Django Conditional Expressions in Queries
Last Updated :
02 Oct, 2023
The conditional expressions allow developers to make decisions and manipulate data within queries, templates, and Python code, making it easier to create complex and dynamic web applications. In this article, we’ll delve into the world of conditional expressions in Django and explore how they can be used to enhance your web development projects.
Setting up the Project
Installation: To install Django follow these steps.
Creating the Project
Create the project by using the command
Django-admin startproject projectdemo
cd projectdemo
Create an application named ‘gfg’ by using the command:
python3 manage.py startapp gfg
Now add this app to the ‘INSTALLED_APPS’ in the settings file.
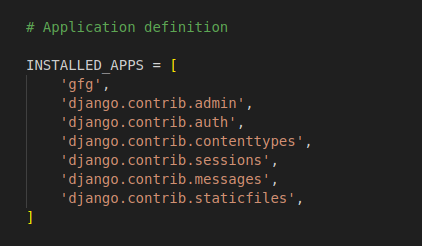
Structuring Our Project
Organize your project directory with a clear file structure.
model.py
The model has three fields: “name” (a character field with a maximum length of 100 characters), “quantity” (an integer field), and “price” (a decimal field with a maximum of 10 digits and 2 decimal places).
Python3
from django.db import models
class Product(models.Model):
name = models.CharField(max_length = 100 )
quantity = models.IntegerField()
price = models.DecimalField(max_digits = 10 , decimal_places = 2 )
def __str__( self ):
return self .name
|
forms.py
This code defines a Django form called ‘ProductForm’ in a file named forms.py within a Django app called ‘products’. The form is used to create and update instances of the Product model.
Python3
from django import forms
from .models import Product
class ProductForm(forms.ModelForm):
class Meta:
model = Product
fields = [ 'name' , 'quantity' , 'price' ]
|
views.py
The code handles product listing and addition. The ‘product_list’ view annotates products to determine their stock status, while the ‘add_product’ view handles the submission of a form to add new products to the database. The HTML templates ‘myapp/index2.html’ and ‘myapp/index.html’ likely contain the presentation and form rendering for these views.
Python3
from django.shortcuts import render
from django.http import HttpResponse
from django.shortcuts import redirect, render
from .forms import ProductForm
def home(request):
return HttpResponse( 'Home Function is redirected to destination_view function' )
from django.shortcuts import render
from django.db.models import Case, When, Value, BooleanField
from .models import Product
def product_list(request):
products = Product.objects.annotate(
in_stock = Case(
When(quantity__gt = 0 , then = Value( True )),
default = Value( False ),
output_field = BooleanField()
)
)
return render(request, 'myapp/index2.html' , { 'products' : products})
def add_product(request):
if request.method = = 'POST' :
form = ProductForm(request.POST)
if form.is_valid():
form.save()
return redirect( 'product_list' )
else :
form = ProductForm()
print ( "hh" )
return render(request, 'myapp/index.html' , { 'form' : form})
|
product_form.html
This HTML code represents a simple web page for adding a product.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Add Product</ title >
</ head >
< body >
< h1 >Add Product</ h1 >
< form method = "post" action = "{% url 'add_product' %}" >
{% csrf_token %}
< label for = "name" >Product Name:</ label >
< input type = "text" id = "name" name = "name" required>< br >< br >
< label for = "quantity" >Quantity:</ label >
< input type = "number" id = "quantity" name = "quantity" required>< br >< br >
< label for = "price" >Price:</ label >
< input type = "number" id = "price" name = "price" step = "0.01" required>< br >< br >
< input type = "submit" value = "Add Product" >
</ form >
</ body >
</ html >
|
product_list.html
This HTML template is used to display a list of products in a Django web application. It iterates through the “products” list, displaying each product’s name, price, and stock status (in stock or out of stock). Additionally, it includes a link for users to add new products to the list.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Product List</ title >
</ head >
< body >
< h1 >Product List</ h1 >
< ul >
{% for product in products %}
< li >
{{ product.name }} -
Price: ${{ product.price }} -
{% if product.in_stock %}
In Stock
{% else %}
Out of Stock
{% endif %}
</ li >
{% endfor %}
</ ul >
</ body >
</ html >
< p >< a href = "{% url 'add_product' %}" >Add a Product</ a ></ p >
|
app/urls.py
This code defines URL patterns for a Django web application in the “pdfapp” module. It uses the Django urls.py file to map URLs to views in the application.
Python3
from django.urls import path
from . import views
urlpatterns = [
path(' ', views.home, name=' home'),
path( 'products/' , views.product_list, name = 'product_list' ),
path( 'add/' , views.add_product, name = 'add_product' ),
]
|
urls.py
This code defines URL patterns for a Django web application of the project.
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' mini.urls')),
]
|
Deployement of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
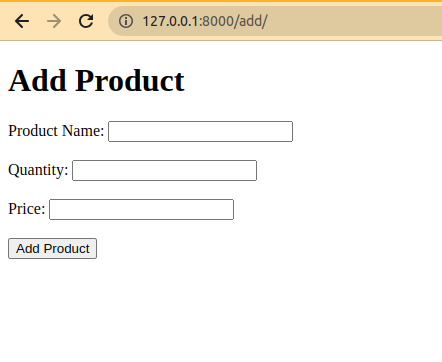
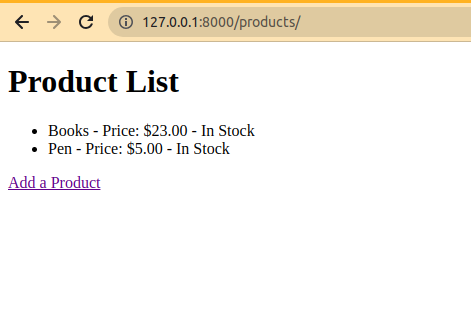
Share your thoughts in the comments
Please Login to comment...