Download button to download data stored in the database using Django
Last Updated :
20 Oct, 2023
In this article, We will guide you through the process of adding download buttons to retrieve data stored in the database using Django. Whether you are building a content management system, or an e-commerce system, allowing users to download data is a common and essential feature.
Setting up the Project
To install Django follow these steps.
Starting the Project Folder
To start the project use this command
django-admin startproject dload
cd dload
To start the app use this command
python manage.py startapp filedownloadapp
Now add this app to the ‘settings.py’
File Structure
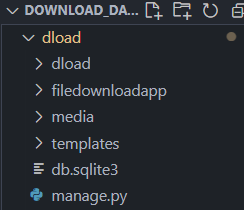
Project structure
Setting up the Files
models.py: To store files with file title, and file itself we need a database table. In filedownloadapp/models.py file create Cheatsheet folder. This defines we need title and its length should be max 100. file and the path to store the file. Whenever file gets re uploaded uploaded_at field also gets updated with the current time.
Python3
from django.db import models
class Cheatsheet(models.Model):
title = models.CharField(max_length = 100 )
file = models.FileField(upload_to = 'cheatsheets/' )
uploaded_at = models.DateTimeField(auto_now_add = True )
def __str__( self ):
return self .title
|
views.py: To show and download files we need to add some function procedures. Add this into filedownloadapp/views.py file.Here, two functions we have created. Cheatsheet function will render all files., and other download_cheatsheet is our downloading buttons function.
Python3
from django.shortcuts import render, HttpResponse
from .models import *
from django.http import FileResponse
from django.shortcuts import get_object_or_404
from .models import Cheatsheet
def download_cheatsheet(request, cheatsheet_id):
cheatsheet = get_object_or_404(Cheatsheet, pk = cheatsheet_id)
file_path = cheatsheet. file .path
response = FileResponse( open (file_path, 'rb' ))
response[ 'Content-Type' ] = 'application/octet-stream'
response[ 'Content-Disposition' ] = f 'attachment; filename="{cheatsheet.title}"'
return response
def cheatsheet(request):
files = Cheatsheet.objects. all ()
print (files)
context = {
'files' : files
}
return render(request, './home.html' , context)
|
settings.py: Below is the required settings to add in Settings.py. and a simple Html template code. The template file will render all uploaded files on page with download button link.
Python3
INSTALLED_APPS = [
"........................."
'filedownloadapp'
]
TEMPLATES = [
{
'BACKEND' : 'django.template.backends.django.DjangoTemplates' ,
'DIRS' : [ 'templates' ],
'APP_DIRS' : True ,
'OPTIONS' : {.......}
]
DATABASES = {
'default' : {
'ENGINE' : 'django.db.backends.sqlite3' ,
'NAME' : BASE_DIR / 'db.sqlite3' ,
}
}
import os
MEDIA_URL = '/media/'
MEDIA_ROOT = os.path.join(BASE_DIR, 'media' )
|
Creating GUI
home.html: The template file will render all uploaded files on page with download button link.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Download Programming Cheatsheets</ title >
</ head >
< style >
.container {
width: 500px;
height: 100%;
margin: 0 auto;
display: flex;
flex-direction: column;
justify-content: center;
}
.card{
width: 100%;
height: auto;
border-radius: 10px;
border: 1px dashed black;
padding: 1rem;
margin-top: 10px;
}
h1{
text-align: center;
font-size: 4em;
}
button{
padding : 5px 5px;
color: green;
}
a{text-decoration:none; color: inherit;}
</ style >
< body >
< div class = "container" >
< h1 >Cheatsheets</ h1 >
{% for file in files %}
< div class = "card" >
< h2 >{{file.title}}</ h2 >
< button > < a href = "{% url 'download_cheatsheet' file.id %}" >Download</ a ></ button >
</ div >
{% endfor %}
</ div >
</ body >
</ html >
|
admin.py: We have defined the model and now register it into admin.py file.
Python3
from django.contrib import admin
from .models import *
admin.site.register(Cheatsheet)
|
Setting up Path
We have 2 folders one of it is base project dload and other is filedownloadapp. to pass the incoming request from the base project folder to app we will need to create urls.
dload/urls.py: Here we defining app URL path.
Python3
from django.contrib import admin
from django.urls import path, include
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' filedownloadapp.urls'))
]
urlpatterns + = static(settings.MEDIA_URL, document_root = settings.MEDIA_ROOT)
|
filedownloadapp/urls.py: Here we defining all the URL paths.
Python3
from django.contrib import admin
from django.urls import path
from . import views
urlpatterns = [
path('', views.cheatsheet, name = "cheatsheet" ),
path( 'download/<int:cheatsheet_id>/' ,
views.download_cheatsheet, name = 'download_cheatsheet' ),
]
|
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
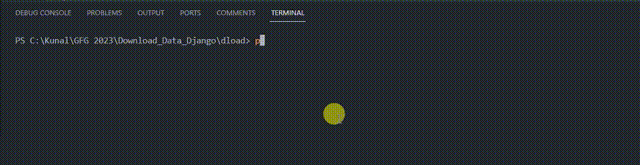
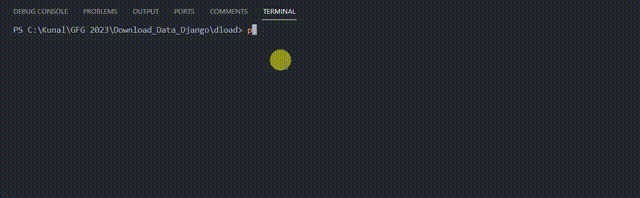
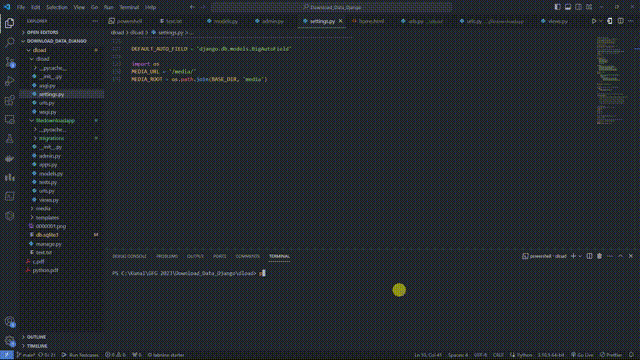
Share your thoughts in the comments
Please Login to comment...