Draw Colourful Star Pattern in Turtle – Python
Last Updated :
04 Apr, 2023
In this article we will use Python’s turtle library to draw a spiral of stars, filled with randomly generated colours. We can generate different patterns by varying some parameters.
modules required:
turtle:
turtle library enables users to draw picture or shapes using commands, providing them with a virtual canvas.
turtle comes with Python’s Standard Library. It needs a version of Python with Tk support, as it uses tkinter for the graphics.
Explanation:
First we set each of the parameters for the spiral: number of stars, exterior angle of the stars and angle of rotation for the spiral. The colours are chosen randomly by choosing three random integers for rgb values, and so each time we get a different colour combination.
In the implementation below, we will draw a pattern of 30 stars, with exterior angle 144 degrees and angle of rotation 18 degrees.
Python3
from turtle import *
import random
speed(speed = 'fastest' )
def draw(n, x, angle):
for i in range (n):
colormode( 255 )
a = random.randint( 0 , 255 )
b = random.randint( 0 , 255 )
c = random.randint( 0 , 255 )
pencolor(a, b, c)
fillcolor(a, b, c)
begin_fill()
for j in range ( 5 ):
forward( 5 * n - 5 * i)
right(x)
forward( 5 * n - 5 * i)
right( 72 - x)
end_fill()
rt(angle)
n = 30
x = 144
angle = 18
draw(n, x, angle)
|
Output:
By changing the exterior angle to 72, we can get a pattern of pentagons as such:
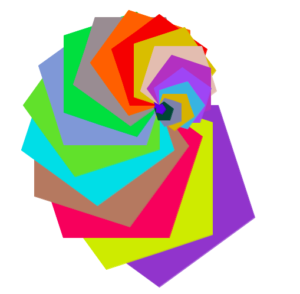
20 pentagons, 18 degree spiral
Share your thoughts in the comments
Please Login to comment...