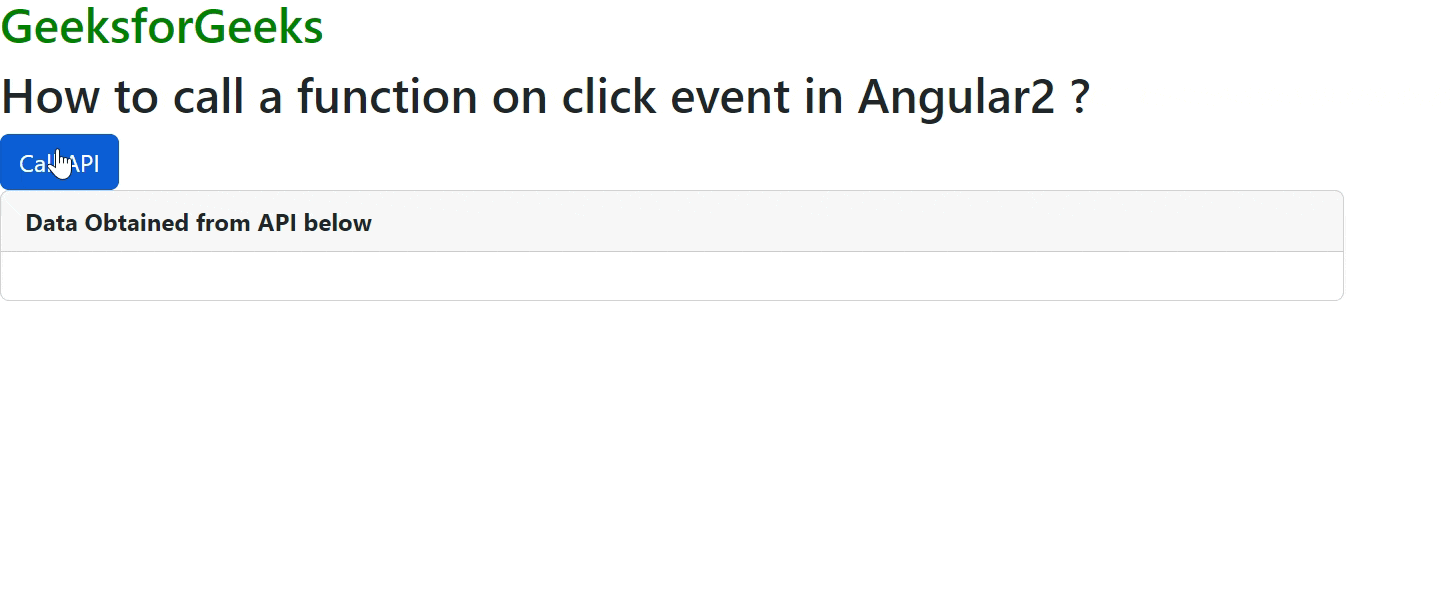
Example 2: In this example, we will fire an alert on click.
HTML
< head >
< link href =
rel = "stylesheet" >
</ head >
< div style = "width: 70%;" >
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to call a function on
click event in Angular2 ?
</ h2 >
< button class = "btn btn-primary"
(click)="fireAlert()">
Fire Alert
</ button >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { KeyValue }
from '@angular/common' ;
import { Pipe, PipeTransform }
from '@angular/core' ;
import { HttpClient }
from '@angular/common/http' ;
import { TmplAstHoverDeferredTrigger }
from '@angular/compiler' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any
constructor(private http: HttpClient) { }
fireAlert() {
alert( "Hi Geek! Please vote for this article for GPL 2023" )
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
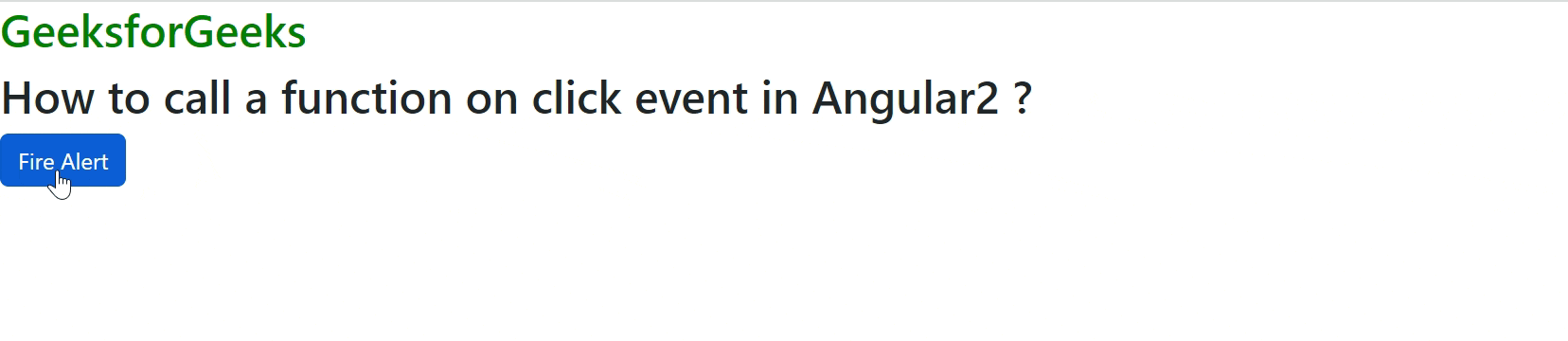
Share your thoughts in the comments
Please Login to comment...