Globals View in Express JS
Last Updated :
04 Apr, 2024
Express.js is a popular web framework for Node.js that simplifies the process of building web applications and APIs. One of the features provided by Express.js is the ability to define and use global variables within your application’s views.
In this article, we’ll explore what globals views are, how to set them up in Express.js, and best practices for using them effectively.
What is a Global View?
Global views in Express.js refer to the practice of making certain variables accessible across all views within an Express application. These variables can hold data that needs to be shared globally, such as user information, configuration settings, or constant values. By using global views, you can avoid repetitive data passing and enhance the overall organization of your application.
Common Globals in Express.js:
- req: Holds incoming request data like URLs, parameters, and headers.
- res: Sends back the HTTP response to the client.
- app: Represents the Express application, used for setup and configuration.
- locals: Stores variables specific to each request.
- __dirname: Gives the directory name of the current module.
- __filename: Gives the filename of the current module.
Setting Up Global Views
To set up global views in Express.js, you can utilize middleware functions provided by the framework. Middleware functions have access to the request (req
) and response (res
) objects, allowing you to modify data or behavior before sending a response to the client. Here’s an example of how you can set up global views:
// Import required modules
const express = require('express');
const app = express();
// Define global variables middleware
app.use((req, res, next) => {
res.locals.currentUser = req.user; // Example: storing user information
res.locals.appName = 'MyApp'; // Example: application name
next();
});
// Route handling
app.get('/', (req, res) => {
res.render('index'); // Render index view with global variables
});
// Start the server
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Explanation: In this above example, We use app.use
to create a middleware function that sets global variables using res.locals
.
res.locals.currentUser
and res.locals.appName
are examples of global variables that can be accessed in any view.
Accessing Global Variables in Views
Once global variables are set up, you can access them directly within your views. Popular templating engines like EJS, Pug, and Handlebars can render these variables seamlessly. Here’s an example using EJS:
<!-- views/index.ejs -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><%= appName %></title>
</head>
<body>
<h1>Welcome <%= currentUser.username %></h1>
<!-- Other HTML content -->
</body>
</html>
Explanation: In this EJS template, <%= appName %>
displays the application name.
<%= currentUser.username %>
accesses the username of the current user.
Best Practices for Using Global Variables
To ensure efficient and secure usage of global views, consider the following best practices:
- Limited Scope: Use global variables sparingly and only for data that truly needs to be shared across views.
- Security Measures: Avoid storing sensitive information in global variables, especially in client-side accessible views.
- Clear Naming: Choose descriptive names for global variables to improve code readability and maintenance.
- Middleware Order: Pay attention to the order of middleware functions to ensure global variables are set before rendering views.
Steps to Setup Application
Step 1: Create a express application by using this command
npm init
Step 2: Install the necessary packages/libraries in your project using the following commands.
npm install express ejs
Folder Structure:
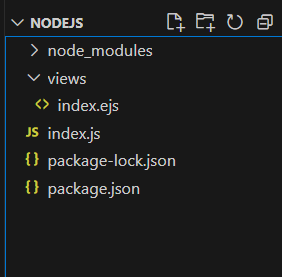
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3",
}
Example: Illustartipon to showcase the use of global views.
HTML
//index.js
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Global View Example</title>
</head>
<body>
<h1>
<%= globalVariable %>
</h1>
</body>
</html>
Javascript
const express = require('express');
const app = express();
app.set('view engine', 'ejs');
app.use((req, res, next) => {
res.locals.globalVariable = 'Hello, Global!';
next();
});
app.get('/', (req, res) => {
res.render('index');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Step to Run Application: Run the application using the following command from the root directory of the project
node .\index.js
Output: Your project will be shown in the URL http://localhost:3000/

Conclusion
Global views in Express.js provide a convenient way to manage shared data across views in your web application. By setting up global variables using middleware and following best practices, you can enhance code organization, reduce redundancy, and improve overall development efficiency. Leveraging global views responsibly can lead to more maintainable and scalable Express.js applications.
Share your thoughts in the comments
Please Login to comment...