How does Redux Toolkit simplify Redux development?
Last Updated :
25 Apr, 2024
Redux is a powerful state management library for JavaScript applications, but setting it up and managing boilerplate code can be cumbersome. Redux Toolkit is an official package from the Redux team designed to streamline Redux development and reduce boilerplate code. In this article, we’ll explore how Redux Toolkit simplifies Redux development and improves developer productivity.
What is Redux Toolkit?
Redux Toolkit is an opinionated set of utilities and tools for efficient Redux development. It provides a collection of pre-configured Redux packages and abstractions that abstract away much of the complexity of setting up and managing Redux applications. Redux Toolkit is built on top of Redux principles and best practices, making it an ideal choice for both beginners and experienced Redux developers.
- Boilerplate Reduction: Redux Toolkit reduces the need for boilerplate code when setting up Redux. It offers utility functions like
createSlice()
for creating reducers and action creators together, saving developers time and effort. - Immutable Updates: The Redux Toolkit promotes immutable updates to the state, ensuring predictability and simplified debugging. It utilizes the immer library internally, allowing developers to write reducer logic that appears to mutate state directly while producing a new immutable state.
- Built-in Middleware: Redux Toolkit comes with pre-configured middleware like redux-thunk for handling asynchronous actions without manual setup. This reduces configuration overhead and simplifies the development process.
- Performance Optimizations: Redux Toolkit includes performance optimizations such as “batched” state updates. This can improve performance in large applications by reducing the number of re-renders triggered by state changes, resulting in smoother user experiences.
Prerequisites:
Steps to create React app
Step 1: Create a reactJS application by using this command
npx create-react-app my-app
Step 2:Â Navigate to the project directory
cd my-app
Step 3:Â Install the necessary packages/libraries in your project using the following commands.
npm install redux react-redux @reduxjs/toolkit
Project Structure:
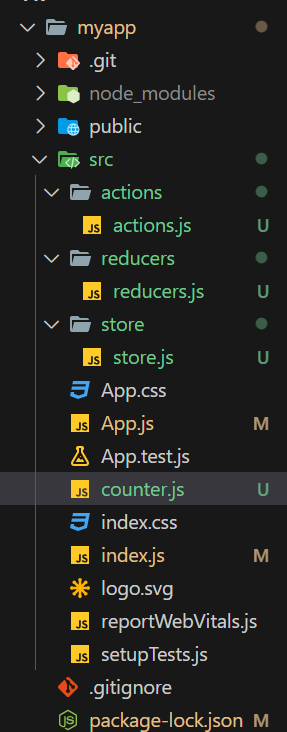
The updated dependencies in package.json file will look like:
"dependencies": {
"@reduxjs/toolkit": "^2.2.3",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.1",
"react-scripts": "5.0.1",
"redux": "^5.0.1",
"web-vitals": "^2.1.4"
}
Using Traditional Redux Approach:
Involves defining actions, action creators, and reducers separately, leading to verbose code and manual state management.
Approach:
- Involves defining action types, action creators, and reducers separately, leading to fragmented code organization.
- Requires writing repetitive code for defining actions, action creators, and reducers.
- Store setup involves creating a Redux store manually using
createStore
from Redux. - Dispatching actions requires accessing the store and dispatching actions using
store.dispatch()
.
Example: This example uses the traditional redux approach to justify the above title.
CSS
/* App.css */
button {
margin: 10px;
}
JavaScript
// src/actions/actions.js
export const increment = () => ({
type: 'INCREMENT'
});
export const decrement = () => ({
type: 'DECREMENT'
});
JavaScript
// src/reducers/reducers.js
const initialState = {
count: 0
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return {
count: state.count + 1
};
case 'DECREMENT':
return {
count: state.count - 1
};
default:
return state;
}
};
export default counterReducer;
JavaScript
// src/store/store.js
import { createStore } from 'redux';
import counterReducer from '../reducers/reducers';
const store = createStore(counterReducer);
export default store;
JavaScript
// src/app.js
import './App.css';
import Counter from './counter';
function App() {
return (
<div className="App">
<Counter/>
</div>
);
}
export default App;
JavaScript
// src/counter.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './actions/actions';
const Counter = () => {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
<div>
<h2>Counter: {count}</h2>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
};
export default Counter;
JavaScript
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store/store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step to Run Application:Â Run the application using the following command from the root directory of the project
npm start
Output:Â Your project will be shown in the URL http://localhost:3000/
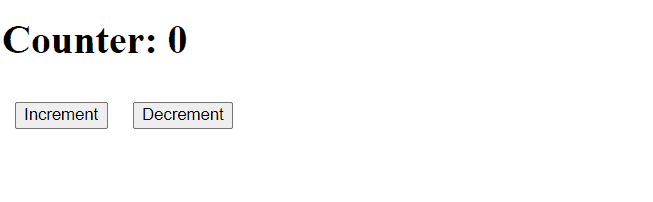
Redux Toolkit streamlines Redux development by providing utilities like “createSlice” for combined reducer and action creation, promoting immutability, simplifying async logic, and offering built-in support for dev tools and middleware.
Approach:
- Utilizes “createSlice” from Redux Toolkit to define reducers and action creators together within a single slice, reducing code fragmentation.
- Promotes immutability by internally leveraging the “immer” library, simplifying state updates without directly mutating state.
- Simplifies handling async actions with “createAsyncThunk”, streamlining asynchronous logic in Redux workflows.
- Offers built-in support for dev tools and Redux middleware, enhancing development experience with debugging capabilities and middleware integration.
Example: This example uses the Redux Toolkit approach to justify the above title.
CSS
/* App.css */
button {
margin: 10px;
}
JavaScript
// app/store.js
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from '../features/counterSlice';
export default configureStore({
reducer: {
counter: counterReducer,
},
});
JavaScript
// components/Counter.js
import React from 'react';
import { useDispatch, useSelector } from 'react-redux';
import { increment, decrement } from '../features/counterSlice';
const Counter = () => {
const count = useSelector(state => state.counter.value);
const dispatch = useDispatch();
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={
() => dispatch(increment())}>
Increment
</button>
<button onClick={
() => dispatch(decrement())}>
Decrement
</button>
</div>
);
};
export default Counter;
JavaScript
// features/counterSlice.js
import { createSlice } from '@reduxjs/toolkit';
export const counterSlice = createSlice({
name: 'counter',
initialState: {
value: 0,
},
reducers: {
increment: state => {
state.value += 1;
},
decrement: state => {
state.value -= 1;
},
},
});
export const {
increment,
decrement
} = counterSlice.actions;
export default counterSlice.reducer;
JavaScript
// App.js
import React from 'react';
import Counter from './components/Counter';
function App() {
return (
<div className="App">
<header
className="App-header">
<Counter />
</header>
</div>
);
}
export default App;
JavaScript
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './app/store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step to Run Application:Â Run the application using the following command from the root directory of the project
npm start
Output:Â Your project will be shown in the URL http://localhost:3000/
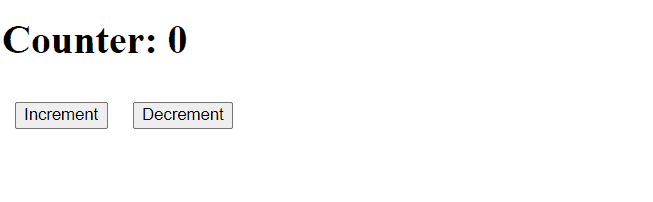
Share your thoughts in the comments
Please Login to comment...