Find K Shortest Path Using OSMnx Routing Module in Python
Last Updated :
21 Mar, 2024
OSMnx, a Python library built on top of OpenStreetMap data, offers a powerful set of tools for street network analysis. One common task in network analysis is finding the K shortest paths between two locations. In this article, we’ll explore how to find the k-shortest path using OSMnx module in Python.
Syntax of osmnx.routing.k_shortest_paths() Function
OSMnx routing module has the ‘k_shortest_path’ functionality to find the k nearest path from the origin node to the destination node. The functionality to calculate weighted shortest paths between graph nodes is as follows:
osmnx.routing.k_shortest_paths(G, orig, dest, k, weight=’length’)
Parameters:
- G (networkx.MultiDiGraph) – input graph
- orig (int) – origin node ID
- dest (int) – destination node ID
- k (int) – number of shortest paths to solve
- weight (string) – edge attribute to minimize when solving shortest paths. default is edge length in meters.
Returns:
path (list) – a generator of k shortest paths ordered by total weight. each path is a list of node IDs.
Note: install geopandas==0.14.3, notebook==7.0.7, osmnx==1.9.1, folium==0.15.1, matplotlib==3.8.2, mapclassify==2.6.1
Find K-Shortest Path Between Origin and Destination Node Using OSMnx
Below is the explanation and procedure of the examples by which we can find the k-shortest path using OSMnx module in Python:
Finding Shortest Paths with OSMnx in Piedmont
Here we utilize OSMnx to fetch a street network for Piedmont, California, then find and plot the 30 shortest paths (by distance) between two arbitrary nodes within the network, showcasing the versatility of OSMnx for routing analysis in Python.
Python3
import osmnx as ox
place = "Piedmont, California, USA"
G = ox.graph_from_place(place, network_type="drive")
# find the shortest path (by distance)
# between these nodes then plot it
orig = list(G)[0]
dest = list(G)[140]
# find k-shortest path
routes = ox.k_shortest_paths(G, orig, dest, k=30, weight="length")
fig, ax = ox.plot_graph_routes(
G, list(routes), route_colors="y",
route_linewidth=4, node_size=0)
Output
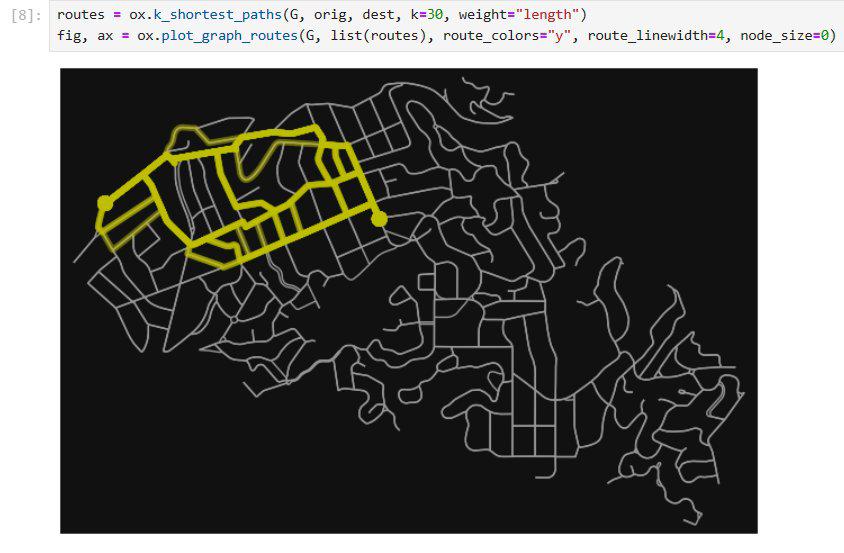
k-shortest path
Visualizing Multiple Shortest Paths in Piedmont, California, USA
Let’s plot it on map. We have to reconstruct the edges (u, v, k) based on the shortest routes. Fetch the graph edges from our multidigraph based on the constructed edges. The graph edges are multiindexed with (u, v, k) params. In this example, we use OSMnx to retrieve the street network data for Piedmont, California, USA. Then, we find the 30 shortest paths between two arbitrary nodes within the network and generate a MultiIndex based on the edges of these routes. Finally, we plot these shortest paths on the map, highlighting them in red for visualization.
Python3
import osmnx as ox
def generate_multindex(route_nodes):
multiindex_list = []
# append the index to list
for u, v in zip(route_nodes[:-1], route_nodes[1:]):
multiindex_list.append((u, v, 0))
return multiindex_list
# load the map data
place = "Piedmont, California, USA"
G = ox.graph_from_place(place, network_type="drive")
# find the shortest path (by distance)
# between these nodes then plot it
orig = list(G)[0]
dest = list(G)[140]
# fetch k shortest path
routes_nodes = ox.k_shortest_paths(G, orig, dest, k=30, weight="length")
# get edges from from above multidigraph
gdf_nodes, gdf_edges = ox.graph_to_gdfs(G)
# generate multiindex based on generated shortest route
routes_index_list = []
for route_nodes in routes_nodes:
multiindex_list = generate_multindex(route_nodes)
routes_index_list.extend(multiindex_list)
# fetch edge details based on multi index list
shrt_gdf_edges = gdf_edges[gdf_edges.index.isin(routes_index_list)]
# plot the shortest route on map
shrt_gdf_edges.explore(color="red")
Output
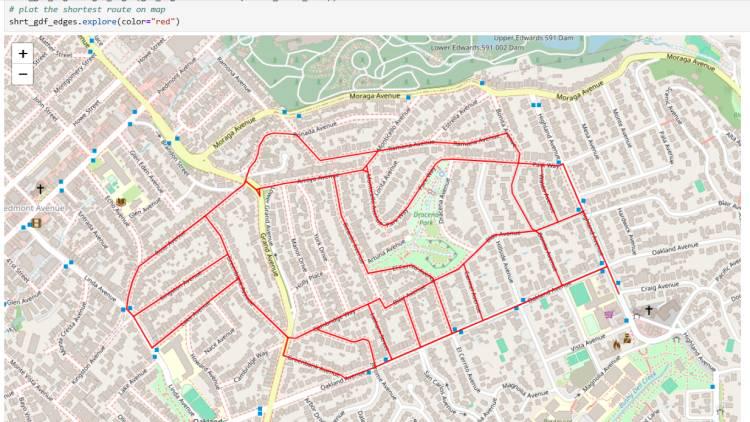
k-shortest path
Share your thoughts in the comments
Please Login to comment...