Why use View Engines with Express ?
Last Updated :
18 Mar, 2024
Express.js is a popular and adaptable framework for developing web apps and APIs using Node.js. Its simplicity, versatility, and solid features make it a popular option among developers. The usage of view engines is an important component of Express.js that greatly helps to its efficacy.
In this article, we’ll look at why view engines are important in Express.js programming and discuss their benefits.
What are View Engines?
View engines are templates that help developers create HTML content by combining fixed HTML with dynamic data. By integrating with frameworks like Express.js, they simplify the process of showing dynamic data on web pages. View engines connect with the framework’s code and display HTML that reflects the app’s logic and data in real time.
In the context of Express.js, popular view engines Include:
- Syntax: Uses double curly braces {{}} for placeholders.
- Features: Supports variables, conditionals, loops, and partials.
- Simplicity: Provides an easy-to-understand syntax for dynamic templating.
- JavaScript Integration: Allows embedding JavaScript within HTML markup.
- Syntax: Uses <% %> for JavaScript code blocks and <%= %> for output.
- Flexibility: Offers familiarity to JavaScript developers and supports dynamic content rendering.
- Concise Syntax: Uses indentation instead of brackets for HTML structure.
- Features: Supports mixins, includes, and filters for modular templates.
- Expressiveness: Provides a powerful yet succinct syntax for template creation.
- Logic-less: Focuses on simplicity and portability without programming logic.
- Syntax: Uses double curly braces {{}} for placeholders and tags.
- Versatility: Compatible with multiple programming languages and environments for templating.
Why Use View Engines with Express.js?
1. Separation of Concerns:
View engines help keep different parts of an application separate, making it easier to manage and scale. Developers can focus on specific tasks (like creating templates) without worrying about other aspects of the application (like business logic). These templates can be used repeatedly throughout the application, ensuring a consistent look and feel while reducing the amount of code needed.
2. Dynamic Content Rendering:
Express.js apps often handle dynamic content from user actions, database results, or external APIs. View engines help with this by letting you put variables, conditions, loops, and other logic right in the HTML templates. This makes it easier to create dynamic web pages while keeping your code organized and readable.
3. Template Inheritance and Layouts:
Express.js supports various view engines that provide functionalities like template inheritance and layouts. Template inheritance involves creating a primary template with shared elements such as headers, footers, and navigation. Other templates can inherit this base template and modify specific sections when necessary. This technique fosters code reuse and modularity, simplifying the management of large web applications.
4. Flexibility and Customization:
Express.js offers numerous view engines, each with distinct styles and capabilities. Developers can select the appropriate engine based on project requirements, coding preferences, and team experience. Handlebars provides simplicity, EJS resembles plain JavaScript for familiarity, while Pug boasts a compact syntax. Express.js allows the use of multiple view engines simultaneously. Furthermore, developers can customize or extend view engines to align with unique project demands, maximizing the framework’s adaptability.
5. Improved Developer Productivity:
View engines enhance developer efficiency by optimizing dynamic content rendering and promoting code reusability. They offer template inheritance and layout management tools, freeing developers from repetitive HTML tasks and presentation concerns. This empowers them to concentrate on developing core features and implementing business logic. As a result, view engines lead to quicker development cycles, reduced product launch time, and improved project efficiency.
Setting up Template Engines in Express.js
Step 1: Create a new directory for your project:
mkdir express-view
cd express-view
Step 2: Initialize a new Node.js project:
npm init -y
Step 3: Installation: Install the desired template engine package using npm or yarn. For example, to use EJS, in express you can run:
npm install express
npm install ejs
Step 4: Configuration: Configure Express.js to use the View engine by setting the `view engine` and `views` directory.
For example:
app.set(‘view engine’, ‘ejs’);
app.set(‘views’, path.join(__dirname, ‘views’));
Step 5: Rendering: Use the `res.render()` method to render templates in middleware functions or route handlers. To add dynamic content to the template, pass data as an object.
For example:
app.get(‘/’, (req, res) => {
const data = { title: ‘Express.js View Engine’, message: ‘Welcome to Geeks For Geeks!’ };
res.render(‘index’, data);
});
Step 6: Creating Templates: In the designated views directory, create template files with the relevant file extension (e.g., `.ejs`, `.pug}). To specify the format and content of the HTML markup, use placeholders and template syntax.
Example:
- Create an `index.js` file:
- Create a `views` directory with an `index.ejs` file inside:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Demo Project</title>
</head>
<body>
<h1>Welcome to Demo Project</h1>
<ul id="item-list">
<% items.forEach((item) => { %>
<li><%= item %></li>
<% }); %>
</ul>
<form id="add-form">
<input type="text" id="item-input" placeholder="Enter item">
<button type="submit">Add Item</button>
</form>
<script src="app.js"></script>
</body>
</html>
Javascript
const express = require('express');
const app = express();
const PORT = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Routes
app.get('/', (req, res) => {
const items = ['Item 1', 'Item 2', 'Item 3']; // Sample items
res.render('index', { items });
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Steps to run the App:
node index.js
Output:
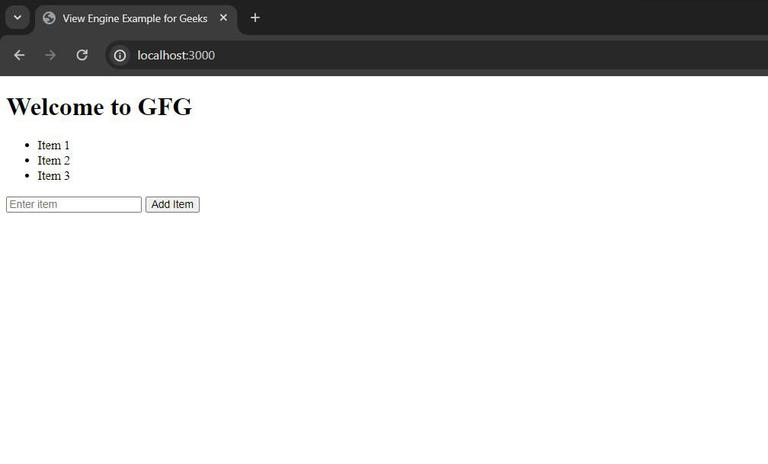
Dynamically rendered web page
Conclusion:
In Express.js, view engines are essential for: – Generating dynamic content on the fly – Splitting code into logical sections – Simplifying and speeding up development – Inheritance and customization of templates – Enhancing website layouts By using view engines in your Express.js projects, you can create modern, full-featured web applications more easily. This leads to code that is more adaptable, manageable, and efficient.
Share your thoughts in the comments
Please Login to comment...