Difference between React.memo() and PureComponent
Last Updated :
05 Apr, 2024
We know that both `React.memo()` and `PureComponent‘ are maximizing techniques in React to improve performance by intercepting unnecessary re-renders. That is the process of building fast and smooth applications. In this article, we will explore how PureComponent and React.memo work and show you how to use them in your React native applications.
React.memo():
React.memo() is a higher-order component provided by React that recollects the rendered output of a functional component. That component is similar to `shouldComponentUpdate()` in class components but for functional components. When you wrap a functional component with `React.memo()`, React will only re-render the component if its props have changed.
Syntax:
const MemoizedComponent = React.memo(FunctionalComponent);
Features:
- React.memo is a higher-order component that memoizes the rendered output of a functional component.
- That component is similar to `shouldComponentUpdate()` in class components but for functional components.
- React.memo() accepts a functional component.
- React.memo() takes an optional second argument, a comparison function.
Example: Below is an example of creating a React.memo().
JavaScript
// src/App.js
import React from 'react';
import './App.css'; // Import CSS file for styling
const MyComponent = ({ data }) => {
return <div>{data}</div>;
};
const MemoizedComponent = React.memo(MyComponent);
const ParentComponent = () => {
const [value, setValue] = React.useState(0);
return (
<div className="container">
<button onClick={() => setValue(value + 1)}>
Increment
</button>
<MemoizedComponent data={value} />
</div>
);
};
export default ParentComponent;
Ouput:
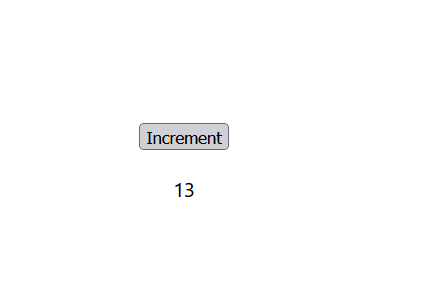
PureComponent:
PureComponent is a base-class component provided by React that implements a shallow comparison of props and states to determine if a component should re-render.that is particularly useful for class components to optimize performance.
Syntax:
class MyComponent extends React.PureComponent {
// Component logic
}
Features:
- PureComponent performs a shallow comparison of props and state to decide whether to re-render.
- It is particularly useful for class components to optimize performance.
- Stateless:Pure componets do not hold any internal state.they recieve data through props and render UI based on that data.
- Performance Optimization:Pure components render implement a shouldComponentUpdate lifecycle method with shallow props and state comparison.
- Easy Testing:with dererministic rendering and predictable behavior,pure components are easier to test.
For the PureComponet Install the required package in your application using the following command.
npm install react-motion
Example: Below is an example of creating a PureComponent.
JavaScript
import React, { PureComponent } from 'react';
class ExampleComponent extends PureComponent {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
handleClick = () => {
this.setState(prevState => ({
count: prevState.count + 1
}));
};
render() {
console.log('Render called');
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
export default ExampleComponent;
Output:
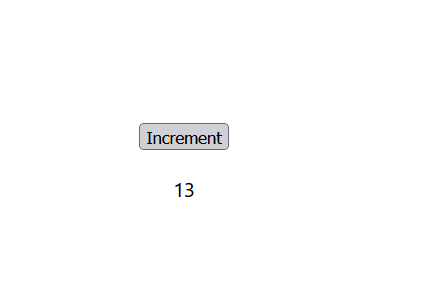
Example of React.memo():
JavaScript
// App.js
import React, {
useState
} from 'react';
import {
Motion,
spring
} from 'react-motion';
import './App.css';
const TodoItem = React.memo(({ todo, onDelete }) => {
return (
<Motion defaultStyle={{ opacity: 0, x: -50 }}
style={{ opacity: spring(1), x: spring(0) }}>
{({ opacity, x }) => (
<li style={{
opacity,
transform: `translateX(${x}px)`
}}>
<span>{todo}</span>
<button onClick={onDelete}>
Delete
</button>
</li>
)}
</Motion>
);
});
const App = () => {
const [todos, setTodos] = useState([]);
const [inputValue, setInputValue] = useState('');
const handleInputChange = (e) => {
setInputValue(e.target.value);
};
const handleAddTodo = () => {
if (inputValue.trim() !== '') {
setTodos((prevTodos) =>
[...prevTodos, inputValue]);
setInputValue('');
}
};
const handleDeleteTodo = (index) => {
setTodos((prevTodos) =>
prevTodos.filter((_, i) => i !== index));
};
return (
<div className="app">
<h1 className="title">React Motion To-Do List</h1>
<div className="input-container">
<input
type="text"
value={inputValue}
onChange={handleInputChange}
placeholder="Enter your to-do"
/>
<button onClick={handleAddTodo}>Add</button>
</div>
<ul className="todo-list">
{todos.map((todo, index) => (
<TodoItem key={index} todo={todo}
onDelete={() => handleDeleteTodo(index)} />
))}
</ul>
</div>
);
};
export default App;
Output:
.gif)
Difference Between React.memo() and PureComponent:
Feature
| React.memo()
| PureComponent
|
---|
Type
| Higher order component
| Base class component
|
---|
Performance
| Good for functional components
| Good for class components
|
---|
Re-render logic
| Memoizes based on props
| Performs shallow comparison of props and state
|
---|
Usage
| Functional Components
| Class components
|
---|
Conclusion:
Using React.memo() and PureComponent are both powerful tools provided by React to optimize performance by preventing unnecessary re-renders. Understanding their differences and choosing the suitable one based on the component’s type and basic requirements can significantly improve the efficiency of React applications.
Share your thoughts in the comments
Please Login to comment...