How to log all sql queries in Django?
Last Updated :
13 Oct, 2023
Django, a popular web framework for Python, simplifies the development of database-driven applications. When building Django applications, it’s crucial to monitor and optimize the SQL queries executed by your application for performance and debugging purposes. One effective way to achieve this is by logging all SQL queries. In this article, we will focus on how to log all SQL queries in Django. For doing a log of Django SQL queries we have to follow certain procedures which we are going to discuss here.
What is SQL Query?
SQL is a computer language that is used for storing, manipulating, and retrieving data in a structured format. This language was invented by IBM. Here SQL stands for Structured Query Language. Interacting databases with SQL queries, we can handle a large amount of data. There are several SQL-supported database servers such as MySQL, PostgreSQL, SQLite3, and so on. Data can be stored in a secured and structured format through these database servers. SQL queries are often used for data manipulation and business insights better.
Why to Log SQL Queries?
Logging SQL queries in your Django application serves several important purposes:
- Performance Optimization: By monitoring SQL queries, you can identify and address slow or inefficient database operations, thus improving your application’s performance.
- Debugging: When unexpected behavior occurs, having access to the SQL queries executed can help you pinpoint the issue more effectively.
- Security: Monitoring queries can assist in detecting potential security vulnerabilities such as SQL injection attacks.
Setting up the Project
To understand this concept we have to first create a simple Django-based web application, you can learn from this article https://www.geeksforgeeks.org/getting-started-with-django/. In this article, you will learn what a basic Django application looks like and all its functionality.
Steps to log all SQL Queries in Django
Configure Logging Settings
The first step is to configure Django’s logging settings. This will determine where and how the SQL queries are logged. Open your project’s ‘settings.py’ file and add the following configuration to the LOGGING dictionary:
- Two handlers are defined: ‘console’ for logging to the console and ‘file’ for logging to a file named ‘django_queries.log’. You can customize the filename and path.
- The logger ‘django.db.backends’ is set to log at the ‘DEBUG’ level, which includes SQL queries.
Python3
LOGGING = {
'version' : 1 ,
'disable_existing_loggers' : False ,
'handlers' : {
'console' : {
'level' : 'DEBUG' ,
'class' : 'logging.StreamHandler' ,
},
'file' : {
'level' : 'DEBUG' ,
'class' : 'logging.FileHandler' ,
'filename' : 'django_queries.log' ,
},
},
'loggers' : {
'django.db.backends' : {
'handlers' : [ 'console' , 'file' ],
'level' : 'DEBUG' ,
'propagate' : False ,
},
},
}
|
Enable Database Query Logging
In Django, you can enable query logging selectively, typically in specific views or parts of your code where you want to capture queries. Use the connection object to set the ‘force_debug_cursor’ attribute to ‘True’. By enabling ‘force_debug_cursor’ , Django will log all SQL queries executed during the execution of the associated code.
views.py: For which views we want to print log we just need to add above 2 lines in that views and it will create log for us in file name we mentioned in logging setting in ‘setting.py file’.
Python3
from django.db import connection
def my_view(request):
connection.force_debug_cursor = True
connection.force_debug_cursor = False
|
SQL Queries Log File
Once we have configured the logging settings and enabled query logging where needed, you can view the logs. SQL queries will be logged to both the console and the specified log file.
To view logs in the console, simply run your Django application, and the queries will be displayed there. To access the log file, navigate to the specified path and open the ‘django_queries.log’ file. You can use text editors or command-line tools to view its contents.
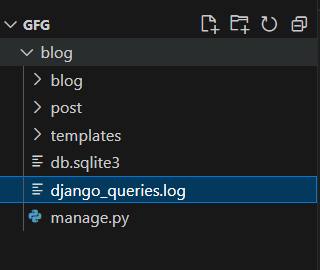
django_queries.log file location
Deployement of Project
Command to apply migration
python3 manage.py makemigrations
python3 manage.py migrate
Command to runserver
python3 manage.py runserver
Output
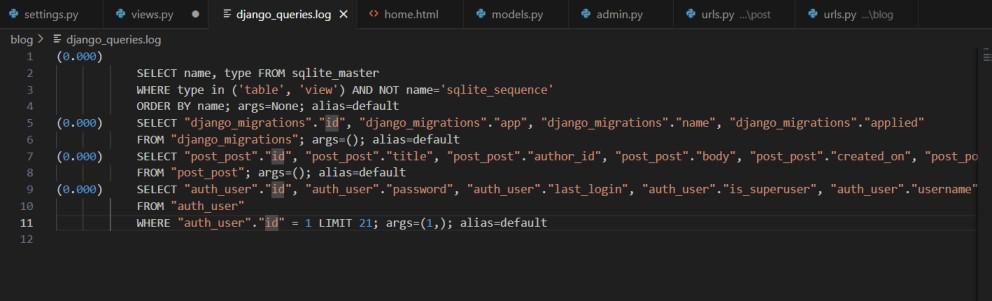
django_queries.log file content
Share your thoughts in the comments
Please Login to comment...