How to Scroll to an Element on click in Angular ?
Last Updated :
06 Dec, 2023
In this article, we will see how to scroll to an element on click in Angular. Here, we will create a component that enables scrolling to specific targets when a button is pressed within the document from one target to another.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
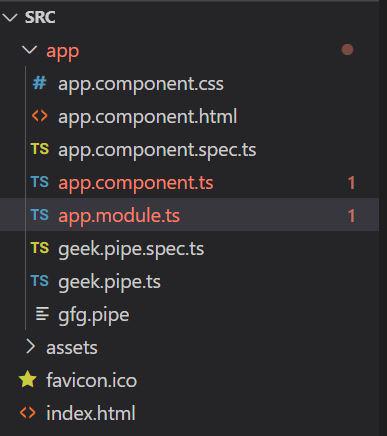
Example 1: In this example, we have multiple cards, we can scroll from one card to another with a button click. We have defined ‘name’ and ‘target’ for each card, and hence passing the same in a function scroll. In the .ts file, we have implemented the scroll function and utilized scrollIntoView.
HTML
< link href =
rel = "stylesheet" >
< div class = "jumbotron"
#first name = "first" >
< h2 class = "display-4 text-success" >
GeeksforGeeks
</ h2 >
< h2 class = "display-4" >
Scroll to element on click in Angular
</ h2 >
< hr class = "my-4" >
< p >
Click on the below button
to starting learning.
</ p >
< button (click)="scroll(target)"
class = "btn btn-primary" >
Scroll To last
</ button >
</ div >
< div class = "card"
#HTML name = "HTML" >
< div class = "card-header" >
HTML
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Front End Technologies
</ h5 >
< p class = "card-text" >
HTML, CSS, Javascript,
Angular, React.js
</ p >
< button (click)="scroll(java)"
class = "btn btn-primary" >
Scroll To Java
</ button >
</ div >
</ div >
< br >
< div class = "card"
#java name = "java" >
< div class = "card-header" >
Java
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Back End Technologies
</ h5 >
< p class = "card-text" >
Springboot, APIS
</ p >
< button (click)="scroll(HTML)"
class = "btn btn-primary" >
Scroll To HTML
</ button >
</ div >
</ div >
< br >
< div class = "card"
#target name = "last" >
< div class = "card-header" >
Last
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Backend Technologies
</ h5 >
< p class = "card-text" >
Node.js, Django,Express
</ p >
< button (click)="scroll(first)"
class = "btn btn-primary" >
Scroll To first
</ button >
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { Router, NavigationEnd }
from '@angular/router' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent implements OnInit {
constructor(private router: Router) { }
ngOnInit() {
this .router.events.subscribe((event) => {
if (!(event instanceof NavigationEnd)) {
return ;
}
window.scrollTo(0, 0)
});
}
scroll(el: HTMLElement) {
el.scrollIntoView();
console.log( "Scrolling to " + el.getAttribute( "name" ))
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { RouterModule, Routes }
from '@angular/router' ;
import { AppComponent }
from './app.component' ;
const routes: Routes = [
{ path: '' , component: AppComponent },
];
@NgModule({
imports: [BrowserModule,
RouterModule.forRoot(routes)],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
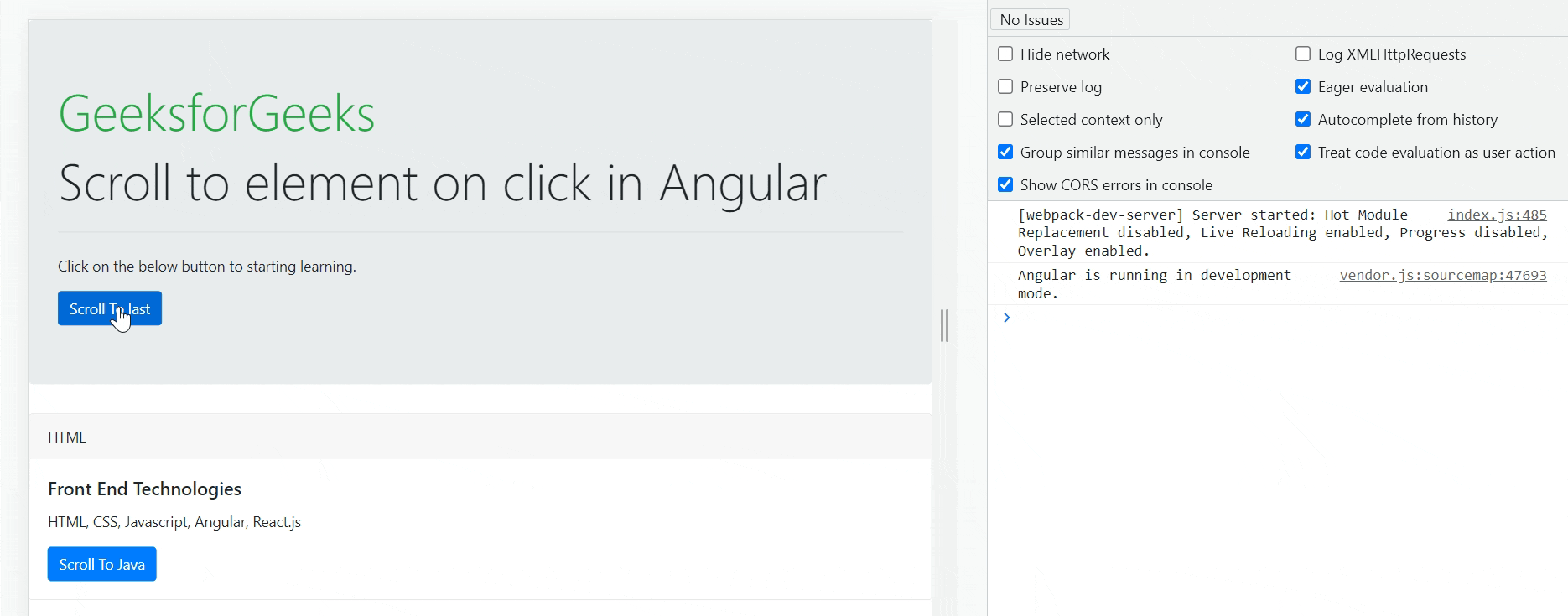
Example 2: In this example, we can add a smooth scrolling parameter, by adding {behavior:”smooth”} to scrollIntoView, which will smoothly help the scrolling.
HTML
< link href =
rel = "stylesheet" >
< div class = "jumbotron"
#first id = "first" >
< h2 class = "display-4 text-success" >
GeeksforGeeks
</ h2 >
< h2 class = "display-4" >
Scroll to element on click in Angular
</ h2 >
< hr class = "my-4" >
< p >
Click on the below button
to starting learning.
</ p >
< button (click)="scroll(target)"
class = "btn btn-primary" >
Scroll To last
</ button >
</ div >
< div class = "card"
#HTML id = "HTML" >
< div class = "card-header" >
HTML
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Front End Technologies
</ h5 >
< p class = "card-text" >
HTML, CSS, Javascript,
Angular, React.js
</ p >
< button (click)="scroll(java)"
class = "btn btn-primary" >
Scroll To Java
</ button >
</ div >
</ div >
< br >
< div class = "card"
#java id = "java" >
< div class = "card-header" >
Java
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Back End Technologies
</ h5 >
< p class = "card-text" >
Springboot, APIS
</ p >
< button (click)="scroll(HTML)"
class = "btn btn-primary" >
Scroll To HTML
</ button >
</ div >
</ div >
< br >
< div class = "card"
#target id = "last" >
< div class = "card-header" >
Last
</ div >
< div class = "card-body" >
< h5 class = "card-title" >
Backend Technologies
</ h5 >
< p class = "card-text" >
Node.js, Django,Express
</ p >
< button (click)="scroll(first)"
class = "btn btn-primary" >
Scroll To first
</ button >
</ div >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { Router, NavigationEnd } from '@angular/router' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent implements OnInit {
constructor(private router: Router) { }
ngOnInit() {
this .router.events.subscribe((event) => {
if (!(event instanceof NavigationEnd)) {
return ;
}
window.scrollTo(0, 0)
});
}
scroll(el: HTMLElement) {
el.scrollIntoView({ behavior: "smooth" });
console.log( "Scrolling to " + el.getAttribute( "id" ))
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { RouterModule, Routes }
from '@angular/router' ;
import { AppComponent } from './app.component' ;
const routes: Routes = [
{ path: '' , component: AppComponent },
];
@NgModule({
imports: [BrowserModule,
RouterModule.forRoot(routes)],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
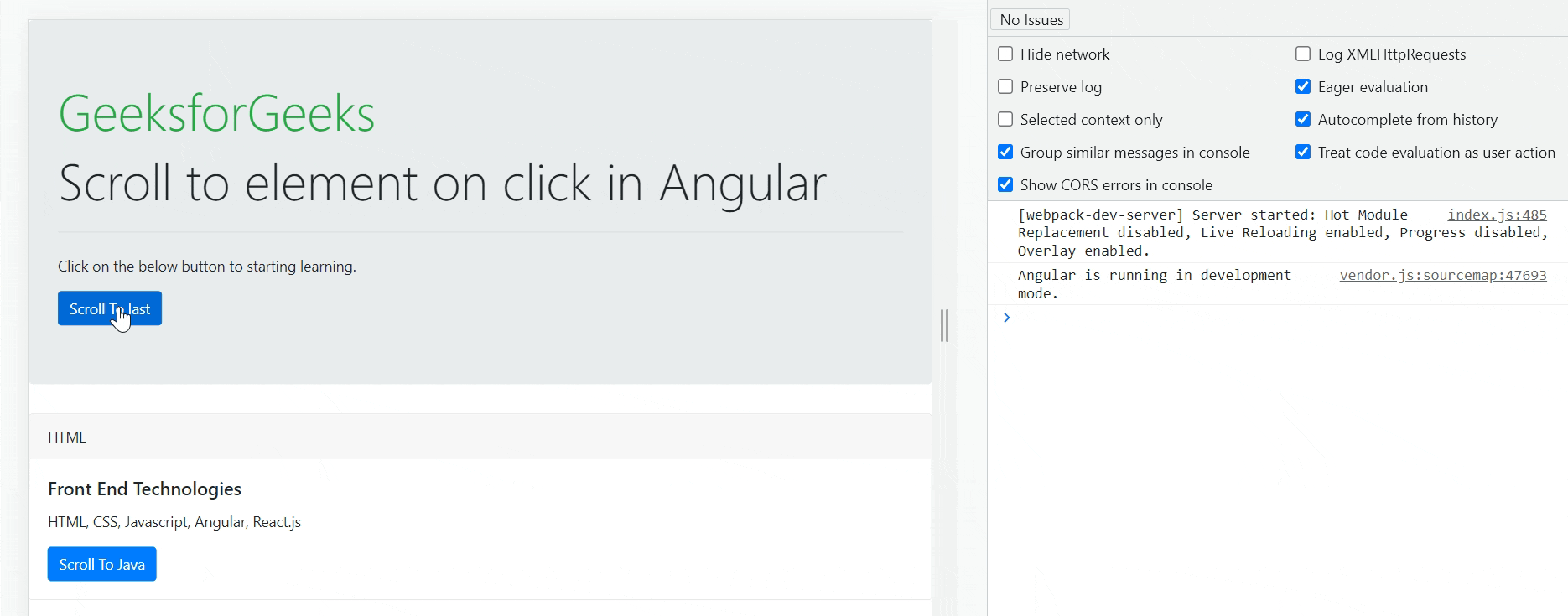
Share your thoughts in the comments
Please Login to comment...