FlushError in Django
Last Updated :
19 Jan, 2024
In this article we will discuss Flushing in Django we will dive into the all-important concept related to Flush and also we will discuss the FlushError reason that occurs in Django and the approaches to solve it.
Flushing in Django
Flushing a database means getting rid of all the information stored in it. It’s like starting from scratch and erasing everything that was saved in the database tables. This is important in situations like when you’re working on developing or testing a project, or when you need to handle data that’s private or important. In Django, there’s a handy feature called the ‘flush()’ method that makes it easy to reset the database by removing all its records in Python.
Below, is the flush
command for resetting the database by removing all data from the tables.
python manage.py flush
Implement Flushing in Django
Here is the step-by-step implementation of the Flushing in Django.
To install Django follow these steps.
Starting the Project Folder
To start the project and app use this command
django-admin startproject core
cd core
python manage.py startapp home
Now add this app to the ‘settings.py’.
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"home",
]
File Structure
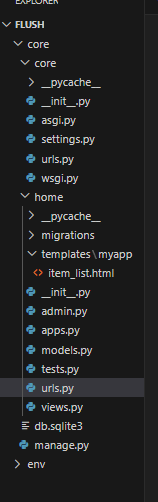
Setting Necessary Files
models.py: In below , Django model defines an ‘Item’ with ‘name’ and ‘description’ fields, both specifying their respective data types. The `__str__` method determines the string representation of the model instance, using its name.
Python3
from django.db import models
class Item(models.Model):
name = models.CharField(max_length = 255 )
description = models.TextField()
def __str__( self ):
return self .name
|
views.py:Â In below code, Django view function, ‘item_list’, retrieves all ‘Item’ objects from the database, and renders the ‘myapp/item_list.html’ template with the ‘items’ context variable for display.
Python3
from django.shortcuts import render
from .models import Item
def item_list(request):
items = Item.objects. all ()
return render(request, 'myapp/item_list.html' , { 'items' : items})
|
project/urls.py : In below code Django URL configuration sets up paths for the admin panel and includes URLs from the ‘home’ app for the root URL.
Python3
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' home.urls')),
]
|
app/urls.py : In below code, Django URL configuration defines a path ‘items/’ that maps to the ‘item_list’ view in the ‘home’ app, with the namespace ‘home’ for reverse URL lookups.
Python3
from django.urls import path
from .views import item_list
app_name = 'home'
urlpatterns = [
path( 'items/' , item_list, name = 'item_list' ),
]
|
Creating GUI
myapp/item_list.html : In below, HTML template (`item_list.html`) renders a page titled “Item List” with an unordered list (`ul`) displaying each item’s name and description retrieved from the Django view using a for loop (`{% for item in items %}`).
HTML
<!DOCTYPE html>
< html >
< head >
< title >Item List</ title >
</ head >
< body >
< h1 >Item List</ h1 >
< ul >
{% for item in items %}
< li >{{ item.name }} - {{ item.description }}</ li >
{% endfor %}
</ ul >
</ body >
</ html >
|
admin.py:Here we are registering our models.
Python3
from django.contrib import admin
from .models import Item
admin.site.register(Item)
|
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Flush Commands for Flushing the Data in Django
python3 manage.py flush
Output :
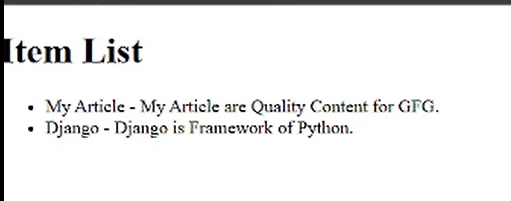
How to Solve FlushError in Django?
Answer : To fix the flushing issue in Django, you can use a simple method. Just run two commands to update the database and create a superuser for accessing the admin panel. When you use the flush command, it removes the table and all the database. To recover the database, run the migration commands and create the superuser again. Here are the commands:
python3 manage.py makemigrations
python3 manage.py migrate
python3 manage.py createsuperuser
Conclusion
In conclusion, addressing the django.db.transaction.exceptions.FlushError in Django involves implementing strategic approaches. Utilizing select_for_update to lock records during transactions helps mitigate concurrency issues, while handling unique constraint violations through exception handling ensures data integrity. Additionally, avoiding circular dependencies in model relationships within transactions prevents the occurrence of this error.
Share your thoughts in the comments
Please Login to comment...