Explain different types of Postman Assertions
Last Updated :
05 Jan, 2024
Postman is a popular API development and testing tool that allows developers to design, test, and document APIs. One crucial aspect of API testing in Postman is the use of assertions, which are validations performed on the responses received from API requests. Assertions help ensure that the API behaves as expected and that the data exchanged is accurate. In this article, we will delve into different types of assertions in Postman, discussing their significance and how to implement them.
There are several approaches to implementing assertions in Postman, each serving a unique purpose. The main assertion types include:
Status Code Assertions:
Status Code Assertions are fundamental validations that ensure the server responds with the expected HTTP status code. They help identify if the request was successful or encountered an error.
To implement a Status Code Assertion:
- 1. Create a request in Postman.
- 2. Go to the “Tests” tab.
- 3. Write the assertion code using the `pm.response` object.
Javascript
pm.test( "Status code is 200 OK" , function () {
pm.response.to.have.status(200);
});
|
Output:
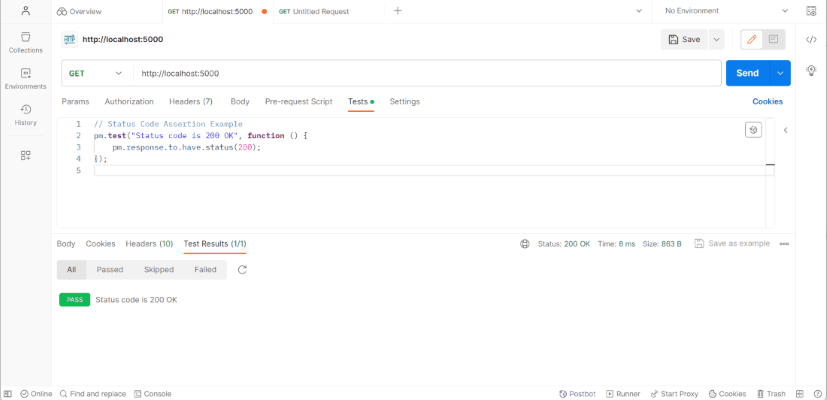
Status Code Assertions
Response Body Assertions:
These assertions validate the content of the response body. You can check for specific values, patterns, or elements within the response.
To implement a Response Body Assertion:
- 1. Access the “Tests” tab.
- 2. Write assertions using the `pm.response` object.
Javascript
pm.test( "Response body should contain user with ID 1" , function () {
var jsonData = pm.response.json();
pm.expect(jsonData).to.have.property( 'user' )
.to.have.property( 'id' ).to.eql(1);
});
|
Output:
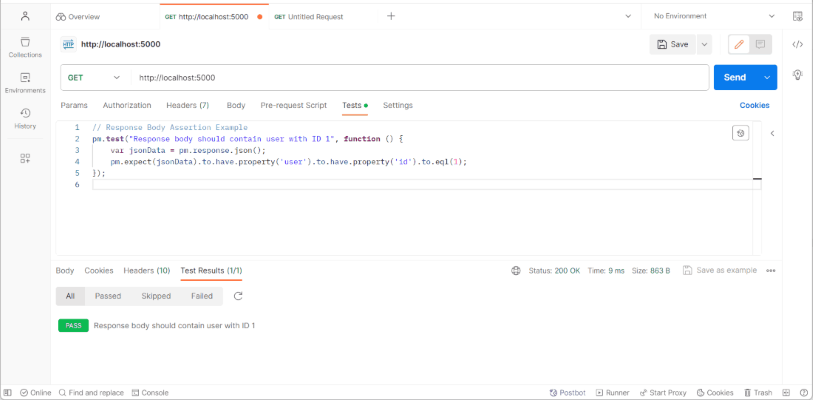
Response Body Assertions
Header Assertions validate the presence and values of headers in the API response.
To implement a Header Assertion:
- 1. Navigate to the “Tests” tab.
- 2. Write assertions using the `pm.response` object.
Javascript
pm.test( "Content-Type header is present and has value 'application/json'" ,
function () {
pm.response.to.have.header( "Content-Type" );
pm.response.to.have.header( "Content-Type" , "application/json" );
});
|
Output:
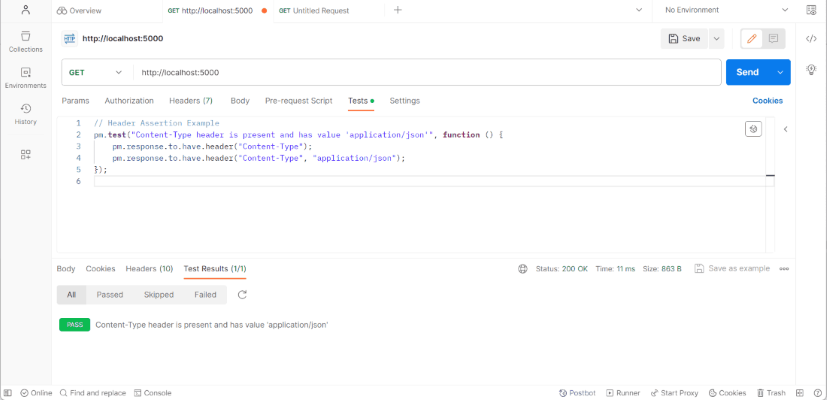
Header Assertions
JSON Schema Assertions
JSON Schema Assertions ensure that the response adheres to a specified JSON schema.
To implement a JSON Schema Assertion:
- 1. Go to the “Tests” tab.
- 2. Write assertions using the `tv4` library.
Javascript
const schema = {
"type" : "object" ,
"properties" : {
"user" : { "type" : "object" }
},
"required" : [ "user" ]
};
pm.test( "Response body adheres to JSON schema" , function () {
pm.response.to.have.jsonSchema(schema);
});
|
Output:
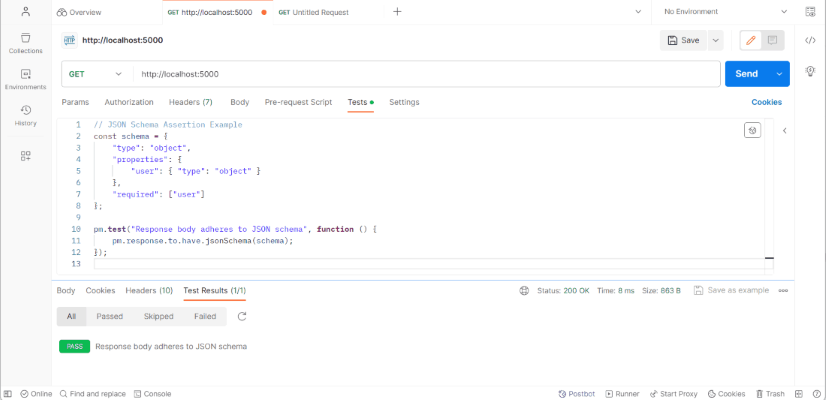
JSON Schema Assertions
XML Assertions:
XML Assertions validate the structure and content of XML responses.
To implement an XML Assertion:
- 1. Access the “Tests” tab.
- 2. Write assertions using the `pm.response` object or external libraries.
Javascript
const xml2js = require( 'xml2js' );
pm.test( "Response body is a valid XML with specific content" , function () {
var xmlResponse = pm.response.text();
xml2js.parseString(xmlResponse, function (err, result) {
pm.expect(result).to.have.property( 'user' )
.to.have.property( 'id' ).to.eql( '1' );
});
});
|
Output:
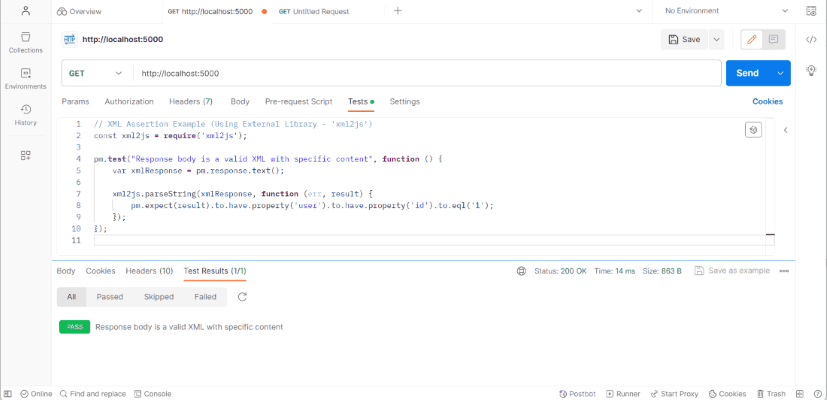
XML Assertions
Share your thoughts in the comments
Please Login to comment...