Find the sum of first N terms of the series 2×3 + 4×4 + 6×5 + 8×6 + …
Last Updated :
25 May, 2022
Given an integer N. The task is to find the sum upto N terms of the given series:
2×3 + 4×4 + 6×5 + 8×6 + … + upto n terms
Examples:
Input : N = 5
Output : Sum = 170
Input : N = 10
Output : Sum = 990
Let the N-th term of the series be tN.
t1 = 2 × 3 = (2 × 1)(1 + 2)
t2 = 4 × 4 = (2 × 2)(2 + 2)
t3 = 6 × 5 = (2 × 3)(3 + 2)
t4 = 8 × 6 = (2 × 4)(4 + 2)
.
.
.
tN = (2 × N)(N + 2)
The sum of n terms of the series,
Sn = t1 + t2 +... + tn
=
=
=
=
=
=
=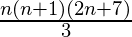
Below is the implementation of above approach:
C++
#include<iostream>
using namespace std;
void Sum_upto_nth_Term( int n)
{
int r = n * (n + 1) *
(2 * n + 7) / 3;
cout << r;
}
int main()
{
int N = 5;
Sum_upto_nth_Term(N) ;
return 0;
}
|
Java
import java.io.*;
class GFG {
static void Sum_upto_nth_Term( int n)
{
int r = n * (n + 1 ) *
( 2 * n + 7 ) / 3 ;
System.out.println(r);
}
public static void main (String[] args) {
int N = 5 ;
Sum_upto_nth_Term(N);
}
}
|
Python3
def Sum_upto_nth_Term(n):
return n * (n + 1 ) * ( 2 * n + 7 ) / / 3
N = 5
print (Sum_upto_nth_Term(N))
|
C#
using System;
class GFG
{
static void Sum_upto_nth_Term( int n)
{
int r = n * (n + 1) *
(2 * n + 7) / 3;
Console.Write(r);
}
public static void Main()
{
int N = 5;
Sum_upto_nth_Term(N);
}
}
|
PHP
<?php
function Sum_upto_nth_Term( $n )
{
$r = $n * ( $n + 1) *
(2 * $n + 7) / 3;
echo $r ;
}
$N = 5;
Sum_upto_nth_Term( $N );
?>
|
Javascript
<script>
function Sum_upto_nth_Term(n)
{
let r = n * (n + 1) *
(2 * n + 7) / 3;
document.write(r);
}
let N = 5;
Sum_upto_nth_Term(N) ;
</script>
|
Time Complexity: O(1), it is a constant.
Auxiliary Space: O(1), no extra space is required.
Share your thoughts in the comments
Please Login to comment...