Get the numeric part of CSS property using jQuery
Last Updated :
13 Feb, 2023
Given a HTML document, The task is to get the numeric part of the CSS properties. Like if margin-top = 10px we want to extract only 10. Here few of the methods discussed. First few methods to understand.
- jQuery on() Method This method adds one or more event handlers for the selected elements and child elements. Syntax:
$(selector).on(event, childSel, data, fun, map)
- Parameters:
- event: This parameter is required. It specifies one or more than one event(s) or namespaces to add to the selected elements. If there are multiple event values, separate them by space. Event must be a valid.
- childSel: This parameter is optional. It specifies that the event handler should only be attached to the defined child elements.
- data: This parameter is optional. It specifies additional data to pass to the function.
- fun: This parameter is required. It specifies the function to run when the event occurs.
- map: It specifies an event map ({event:func(), event:func(), …}) having one or more event to add to the selected elements, and functions to run when the events happens.
- jQuery text() Method This method set/return the text content of the selected elements. If this method is used to return content, it provides the text content of all matched elements (HTML tags will be removed). If this method is used to set content, it replace the content of ALL matched elements. Syntax:
$(selector).text()
$(selector).text(content)
- Set text content using a function:
$(selector).text(function(index, curContent))
- content: This parameter is required. It specifies the new text content for the selected elements.
- function(index, curContent): This parameter is optional. It specifies a function that returns the new text content for the selected elements.
- index: It returns the index position of the element in the set.
- curContent: It returns current content of selected elements.
- jQuery css() Method This method set/return one or more than one style properties for the defined elements. Syntax:
- It returns a CSS Property:
css("propertyname")
css("propertyname", "value")
- It sets Multiple CSS Properties:
css({"propertyname":"value", "propertyname":"value", ...});
- propertyName: It specifies the property of element.
- value: It specifies the value of element.
- replace() method This method searches for strings for a defined value, or a regular expression, and returns a new string with the replaced defined value. Syntax:
string.replace(searchVal, newvalue)
- Parameters:
- searchVal: This parameter is required. It specifies the value, or regular expression, that is going to replace by the new value.
- newvalue: This parameter is required. It specifies the value to replace the search value with.
Example 1: This example selects the element and then extracts its property using .css() method, a RegExp and replace() method.
html
<!DOCTYPE html>
< html >
< head >
< title >
JQuery | Get numeric part of CSS property.
</ title >
< style >
#GFG_UP {
font-size: 17px;
font-weight: bold;
}
#GFG_DOWN {
color: green;
font-size: 24px;
font-weight: bold;
}
button {
margin-top: 20px;
}
</ style >
</ head >
</ script >
< body style="text-align:center;" id="body">
< h1 style="color:green;">
GeeksforGeeks
</ h1 >
< p id="GFG_UP">
</ p >
< button >
click here
</ button >
< p id="GFG_DOWN">
</ p >
< script >
$('#GFG_UP').text('Click on the button to get numeric value from CSS property');
$('button').on('click', function() {
var data = $('button').css('marginTop').replace(/[^-\d\.]/g, '');
$('#GFG_DOWN').text("Value of marginTop property of button = " + data);
});
</ script >
</ body >
</ html >
|
Output:
- Before clicking the button:
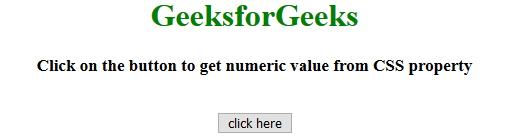
- After clicking the button:
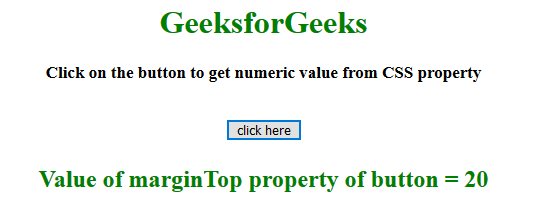
Example 2: This example selects the element with [id = ‘GFG_UP’] and then extracts its fontSize property using .css() method, a RegExp and replace() method.
html
<!DOCTYPE html>
< html >
< head >
< title >
JQuery | Get numeric part of CSS property.
</ title >
< style >
#GFG_UP {
font-size: 17px;
font-weight: bold;
}
#GFG_DOWN {
color: green;
font-size: 24px;
font-weight: bold;
}
button {
margin-top: 20px;
}
</ style >
</ head >
</ script >
< body style="text-align:center;" id="body">
< h1 style="color:green;">
GeeksforGeeks
</ h1 >
< p id="GFG_UP">
</ p >
< button >
click here
</ button >
< p id="GFG_DOWN">
</ p >
< script >
$('#GFG_UP').text('Click on the button to get numeric value from CSS property');
$('button').on('click', function() {
var data = $('#GFG_UP').css('fontSize').replace(/[^-\d\.]/g, '');
$('#GFG_DOWN').text("FontSize property of element[id = 'GFG_UP'] = " + data);
});
</ script >
</ body >
</ html >
|
Output:
- Before clicking the button:
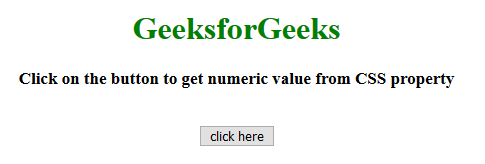
- After clicking the button:
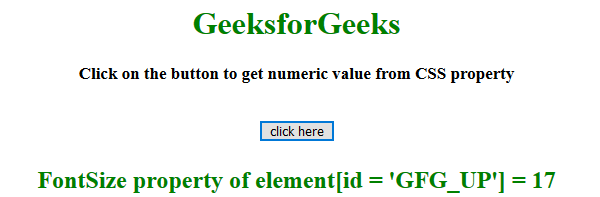
Share your thoughts in the comments
Please Login to comment...