Force Sensitive Resistor ( FSR ) with Arduino
Last Updated :
30 Dec, 2022
In this article, we will learn about Force sensors and the measurement of force sensors using Arduino.
Arduino is an open-source electronics platform. It consists ATmega328P 8-bit Microcontroller. It can be able to read inputs from different sensors & we can send instructions to the microcontroller in the Arduino. It provides Arduino IDE to write code & connect the hardware devices like Arduino boards & sensors.
Force Sensitive Resistor ( FSR):
Force Sensitive Resistor is a transducer that converts mechanical forces like weight, tension, pressure, and compression into an electrical signal. It is widely used in weight measurement. As the force applied to the sensor increases, the resistance of the sensor decreases.
Components Required:
- Arduino Uno R3
- Force sensor
- 5 x LEDs
- 10K resistor
- 100 Ohms resistor
- Jumper wire
Circuit diagram:
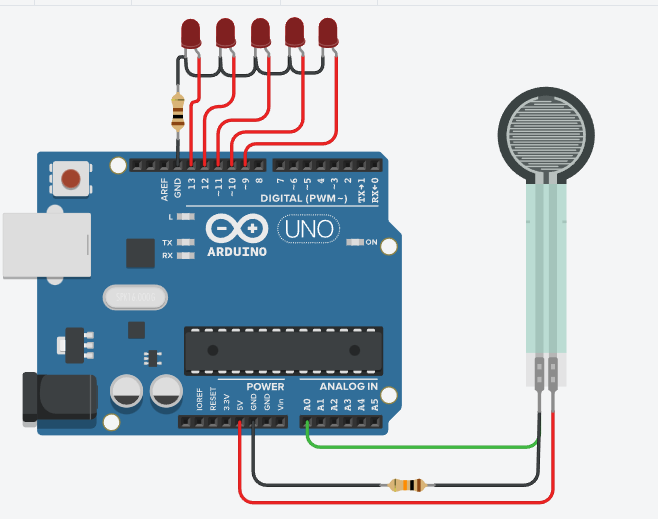
Force Sensor with Arduino circuit
Setup:
- Connect 5V of Arduino to one terminal of the FSR sensor.
- Connect another terminal of the FSR sensor to the Arduino Analog pin directly GND pin with a 10K resistor.
- Connect Level 1 LED to Digital Pin 13.
- Connect Level 2 LED to Digital Pin 12.
- Connect Level 3 LED to Digital Pin 11.
- Connect Level 4 LED to Digital Pin 10.
- Connect Level 5 LED to Digital Pin 9.
- Connect the negative terminal of all the LEDs to the GND pin with a Resistance of 100 Ohm.
- Upload the code to Arduino Uno Board using Arduino IDE.
- Set the Serial monitor to a 9600 baud rate.
Analog to Digital conversion:
Analog Read output = (Input Voltage / 5 ) * 1024
Level indication:
Level |
Analog value range |
1 |
0 to 200 |
2 |
200 to 400 |
3 |
400 to 600 |
4 |
600 to 800 |
5 |
800 to 1023 |
Arduino code:
C++
void setup() {
Serial.begin(9600);
pinMode(13,OUTPUT);
pinMode(12,OUTPUT);
pinMode(11,OUTPUT);
pinMode(10,OUTPUT);
pinMode(9,OUTPUT);
}
void loop() {
int level;
level = analogRead(0);
Serial.println( "Analog value:" );
Serial.println(level);
digitalWrite(13,LOW);
digitalWrite(12,LOW);
digitalWrite(11,LOW);
digitalWrite(10,LOW);
digitalWrite(9,LOW);
if (level<200)
{
digitalWrite(13,HIGH);
}
else if (level<400)
{
digitalWrite(12,HIGH);
}
else if (level<600)
{
digitalWrite(11,HIGH);
}
else if (level<800)
{
digitalWrite(10,HIGH);
}
else if (level<1023)
{
digitalWrite(9,HIGH);
}
}
|
Output:
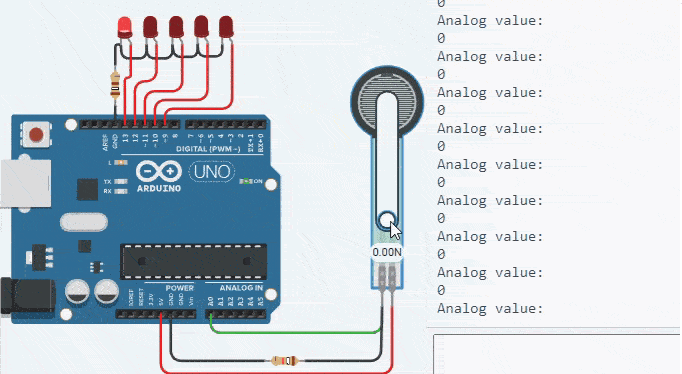
Force sensor Output
Applications:
- Weight measurement
- Pressure measurement
Share your thoughts in the comments
Please Login to comment...