Difference Between get() and navigate() in Selenium
Last Updated :
10 Mar, 2024
An Automation tool that lets users automate the web page and test it on various browsers is known as Selenium. Selenium helps the users to load the web page through two different functions, i.e., get and navigate. Both of these functions have their advantages as well as disadvantages. In this article, we will compare the get and navigate functions in detail.
Difference Between get() and navigate() in Selenium
The following factors are considered to differentiate between the get and navigate functions in Selenium.
Factors
|
get()
|
navigate()
|
Interface Part
|
The get function is a part of the Webdriver interface.
|
The navigate function is a part of the Navigation interface.
|
Wait for webpage load
|
The get function waits until the webpage is loaded properly and available to return control.
|
The navigate function does not wait for the webpage to be loaded properly.
|
History tracking feature
|
The get function does not have the history tracking feature, thus enabling the user not to go back.
|
The navigate function has a history tracking feature. Thus, the user can go back to the previous web page too.
|
Refresh feature
|
The get function does not support the refresh feature.
|
The navigate function supports the refresh feature.
|
Forward feature
|
The get function does not support the forward function once the user has moved to the earlier webpage.
|
The navigate function supports the forward function and lets the user move to the next page once the user has moved to the earlier webpage.
|
Examples of get() and navigate() in Selenium
Now, we will explain the get() and navigate() functions with examples of both.
1. get() in Selenium
In this example, we have opened the Geeks For Geeks website (link) using the get function.
Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium3 {
public static void main(String[] args) {
System.setProperty( "webdriver.chrome.driver" ,
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
}
}
|
Output:
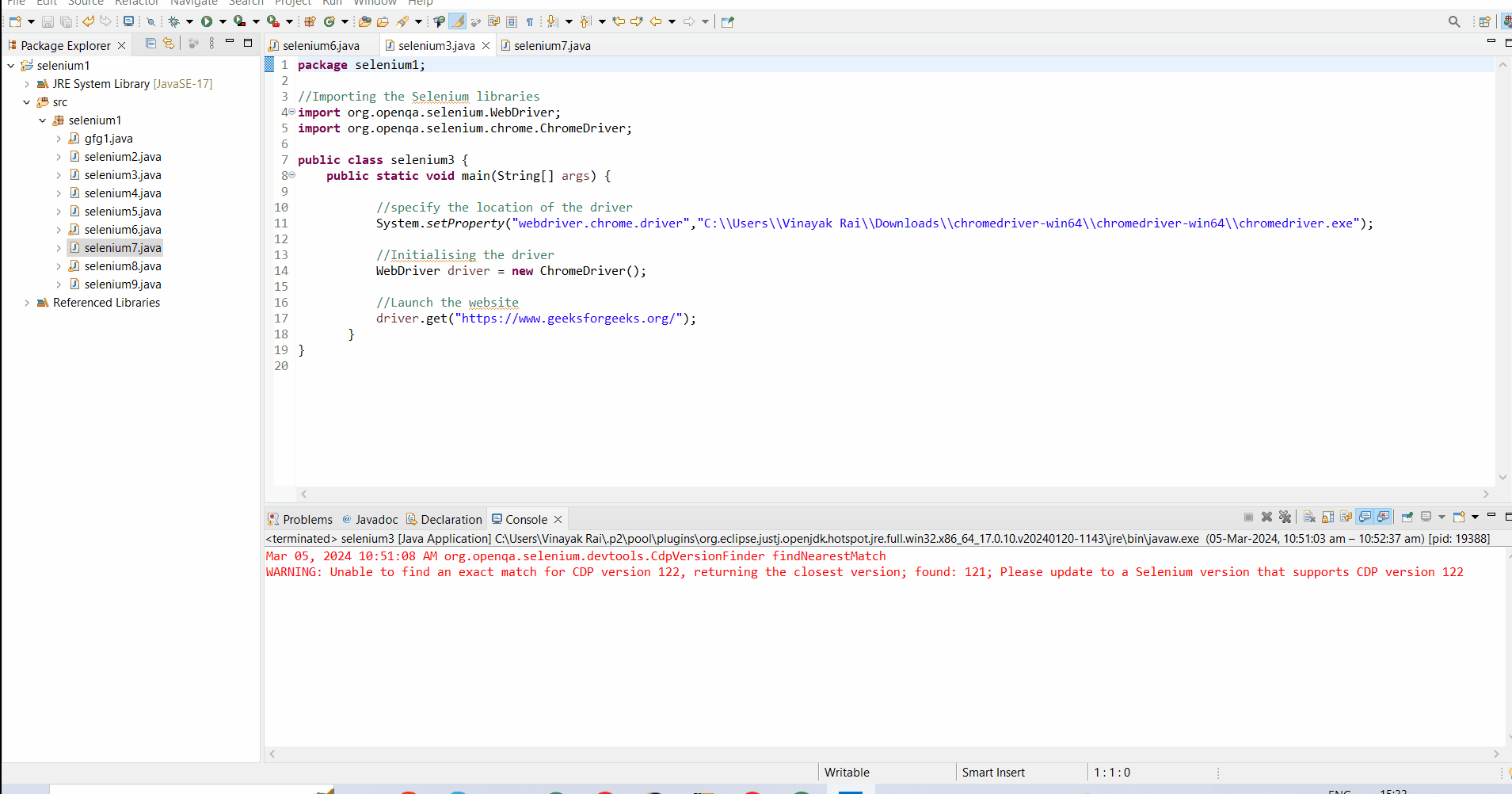
2. navigate() in Selenium
In this example, we have opened the Geeks For Geeks website (link) using navigate().to() function. Then, we have refreshed the browser using navigate().refresh(), click on the element having the text ‘Trending Now’. Moreover, we will now go back to the previous page using navigate().back() function and to next page using navigate().forward() function.
Java
package selenium1;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class selenium6 {
public static void main(String[] args) throws InterruptedException {
System.setProperty( "webdriver.chrome.driver" ,
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win64\\chromedriver-win64\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait( 5 , TimeUnit.SECONDS);
driver.navigate().refresh();
driver.findElement(By.linkText( "Trending Now" )).click();
driver.manage().timeouts().implicitlyWait( 5 , TimeUnit.SECONDS);
driver.navigate().back();
driver.manage().timeouts().implicitlyWait( 5 , TimeUnit.SECONDS);
driver.navigate().forward();
}
}
|
Output:
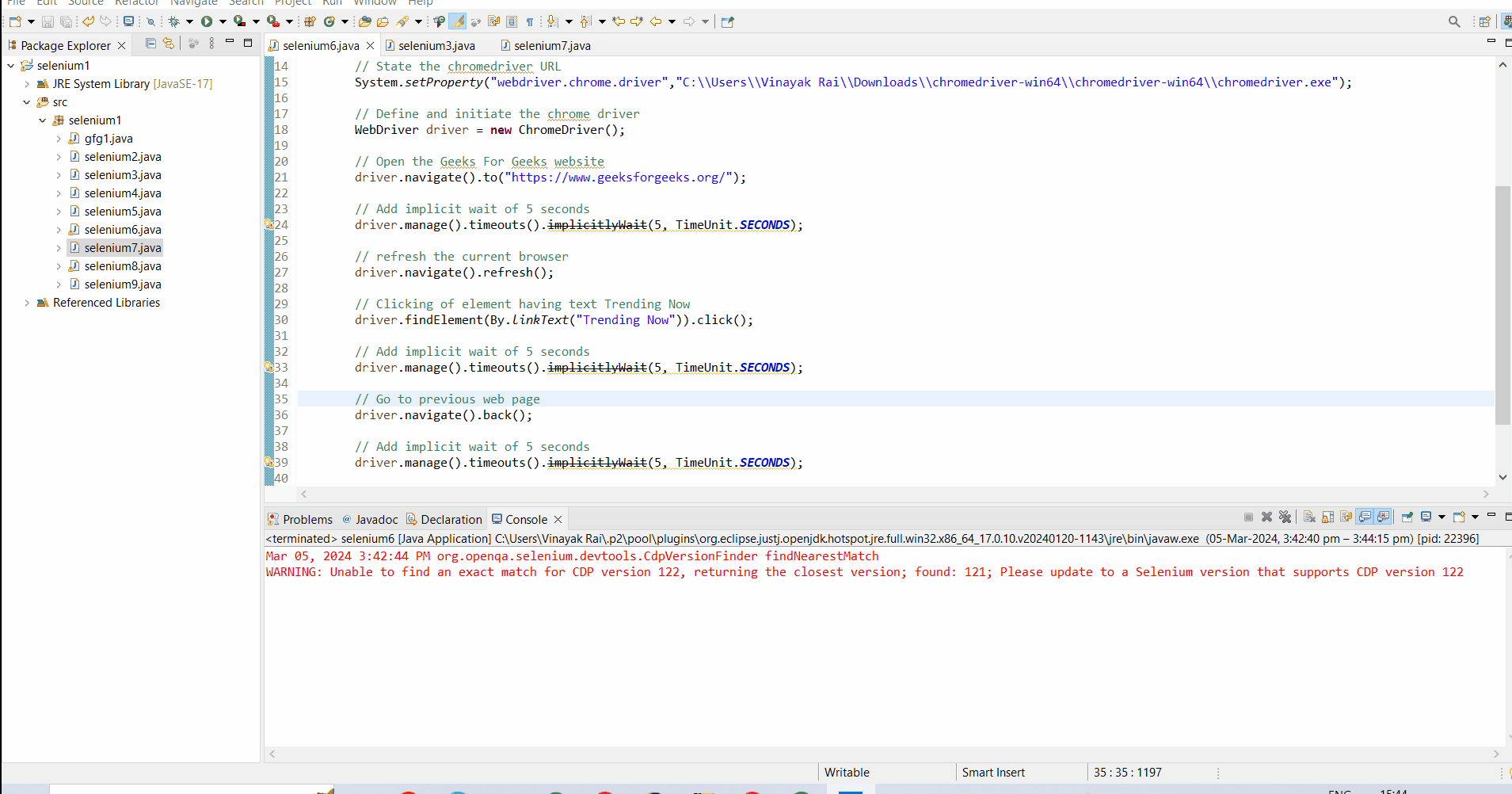
Conclusion
In conclusion, get and navigate functions perform almost the same action. Most commonly, the get() function is used to open a web page as it waits for the web page to load properly. However, you can choose which function to use based on various factors stated in the article.
Share your thoughts in the comments
Please Login to comment...