Express JS res.redirect() Function
Last Updated :
25 Dec, 2023
The res.redirect() function redirects to the URL derived from the specified path, with specified status, an integer (positive) which corresponds to an HTTP status code. The default status is “302 Found”.
Syntax:
res.redirect([status] path)
Parameter: This function accepts two parameters as mentioned above and described below:
- status: This parameter holds the HTTP status code
- path: This parameter describes the path.
Return Value: It returns an Object.
Type of paths we can enter:
- The path can be relative to the root of the hostname e.g : /user with relative to http://hostname/user/cart will redirect to http://hostname/user
- The path can be relative to the current URL e.g: /addtocart with relative to http://hostname/user/ will redirect to http://hostname/user/addtocart
Steps to Install the express module:
Step 1: You can install this package by using this command.
npm install express
Step 2: After installing the express module, you can check your express version in the command prompt using the command.
npm version express
Step 3: After that, you can just create a folder and add a file, for example, index.js.
Project Structure:
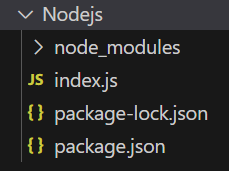
Project Structure
Example 1: Below is the code example of the res.redirect().
javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.get( '/' , function (req, res) {
res.redirect( '/user' );
});
app.get( '/user' , function (req, res) {
res.send( "Redirected to User Page" );
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
Steps to run the program:
Run the index.js file using the below command:
node index.js
Output: Now open the browser and go to http://localhost:3000/, now check your console and you will see the following output:
Server listening on PORT 3000
Browser Output: go to http://localhost:3000/user
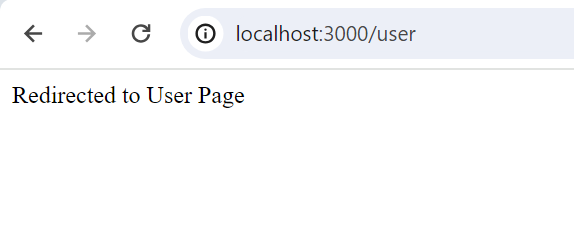
Output
Example 2: Below is the code example of the res.redirect().
javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use( '/verify' , function (req, res, next) {
console.log( "Authenticate and Redirect" )
res.redirect( '/user' );
next();
});
app.get( '/user' , function (req, res) {
res.send( "User Page" );
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
Steps to run the program:
Run the index.js file using the below command:
node index.js
Output: Now open the browser and go to http://localhost:3000/verify, now check your console and you will see the following output:
Server listening on PORT 3000
Authenticate and Redirect
Browser Output:
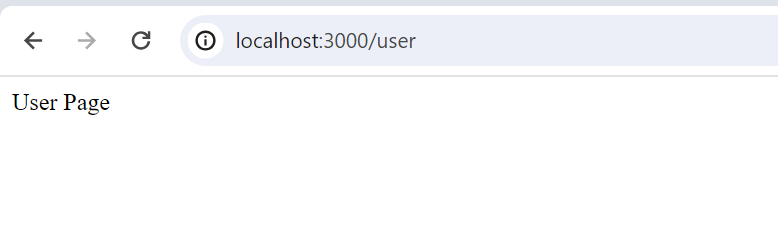
Output
Share your thoughts in the comments
Please Login to comment...