Difference Between the Click & Mousedown Events
Last Updated :
18 Mar, 2024
The mousedown and the click event in JavaScript occur when the mouse button is pressed. But the click event handles the button press as well as the button release while mousedown only handles the button click. Some of the important differences between them are listed below.
Click Event
The click event is triggered when a pointing device button is pressed and released over an element. It is the most used event in JavaScript.
Syntax:
<!-- First way to implement -->
<HTMLElement onclick = "function(){}"></HTMLElement>
<!-- Second way to implement -->
selectedElement.addEventListener('click', callbackFunction(){})
Example: The below code example implements the click event in JavaScript.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Mouse Click Event Example
</title>
<style>
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
}
</style>
</head>
<body>
<button id="myButton">
Click Me
</button>
<script>
const button =
document.getElementById('myButton');
button.addEventListener('click',
function (event) {
console.log('Button clicked!');
alert('Button clicked!');
});
</script>
</body>
</html>
Output:
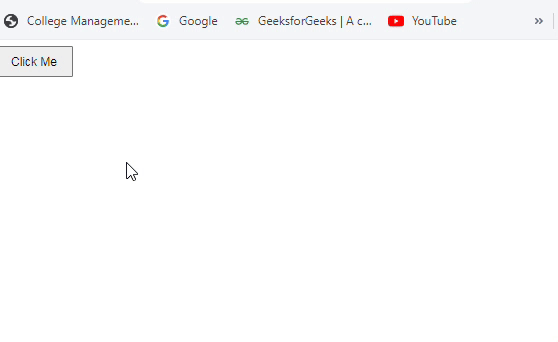
MouseDown Event
The mousedown event is triggered when a pointing device button (such as a mouse button) is initially pressed down over an element. It does not handles release of the button.
Syntax:
<!-- First way to implement -->
<HTMLElement onclick = "function(){}"></HTMLElement>
<!-- Second way to implement -->
selectedElement.addEventListener('click', callbackFunction(){})
Example: The below code example implements the mousedown event in JavaScript .
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Mouse Down Event Example
</title>
<style>
.button {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<button id="myButton" class="button">
Click Me
</button>
<script>
const button =
document.getElementById("myButton");
button.addEventListener("mousedown",
function (event) {
alert
("Mouse button pressed down over the button!");
});
</script>
</body>
</html>
Output:
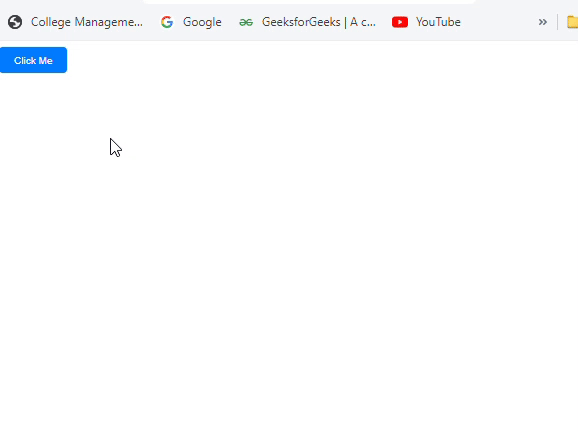
Difference between the click and mousedown events
click event | mousedown event |
---|
This event occurs when a pointing device button(usually a mouse button) is pressed and released quickly over an element.
| This event occurs when a pointing device button is pressed down over an element.
|
It follows the sequence of pressing down and then releasing the mouse button over the element.
| It occurs as soon as the mouse button is pressed down, without waiting for its release.
|
Typically used when you want to trigger an action after the user has confirmed their input, such as clicking a button to submit a form.
| Often used in interactions where you need to detect when the user initiates an action by pressing down the mouse button, such as initiating a drag-and-drop operation or displaying a context menu.
|
It is commonly used for various interactive functionalities such navigating to different pages, toggling UI elements, etc.
| It’s commonly used for actions that require continuous interaction or immediate feedback, such as dragging elements, activating tooltips, creating custom UI controls like sliders, etc.
|
The click event occurs after the mousedown and mouseup events, provided that both actions happen within the same element.
| Unlike the click event, the mousedown event doesn’t require the subsequent release of the mouse button to be triggered.
|
It typically signifies a complete action of clicking on an element, as it requires both pressing and releasing the mouse button.
| It signifies the exact moment when the mouse button is pressed down, regardless of whether it’s released afterward.
|
Share your thoughts in the comments
Please Login to comment...