Django Redirects
Last Updated :
25 Sep, 2023
In Django one of the features is the redirect, which allows developers to manage URL redirections within their web applications effortlessly. In this article, we will explore how to use the redirect in Django, covering essential files like views, URLs, and HTML templates.
What is Django redirect?
In Django, the redirect function is a utility that allows you to perform an HTTP redirect. It’s often used in views to redirect users from one URL to another.
Setting up the Project
Let us understand with the help of an example.
Installation
pip3 install django
Step 1: First, make a project with the name ‘project_demo’ by using the command :
Django-admin startproject gfg
Step 2: Create an application named ‘gfg’ by using the command :
python3 manage.py startapp gfg
Now add this app to the ‘INSTALLED_APPS’ in the settings file.
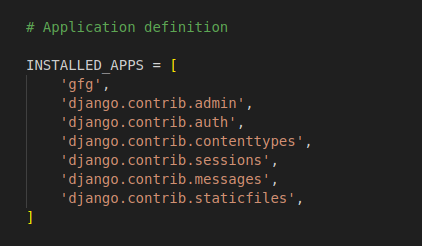
Creating necessary Files
Make all the files as needed:
views.py: This code defines three view functions:
- Here, destination_view is a view function. When a user accesses a URL mapped to this view, it returns an HttpResponse containing the text ‘Home Function is redirected to destination_view function’. This text will be displayed in the user’s browser.
- The home view function is defined here. When a user accesses a URL mapped to this view, it performs a redirection using redirect(‘destination-view’).
- In this code block, a view function called my_redirect_view is defined. It sets target_url to ‘https://geeksforgeeks.org’. This view function uses render to render an HTML template called ‘myapp/index.html’.
Python3
from django.shortcuts import render
from django.http import HttpResponse
from django.shortcuts import redirect, render
def destination_view(request):
return HttpResponse(
'Home Function is redirected to destination_view function' )
def home(request):
return redirect( 'destination-view' )
def my_redirect_view(request):
return render(request, 'myapp/index.html' , { 'target_url' : target_url})
|
index.html: This HTML template provides a user-friendly message for redirection, allowing users to click on a link if the automatic redirection does not happen quickly enough.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Redirection Page</ title >
</ head >
< body >
< h1 >You are being redirected...</ h1 >
< p >If you are not redirected in a few seconds, < a href = "{{ target_url }}" >click here</ a >.</ p >
</ body >
</ html >
|
urls.py: These URL patterns define how incoming requests to specific URLs should be routed to the corresponding view functions within the app.
Python3
from django.urls import path
from . import views
urlpatterns = [
path(' ', views.home, name=' home'),
path( 'my_redirect_view/' , views.my_redirect_view, name = 'my_redirect_view' ),
path( 'destination/' , views.destination_view, name = 'destination-view' ),
]
|
urls.py: In your project’s urls.py, include the URLs from your app.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' gfg.urls')),
]
|
Applying Migrations:
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Deployement of the project:
Run the server with the help of following command:
python3 manage.py runserver
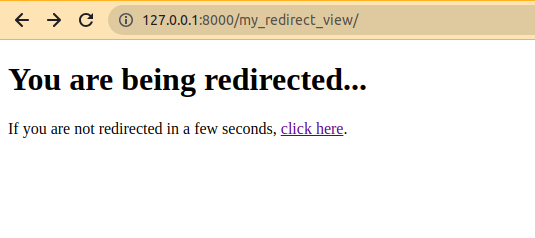
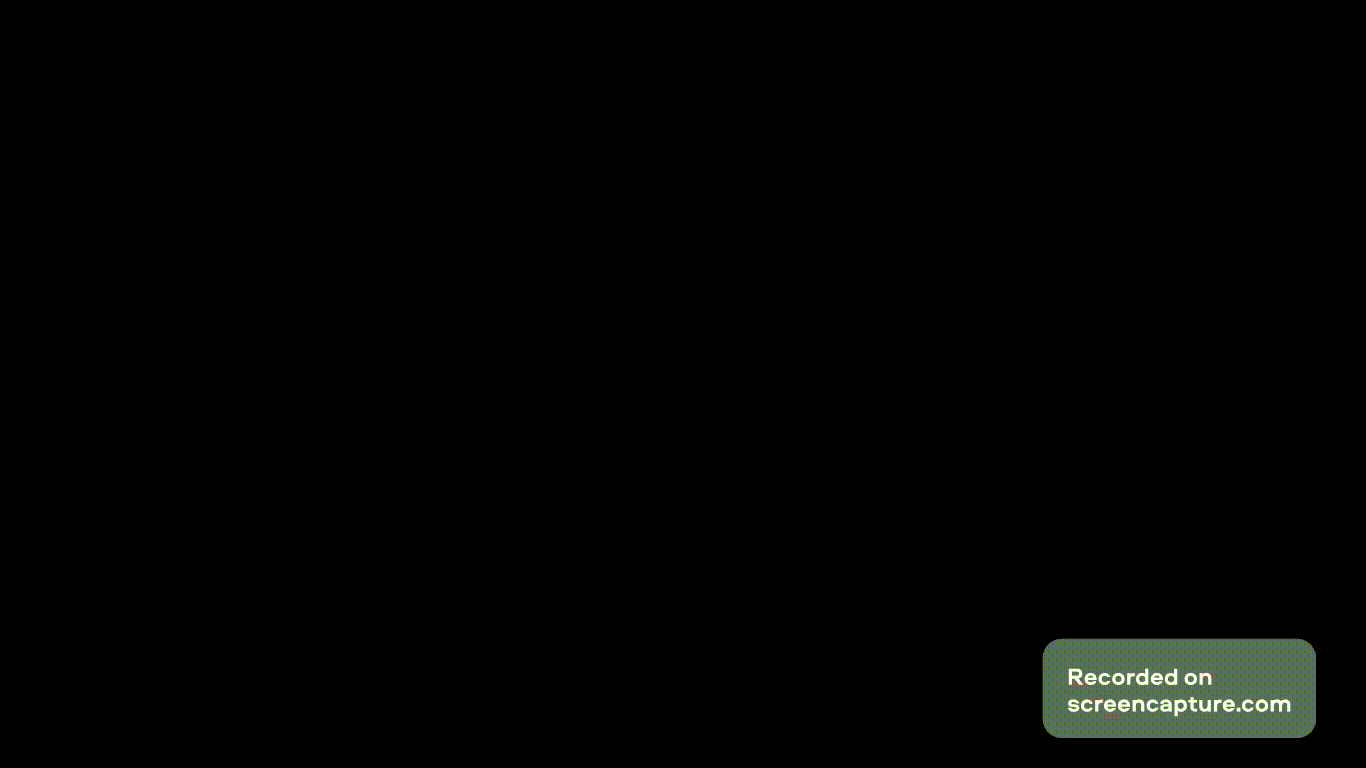
Django Redirects
Share your thoughts in the comments
Please Login to comment...