Difference between getText() and getAttribute() in Selenium WebDriver
Last Updated :
20 Mar, 2024
A collection of libraries that lets the user perform numerous actions on the webpages autonomously is known as Selenium. There are certain ways to get information about an element in Selenium, i.e., getText() and getAttribute(). The getText function is contrary to getAttribute and should be used depending on their appropriate need. In this article, we will compare both of these functions.
Difference between getText() and getAttribute() in Selenium WebDriver
The functions getText and getAttribute can be compared on the various factors.
Factors | getText() | getAttribute() |
---|
Purpose
| The getText() function is used to return the visible text of an element.
| The getAttribute() function is used to return the value of a certain attribute of an element.
|
---|
Visibility Dependency
| The getText() function works only when the specific element is visible on the webpage.
| The getAttribute() function works irrespective of the element is visible or not on the webpage.
|
---|
Obtain hyperlink
| The hyperlink, i.e., href cannot be extracted through this function.
| The hyperlink, i.e., href can be extracted through this function.
|
---|
Interaction Method
| The getText() function interacts with the innerText property of an element.
| The getAttribute function interacts with the attributes of the HTML element.
|
---|
Return Value
| The getText returns the text between opening and closing tags, ignoring leading as well as trailing spaces.
| The getAttribute returns the NULL value in case there is no value specified for an attribute.
|
---|
Examples of getText() and getAttribute()
Now, we will compare both functions with the help of examples.
1. getText() in Selenium
In this example, we have opened the Geeks For Geeks website (link), then we have found an element and obtained the text of the element using getText() function.
Java
//Importing the Selenium libraries
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium3 {
public static void main(String[] args) {
//specify the location of the driver
System.setProperty("webdriver.chrome.driver",
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win32\\chromedriver-win32\\chromedriver.exe");
//Initialising the driver
WebDriver driver = new ChromeDriver();
//Launch the website
driver.get("https://www.geeksforgeeks.org/");
// Find an element
WebElement val=driver.findElement(By.xpath("/html/body/div[2]/div/div/div/div[1]/div[1]/div[1]"));
// Print the text of element
System.out.println(val.getText());
// Close the web driver
driver.quit();
}
}
Output:
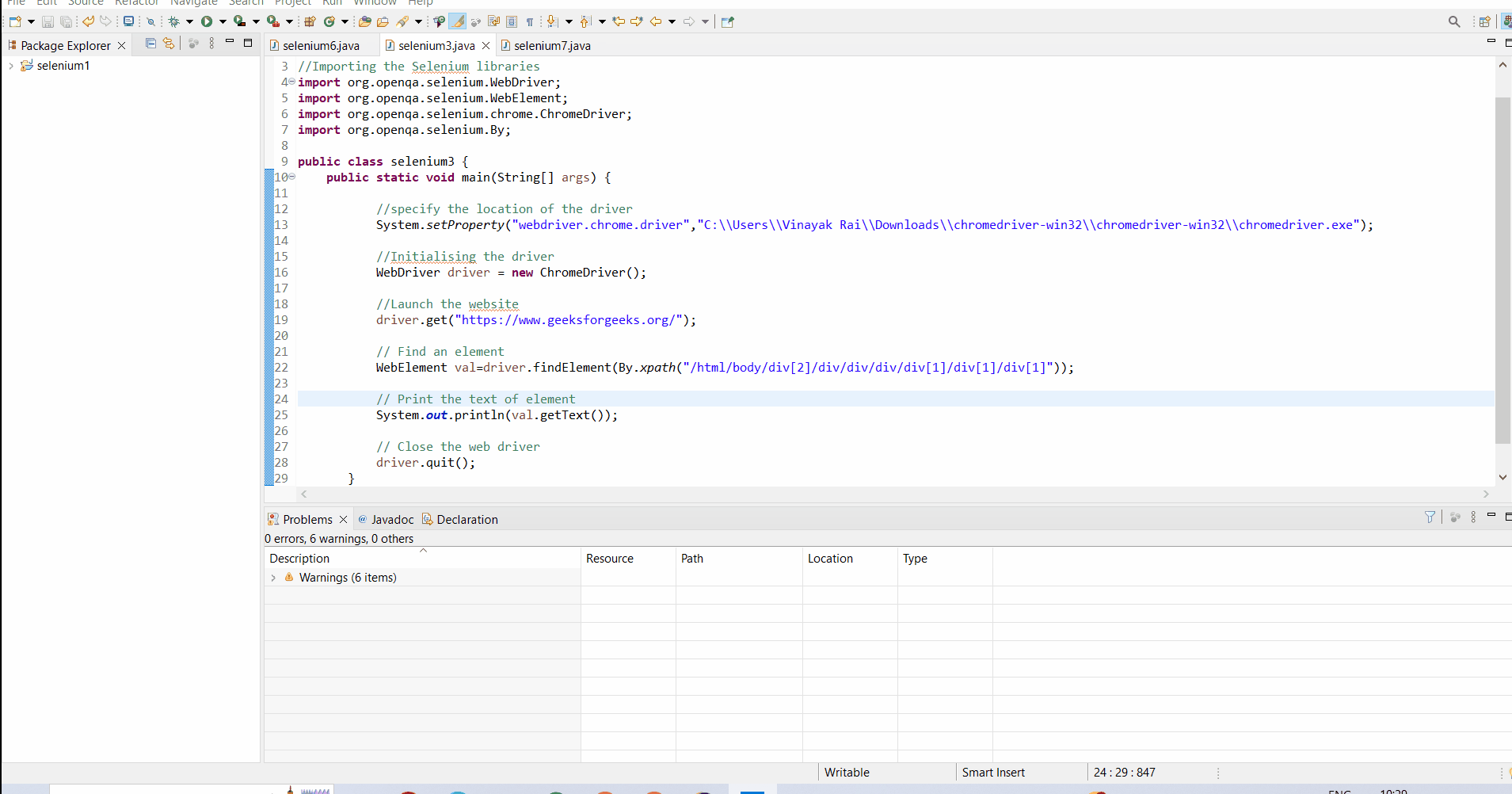
2. getAttribute() in Selenium
In this example, we have opened the Geeks For Geeks website (link), then we have found an element and obtained the class attribute of the specific element using getAttribute() function.
Java
//Importing the Selenium libraries
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.By;
public class selenium3 {
public static void main(String[] args) {
//specify the location of the driver
System.setProperty("webdriver.chrome.driver",
"C:\\Users\\Vinayak Rai\\Downloads\\chromedriver-win32\\chromedriver-win32\\chromedriver.exe");
//Initialising the driver
WebDriver driver = new ChromeDriver();
//Launch the website
driver.get("https://www.geeksforgeeks.org/");
// Find an element
WebElement val=driver.findElement(By.xpath("/html/body/div[2]/div/div/div/div[1]/div[1]/div[1]"));
// Print the class attribute of element
System.out.println(val.getAttribute("class"));
// Close the web driver
driver.quit();
}
}
Output:
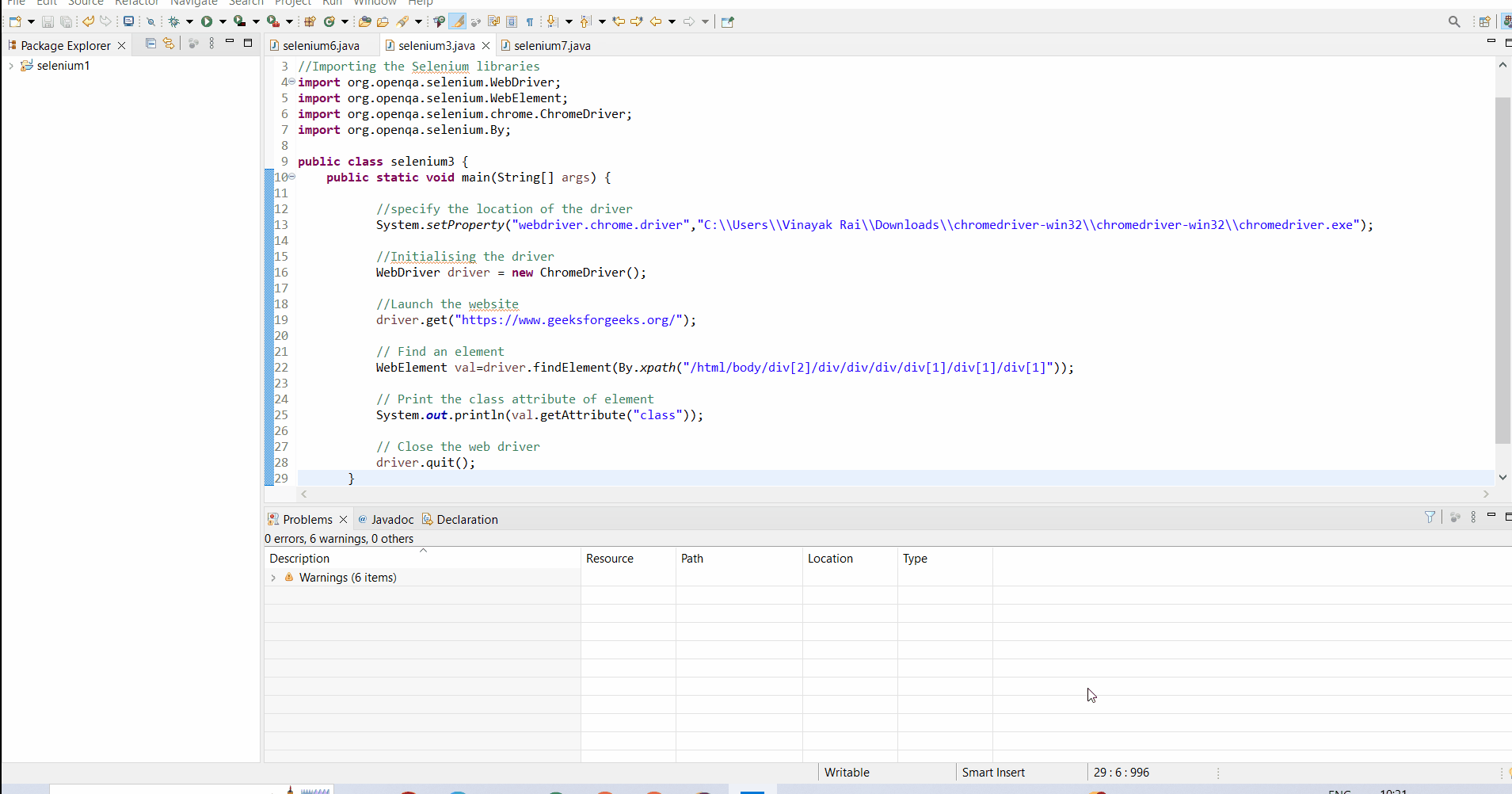
Conclusion
In conclusion, the getText and getAttribute functions have their own significance and should be used by user depending on his requirements. Most commonly, the getText() function is used for obtaining the text of an element. I hope the various factors stated in the article will help you in choosing the appropriate function for you.
Share your thoughts in the comments
Please Login to comment...