How to Get Current Value of a CSS Property in JavaScript ?
Last Updated :
09 Jan, 2024
In this article, we will see how to get the current value of a CSS property using JavaScript.
Below are the approaches used to Get Current Value of a CSS Property in JavaScript:
Approach 1: Inline Style
Inline styles are styles that are present in the HTML in the style
 attribute. To get inline styles, you can use the style
 property.
Example: In this example, we are getting Inline Style CSS.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Get CSS Property Value - Examples</ title >
< style >
#exampleElement {
width: 200px;
height: 100px;
background-color: #3498db;
color: #fff;
text-align: center;
line-height: 100px;
}
</ style >
</ head >
< body >
< div id = "exampleElement"
style = "background-color: #e74c3c;" >Example Element</ div >
< button onclick = "showColor()" >Show Color</ button >
< p id = "colorDisplay" ></ p >
< script >
function showColor() {
let element3 = document.getElementById("exampleElement");
let backgroundColor3 = element3.style.backgroundColor;
document.getElementById("colorDisplay").innerText += "\nColor: " + backgroundColor3;
}
</ script >
</ body >
</ html >
|
Output:
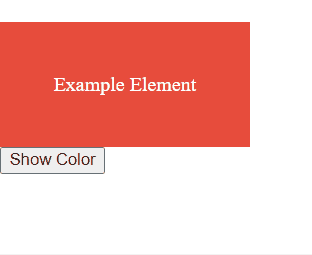
It is used to get all the computed CSS properties and values of the specified element. The use of computed style is displaying the element after styling from multiple sources has been applied. It returns a CSSStyleDeclaration object.
Syntax:
window.getComputedStyle(element, pseudoElement);
Example 1: In this example, we will use the getComputedStyle() method to get the current value of a CSS property.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >
How to Get Current Value of a
CSS Property in JavaScript?
</ title >
< style type = "text/css" id = "GFG" >
body {
text-align: center;
}
h1 {
color: green;
}
#box {
width: 250px;
height: 100px;
line-height: 100px;
color: white;
background-color: rgb(0, 128, 0);
display: block;
margin-left: auto;
margin-right: auto;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h3 >
How to Get Current Value of a
CSS Property in JavaScript?
</ h3 >
< div id = "box" ></ div >
< script >
let id = document.getElementById("box");
let stle = window.getComputedStyle(id);
document.querySelector('div').textContent
= ('background-color: '
+ stle.getPropertyValue('background-color'));
</ script >
</ body >
</ html >
|
Output:
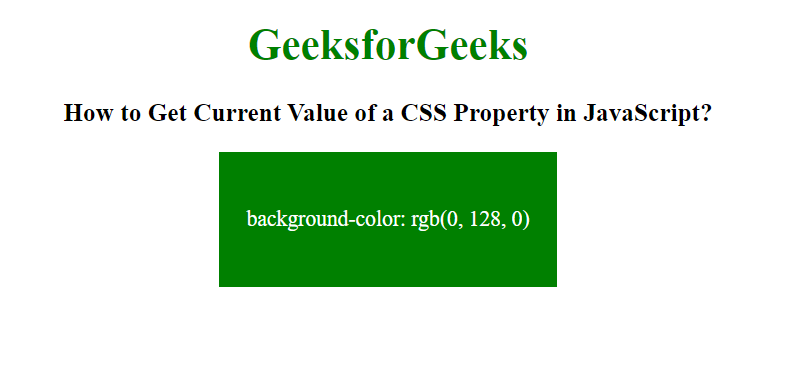
Example 2: In this example, we can get the current value of a CSS property using the window.getComputedStyle() method in JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
How to Get Current Value of a
CSS Property in JavaScript?
</ title >
< style >
body {
text-align: center;
}
#content {
width: 150px;
padding: 30px 20px;
background-color: green;
margin: auto;
}
</ style >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
How to Get Current Value of a
CSS Property in JavaScript?
</ h3 >
< div id = "content" >Welcome to GFG</ div >
< br >
< button onclick = "Fun()" >Get CSS Value</ button >
< pre id = "CSSVal" ></ pre >
< script >
function Fun() {
const element = document.querySelector("#content");
const value = window.getComputedStyle(element)
.getPropertyValue('background-color');
document.getElementById("CSSVal").innerHTML = value;
}
</ script >
</ body >
</ html >
|
Output:
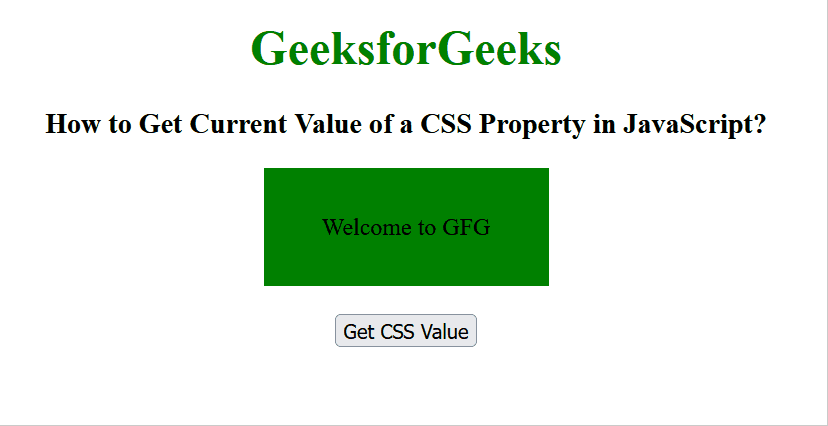
Share your thoughts in the comments
Please Login to comment...