Express res.render() Function
Last Updated :
01 Jan, 2024
The res.render() function in Express is used to render a view and sends the rendered HTML string to the client.Â
Syntax:Â
res.render(view [, locals] [, callback])
Parameters: This function accepts two parameters as mentioned above and described below:Â Â
- Locals: It is an object whose properties define local variables for the view.
- Callback It is a callback function.
Returns: It returns an Object.
Steps to Install the express module:
Step 1: Installing the required modules
npm install express ejs
Step 2: After installing the express module, you can check your express version in the command prompt using the command.
npm version express
Project Structure:
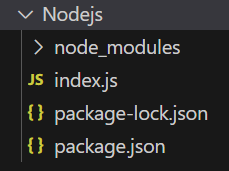
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2",
}
Example 1: Below is the code of res.render() Function implementation.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.set( 'view engine' , 'ejs' );
app.get( '/user' , function (req, res) {
res.render( 'home' );
})
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
HTML
< html >
< head >
< title >res.render() Demo</ title >
</ head >
< body >
< h2 >Welcome to GeeksforGeeks</ h2 >
</ body >
</ html >
|
Steps to run the program:Â
Run the index.js file using the below command:Â
node index.js
Console Output:Â
Server listening on PORT 3000
Browser Output:
Now open the browser and go to http://localhost:3000/user, you can see the following output on your screen:Â
Welcome to GeeksforGeeks
Example 2: Below is the code of res.render() Function implementation.
Javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.set( 'view engine' , 'ejs' );
app.use( '/' , function (req, res, next) {
res.render( 'User' )
next();
});
app.get( '/' , function (req, res) {
console.log( "Render Working" )
res.send();
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
HTML
< html >
< head >
< title >res.render() Demo</ title >
</ head >
< body >
< h2 >Render Function Demo</ h2 >
</ body >
</ html >
|
Steps to run the program:
Run the index.js file using the below command:Â
node index.js
Console Output: After running the above command, you will see the following output on your console screen:Â Â
Server listening on PORT 3000
Render Working
Browser Output: Now open the browser and go to http://localhost:3000, you can see the following output on your screen:Â Â
Render Function Demo
Share your thoughts in the comments
Please Login to comment...