Find the sum of n terms of the series 1 , 2a , 3a2 , 4a3 , 5a4 , …
Last Updated :
30 Aug, 2022
Given a series .
and the value of a. Find the sum of the first n term of the series.
Examples:
Input: a = 3, n = 4
Output: 142
Input: a = 5, n = 1
Output: 1
Brute Force Approach:
A simple approach can be iterating N terms of the series and adding them to calculate the sum for any value of a. Follow the steps below to understand the approach:
For each iteration:
- Calculate an [ n = 0 ].
- Multiply an with (n+1).
- Add (n+1)*an to sum and increment n by 1.
- Repeat the above processes n times.
Illustration:
a = 3 and n = 4
Loop will be executed n number of times i.e 4 in this case.
Loop 1: Initially the value of a = 1, n = 0, sum = 0
- an = 30
= 1 - an * (n+1) = 30 * (0+1)
= 1 * (1)
= 1 - sum = sum + an * (n+1)
= 0 + 1
= 1 - Increment n by 1.
Loop 2: The value of a = 3, n = 1, sum = 1
- an = 31
= 3 - an * (n+1) = 31 * (1+1)
= 3 * (2)
= 6 - sum = sum + an * (n+1)
= 1 + 6
= 7 - Increment value of n by 1.
Loop 3: The value of a = 3, n = 2, sum = 7
- an = 32
= 9 - an * (n+1) = 32 * (2+1)
= 9 * (3)
= 27 - sum = sum + an * (n+1)
= 7 + 27
= 34 - Increment n by 1.
Loop 4: The value of a = 3, n = 3, sum = 34
- an = 33
= 27 - an * (n+1) = 33 * (3+1)
= 27 * (4)
= 108 - sum = sum + an * (n+1)
= 34 + 108
= 142 - Increment the value of n by 1.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void calcSum( int a, int n)
{
if (n < 0)
{
cout << "Invalid Input" ;
return ;
}
if (a == 0 || n == 1)
{
cout << 1;
return ;
}
int Sum = 0;
for ( int i = 0; i < n; i++)
{
int r = pow (a, (i)) * (i + 1);
Sum += r;
}
cout << Sum;
}
int main()
{
int a = 3;
int n = 4;
calcSum(a, n);
return 0;
}
|
Java
import java.util.*;
class GFG{
static void calcSum( int a, int n)
{
if (n < 0 )
{
System.out.print( "Invalid Input" );
return ;
}
if (a == 0 || n == 1 )
{
System.out.print( 1 );
return ;
}
int Sum = 0 ;
for ( int i = 0 ; i < n; i++)
{
int r = ( int ) (Math.pow(a, (i)) * (i + 1 ));
Sum += r;
}
System.out.print(Sum);
}
public static void main(String[] args)
{
int a = 3 ;
int n = 4 ;
calcSum(a, n);
}
}
|
Python3
def calcSum(a, n):
if (n < 0 ):
print ( "Invalid Input" )
return
if (a = = 0 or n = = 1 ):
print ( 1 )
return
Sum = 0
for i in range (n):
r = pow (a, (i)) * (i + 1 )
Sum + = r
print ( Sum )
if __name__ = = "__main__" :
a = 3
n = 4
calcSum(a, n)
|
C#
using System;
using System.Collections;
class GFG {
static void calcSum( int a, int n)
{
if (n < 0)
{
Console.Write( "Invalid Input" );
return ;
}
if (a == 0 || n == 1)
{
Console.Write(1);
return ;
}
int Sum = 0;
for ( int i = 0; i < n; i++)
{
int r = ( int )Math.Pow(a, (i)) * (i + 1);
Sum += r;
}
Console.Write(Sum);
}
public static void Main()
{
int a = 3;
int n = 4;
calcSum(a, n);
}
}
|
Javascript
<script>
function calcSum(a, n)
{
if (n < 0)
{
document.write( "Invalid Input" );
return ;
}
if (a == 0 || n == 1)
{
document.write(1);
return ;
}
let Sum = 0;
for (let i = 0; i < n; i++)
{
let r = Math.pow(a, (i)) * (i + 1);
Sum += r;
}
document.write(Sum);
}
let a = 3;
let n = 4;
calcSum(a, n);
</script>
|
Output:
142
Time Complexity: O(nlogn) since it is using pow function inside a for loop
Auxiliary Space: O(1)
Efficient Approach
In this approach, an efficient solution is proposed using the concept of Geometric progression. The sum of the series of n terms in a Geometric Progression (G.P.) with first term a and common ratio r is given as:
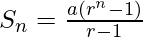
Let’s use this concept to reach a solution to the problem.
Let 
Clearly nth term is 
. (1)
Multiply both sides with ‘a’, we get,

(2)
Subtracting equation (2) from (1), we get


Clearly this is the Geometric Progression (G.P.) of n terms with first term 1 and common ration a.
G.P. of n terms with first term a and common ratio r is:
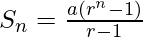
Using the above formula, we have

Dividing both sides by (1 – a), we get



Therefore, the sum of the series
is
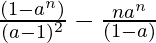
For a != 1 the formula for sum of the series is:
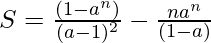
For a = 1 the formula for sum of the series is:
The series reduces to sum of first n natural numbers and the formula becomes-
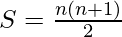
Illustration:
For a = 3, n = 4
Since a != 1, therefore use the formula
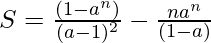
Substituting the values of a and n in the above formula, we get
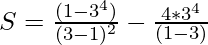

S = -20 – (-162)
S = 142
So, the sum of the series
with value of a = 3 and n = 4 is 142.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void calcSum( int a, int n)
{
if (n < 0)
{
cout << "Invalid Input" ;
return ;
}
if (a == 0 || n == 1)
{
cout << 1;
return ;
}
if (a == 1)
{
if (n % 2 == 0)
cout << (n / 2) * (n + 1);
else
cout << ((n + 1) / 2) * n;
}
int r = pow (a, n);
int d = pow (a - 1, 2);
int Sum = (1 - r * (1 + n - n * a)) / d;
cout << Sum;
}
int main()
{
int a = 3;
int n = 4;
calcSum(a, n);
return 0;
}
|
Java
class GFG {
public static void calcSum( int a, int n)
{
if (n < 0 ) {
System.out.println( "Invalid Input" );
return ;
}
if (a == 0 || n == 1 ) {
System.out.println( 1 );
return ;
}
if (a == 1 ) {
if (n % 2 == 0 )
System.out.println((n / 2 ) * (n + 1 ));
else
System.out.println(((n + 1 ) / 2 ) * n);
}
int r = ( int ) Math.pow(a, n);
int d = ( int ) Math.pow(a - 1 , 2 );
int Sum = ( 1 - r * ( 1 + n - n * a)) / d;
System.out.println(Sum);
}
public static void main(String args[]) {
int a = 3 ;
int n = 4 ;
calcSum(a, n);
}
}
|
Python3
def calcSum(a, n):
if (n < 0 ):
print ( "Invalid Input" );
return ;
if (a = = 0 or n = = 1 ):
print ( 1 );
return ;
if (a = = 1 ):
if (n % 2 = = 0 ):
print ((n / / 2 ) * (n + 1 ));
else :
print (((n + 1 ) / / 2 ) * n);
r = pow (a, n);
d = pow (a - 1 , 2 );
Sum = ( 1 - r * ( 1 + n - n * a)) / / d;
print ( Sum );
if __name__ = = '__main__' :
a = 3 ;
n = 4 ;
calcSum(a, n);
|
C#
using System;
class GFG {
public static void calcSum( int a, int n)
{
if (n < 0) {
Console.WriteLine( "Invalid Input" );
return ;
}
if (a == 0 || n == 1) {
Console.WriteLine(1);
return ;
}
if (a == 1) {
if (n % 2 == 0)
Console.WriteLine((n / 2) * (n + 1));
else
Console.WriteLine(((n + 1) / 2) * n);
}
int r = ( int ) Math.Pow(a, n);
int d = ( int ) Math.Pow(a - 1, 2);
int Sum = (1 - r * (1 + n - n * a)) / d;
Console.WriteLine(Sum);
}
public static void Main() {
int a = 3;
int n = 4;
calcSum(a, n);
}
}
|
Javascript
<script>
function calcSum(a, n)
{
if (n < 0)
{
document.write( "Invalid Input" );
return ;
}
if (a == 0 || n == 1)
{
document.write(1);
return ;
}
if (a == 1)
{
if (n % 2 == 0)
document.write((n / 2) * (n + 1));
else
document.write(((n + 1) / 2) * n);
}
let r = Math.pow(a, n);
let d = Math.pow(a - 1, 2);
let Sum = (1 - r * (1 + n - n * a)) / d;
document.write(Sum);
}
let a = 3;
let n = 4;
calcSum(a, n);
</script>
|
Output:
142
Time Complexity: O(logn) since it is using pow function pow(a,n)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...