Calculate Compass Bearing Using Python OSMnx Bearing Module
Last Updated :
20 Mar, 2024
Bearing is a significant angle for Geographic Information System (GIS) applications. In this article, we will see how to calculate the compass Bearing using the OSMnx bearing module based on lat-Ion Points.
What is Bearing?
Bearing represents the clockwise angle in degrees between the north and the geodesic line from (lat1, lon1) to (lat2, lon2). It is used to define navigation commonly in the fields of street network modeling, vehicle navigation, aircraft or marine navigation, or land surveying. Bearings are always measured from the North, in a clockwise direction. Refer to the below diagram:
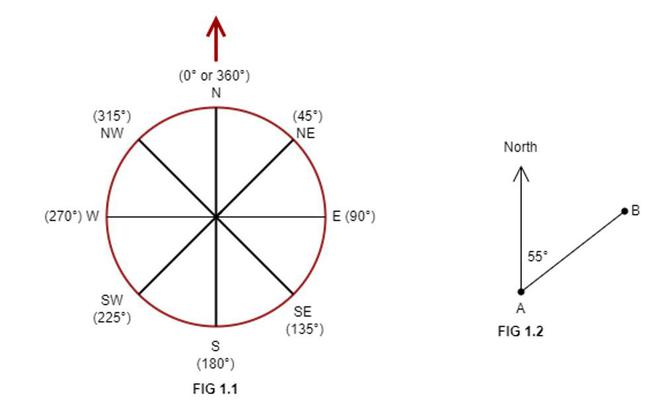
Bearing measured from North
- FIG 1.1 depicts the different bearings measured from north in clockwise direction.
- FIG 1.2 depicts, B bears 55° from A when measured from North in clockwise direction. A and B can be replaced with actual coordinates.
Syntax of osmnx.bearing.calculate_bearing() Function
osmnx.bearing.calculate_bearing(lat1, lon1, lat2, lon2)
Parameters:
- lat1 (float or numpy.array of float) – first point’s latitude coordinate
- lon1 (float or numpy.array of float) – first point’s longitude coordinate
- lat2 (float or numpy.array of float) – second point’s latitude coordinate
- lon2 (float or numpy.array of float) – second point’s longitude coordinate
Returns: bearing – the bearing(s) in decimal degrees
Return Type: float or numpy.array of float
Calculate Compass Bearing Using Python OSMnx Bearing Module
Below is the step-by-step explanation to calculate compass bearing using OSMnx bearing Module in Python:
Step 1: Create GeoDataFrame Using Coordinates and Display Map
Before calculating the bearing, let’s look at the coordinates on the map. below code uses GeoPandas to create a GeoDataFrame from a dictionary of coordinates representing Thiruvananthapuram and Kollam. It then displays the map of these locations.
Python3
import geopandas as gd
from shapely.geometry import Point
# coordinates
tvm_lat, tvm_lon = 8.50606, 76.96153
kol_lat, kol_lon = 8.88795, 76.59550
coordinate_dict = {'name': ['Thiruvananthapuram', 'Kollam'],
'geometry': [Point(tvm_lon, tvm_lat),
Point(kol_lon, kol_lat)]}
# convert corrdinate dictionary to geo dataframe
gdf = gd.GeoDataFrame(coordinate_dict, crs="EPSG:4326")
# displays the map in jupyter
gdf.explore()
Output
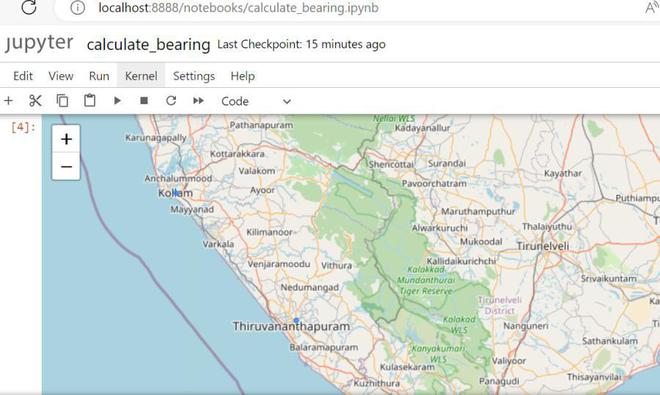
Plotting the coordinates
Step 2: Calculate Bearing Between Two Coordinates Using OSMnx Module
Let’s calculate the bearing for the given lat-lon points. Below code uses OSMnx to calculate the bearing between two sets of coordinates representing Thiruvananthapuram and Kollam. It computes the bearing using the calculate_bearing() function and returns the result.
Python3
import osmnx as ox
# coordinates
tvm_lat, tvm_lon = 8.50606, 76.96153
kol_lat, kol_lon = 8.88795, 76.59550
# calculate bearing
bearing = ox.bearing.calculate_bearing(tvm_lat, tvm_lon, kol_lat, kol_lon)
bearing
Output
316.57324725701557
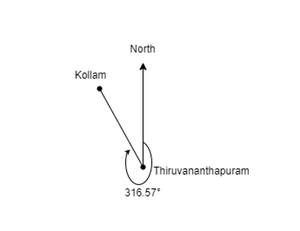
Bearing
The bearing value is 316.57°, which means Kollam bears 316.57° from Thiruvananthapuram.
Now we are familiar with bearing calculation based on latitude and longitude. Let’s see how to pass array of coordinates and calculate the bearings.
Calculate Bearings for Multiple Coordinate Pairs
Below code uses OSMnx and NumPy to compute bearings between Thiruvananthapuram, Kollam, and Pathanamthitta coordinates. It employs calculate_bearing() to determine bearings between Thiruvananthapuram and Kollam, and between Kollam and Pathanamthitta, returning the results.
Python3
import osmnx as ox
import numpy as np
# Thiruvananthapuram coordinates
tvm_lat, tvm_lon = 8.50606, 76.96153
# Kollam coordinates
kol_lat, kol_lon = 8.88795, 76.59550
# Pathanamthitta coordinates
pat_lat, pat_lon = 9.2648, 76.7870
# Calculate bearing for (trivandrum, kollam)
# and (kollam , pathanamthitta)
from_lat = np.array([tvm_lat, kol_lat])
from_lon = np.array([tvm_lon, kol_lon])
to_lat = np.array([kol_lat, pat_lat])
to_lon = np.array([kol_lon, pat_lon])
# calculate bearing
bearing = ox.bearing.calculate_bearing(from_lat, from_lon, to_lat, to_lon)
bearing
Output
array([316.57324726, 26.63232046])
Here we take two set of coordinates – (Thiruvanathapuram and Kollam), (Kollam and Pathanamthitta) From the output we can conclude, Kollam bears 316.57° from Thiruvananthapuram and Pathanamthitta bears 26.63° from Kollam.
Share your thoughts in the comments
Please Login to comment...