How to Alert Users to Errors with React Bootstrap ?
Last Updated :
16 Oct, 2023
In React Bootstrap, an `Alert` is a component used to display various types of messages or notifications to users. These messages can include informational messages, warnings, errors, or any other kind of feedback that you want to communicate to the user. Alerts are commonly used to provide feedback or to convey important information in a visually prominent and user-friendly manner.
Steps to create the application
Step 1: Install React Application
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command
cd foldername
Step 3: Install the required modules using the following command.
npm install react-bootstrap bootstrap
Here are two common approaches to alert users to errors with React Bootstrap:
Approach 1: Using the Alert Component
In this approach,
- variant=”danger” sets the alert to display with a red background for error messages.
- onClose is used to define an action when the close button is clicked, typically to hide the alert.
- dismissible adds a close button to the alert.
Syntax:
<Alert variant="danger" onClose={() => setShow(false)} dismissible>
<Alert.Heading>Error</Alert.Heading>
<p>Error message goes here.</p>
</Alert>
Example: This example shows the use of the above-explained appraoch.
Javascript
import React, { useState } from "react" ;
import { Alert, Button } from "react-bootstrap" ;
import "bootstrap/dist/css/bootstrap.min.css" ;
function ErrorAlertExample() {
const [show, setShow] = useState( false );
const handleButtonClick = () => {
setShow( true );
};
return (
<div>
<Button onClick={handleButtonClick}>
Show Error
</Button>
{show && (
<Alert
variant= "danger"
onClose={() => setShow( false )}
dismissible>
<Alert.Heading>Error</Alert.Heading>
<p>Error message goes here.</p>
</Alert>
)}
</div>
);
}
function App() {
return (
<div
className= "col-12 d-flex
justify-content-center
align-items-center"
style={{ height: "100vh" }}>
<div className= "col-4 h-50" >
<ErrorAlertExample />
</div>
</div>
);
}
export default App;
|
Steps To Run Application:
npm start
Output:
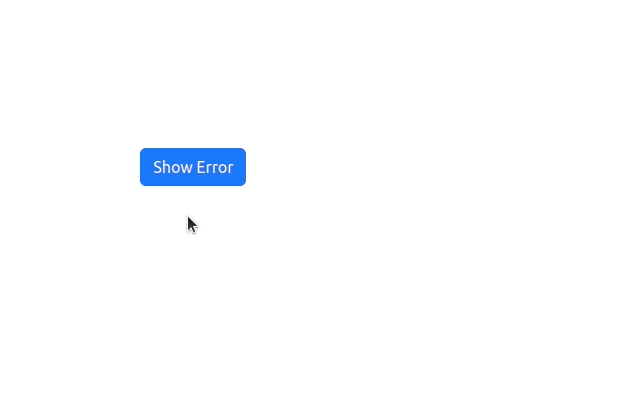
Approach 2: Using the Toast Component
In this approach,
- position defines the location where the toast notification appears.
- bg sets the background color for error messages.
- autohide specifies the duration in milliseconds before the toast automatically disappears.
Syntax
<ToastContainer position="top-end">
<Toast bg="danger" autohide={3000}>
<Toast.Header>
<strong className="me-auto">Error</strong>
</Toast.Header>
<Toast.Body>Error message goes here.</Toast.Body>
</Toast>
</ToastContainer>
Example: This example shows the use of the above-explained approach.
Javascript
import React, { useState } from "react" ;
import {
Button,
Toast,
ToastContainer,
} from "react-bootstrap" ;
import "bootstrap/dist/css/bootstrap.min.css" ;
function ErrorToastExample() {
const [showA, setShow] = useState( false );
const toggleShow = () => setShow(!showA);
return (
<div className= "relative" >
<Button onClick={toggleShow}>Show Error</Button>
<ToastContainer>
<Toast
bg= "danger"
autohide={100}
show={showA}
onClose={toggleShow}>
<Toast.Header>
<strong className= "me-auto" >
Error
</strong>
</Toast.Header>
<Toast.Body>
Error message goes here.
</Toast.Body>
</Toast>
</ToastContainer>
</div>
);
}
function App() {
return (
<div
className= "col-12 d-flex
justify-content-center
align-items-center"
style={{ height: "100vh" }}>
<div className= "col-4 h-50" >
<ErrorToastExample />
</div>
</div>
);
}
export default App;
|
Steps To Run Application:
npm start
Output:
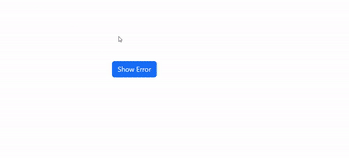
output
Share your thoughts in the comments
Please Login to comment...