Find Shortest Path Using Python OSMnx Routing Module
Last Updated :
18 Mar, 2024
This article demonstrates how to use the OSMnx routing module to find the shortest path, illustrated with practical examples.
Syntax of osmnx.routing.shortest_path() Function
OSMnx routing module has the ‘shortest_path’ functionality to find the nearest path from the origin node to the destination node. The function is as follows:
osmnx.routing.shortest_path(G, orig, dest, weight=’length’, cpus=1)
Parameters:
- G (networkx.MultiDiGraph) – input graph
- orig (int or list) – origin node ID, or a list of origin node IDs
- dest (int or list) – destination node ID, or a list of destination node IDs
- weight (string) – edge attribute to minimize when solving shortest path
- cpus (int) – how many CPU cores to use; if None, use all available. It allows parallel processing but make sure not to exceed the available RAM
Returns: path – list of node IDs constituting the shortest path, or, if orig and dest are lists, then a list of path lists
Return Type: List
The shortest_path functionality make use of Dijkstra’s shortest path algorithm to find the shortest path between the origin node and destination node.
Find Shortest Path Using Python OSMnx Routing Module
Let’s implement the functionality to find the shortest path. As a first step we need to get the origin node and destination node. We make use of Thiruvanathapuram city data using osmnx and fetch the origin and destination node w.r.t Kazhakkootam and Medical College respectively. The code as follows:
Python3
import osmnx as ox
# load thiruvananthapuram city
place = "Thiruvananthapuram, Kerala"
G = ox.graph_from_place(place, network_type="drive")
# kazhakootam coordinates
kzh_lat, kzh_lon = 8.5686, 76.8731
# medical college coordinates
mdcl_lat, mdcl_lon = 8.52202892500963, 76.926448394559
# fetch the nearest node w.r.t coordinates
kzh_node = ox.distance.nearest_nodes(G, kzh_lon, kzh_lat)
mdcl_node = ox.distance.nearest_nodes(G, mdcl_lon, mdcl_lat)
print("Kazhakkottam Node: {kzh_node}, \
Medical College Node: {mdcl_node}".format(
kzh_node=kzh_node, mdcl_node=mdcl_node))
Output:
Kazhakkottam Node: 1141007999, Medical College Node: 9992653265
Now we have the nodes. Let’s calculate the shortest distance form Kazhakootam node (origin node) to Medical College node(destination node). The code as shown below:
Python3
import osmnx as ox
place = "Thiruvananthapuram, Kerala"
G = ox.graph_from_place(place, network_type="drive")
# orgin node and destination node
orig, dest = 1141007999, 9992653265
# find shortest path
route_nodes = ox.routing.shortest_path(G, orig, dest, weight="length")
# plot the shortes path
fig, ax = ox.plot_graph_route(G, route_nodes, route_color="r",
route_linewidth=6, node_size=0)
Output:
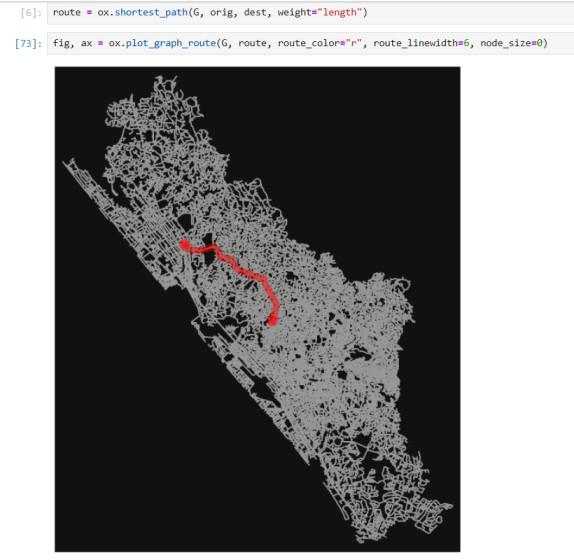
shortest path plot
Let’s plot it on map. We have to reconstruct the edges (u, v, k) based on the shortest route generated from above code. Fetch the graph edges from our multidigraph based on the constructed edges. The graph edges are multiindexed with (u, v, k) params. The code as follows:
Python3
def generate_multindex(route_nodes):
multiindex_list = []
# append the index to list
for u, v in zip(route_nodes[:-1], route_nodes[1:]):
multiindex_list.append((u, v, 0))
return multiindex_list
# get edges from from above multidigraph
gdf_nodes, gdf_edges = ox.graph_to_gdfs(G)
# generate multiindex based on generated shortest route
multiindex_list = generate_multindex(route_nodes)
# fetch edge details based on multi index list
shrt_gdf_edges = gdf_edges[gdf_edges.index.isin(multiindex_list)]
# plot the shortest route on map
shrt_gdf_edges.explore(color="red")
Output:
The path in red color depicts the shortest path from Kazhakkottam to Medical Colledge.
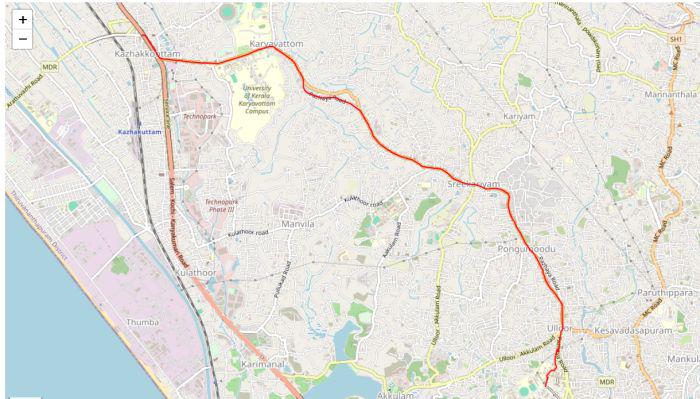
shortest path
Share your thoughts in the comments
Please Login to comment...