Depth First Search or DFS for a Graph
Last Updated :
16 Feb, 2024
Depth First Traversal (or DFS) for a graph is similar to Depth First Traversal of a tree. The only catch here is, that, unlike trees, graphs may contain cycles (a node may be visited twice). To avoid processing a node more than once, use a boolean visited array. A graph can have more than one DFS traversal.
Example:Â
Input: n = 4, e = 6Â
0 -> 1, 0 -> 2, 1 -> 2, 2 -> 0, 2 -> 3, 3 -> 3Â
Output: DFS from vertex 1 : 1 2 0 3Â
Explanation:Â
DFS Diagram:Â
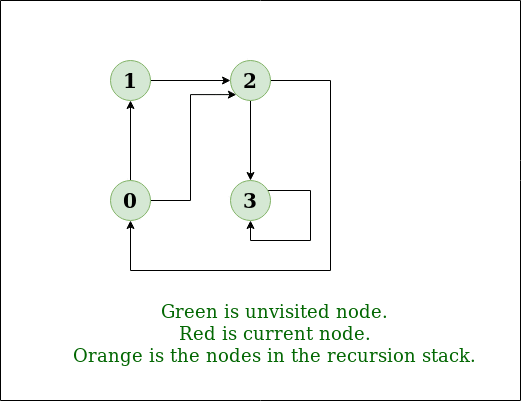
Example 1
Input: n = 4, e = 6Â
2 -> 0, 0 -> 2, 1 -> 2, 0 -> 1, 3 -> 3, 1 -> 3Â
Output: DFS from vertex 2 : 2 0 1 3Â
Explanation:Â
DFS Diagram:Â
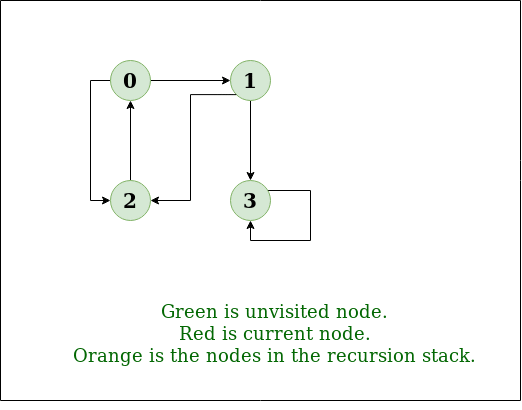
Example 2
How does DFS work?
Depth-first search is an algorithm for traversing or searching tree or graph data structures. The algorithm starts at the root node (selecting some arbitrary node as the root node in the case of a graph) and explores as far as possible along each branch before backtracking.
Let us understand the working of Depth First Search with the help of the following illustration:
Step1: Initially stack and visited arrays are empty.
-copy-660.webp)
Stack and visited arrays are empty initially.
Step 2: Visit 0 and put its adjacent nodes which are not visited yet into the stack.
-copy-660.webp)
 Visit node 0 and put its adjacent nodes (1, 2, 3) into the stack
Step 3: Now, Node 1 at the top of the stack, so visit node 1 and pop it from the stack and put all of its adjacent nodes which are not visited in the stack.
-copy-660.webp)
 Visit node 1
Step 4: Now, Node 2 at the top of the stack, so visit node 2 and pop it from the stack and put all of its adjacent nodes which are not visited (i.e, 3, 4) in the stack.
-copy-660.webp)
 Visit node 2 and put its unvisited adjacent nodes (3, 4) into the stack
Step 5: Now, Node 4 at the top of the stack, so visit node 4 and pop it from the stack and put all of its adjacent nodes which are not visited in the stack.
-copy-660.webp)
 Visit node 4
Step 6: Now, Node 3 at the top of the stack, so visit node 3 and pop it from the stack and put all of its adjacent nodes which are not visited in the stack.
-copy-660.webp)
Visit node 3
Now, Stack becomes empty, which means we have visited all the nodes and our DFS traversal ends.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
class Graph {
public :
map< int , bool > visited;
map< int , list< int > > adj;
void addEdge( int v, int w);
void DFS( int v);
};
void Graph::addEdge( int v, int w)
{
adj[v].push_back(w);
}
void Graph::DFS( int v)
{
visited[v] = true ;
cout << v << " " ;
list< int >::iterator i;
for (i = adj[v].begin(); i != adj[v].end(); ++i)
if (!visited[*i])
DFS(*i);
}
int main()
{
Graph g;
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
cout << "Following is Depth First Traversal"
" (starting from vertex 2) \n" ;
g.DFS(2);
return 0;
}
|
Java
import java.io.*;
import java.util.*;
class Graph {
private int V;
private LinkedList<Integer> adj[];
@SuppressWarnings ( "unchecked" ) Graph( int v)
{
V = v;
adj = new LinkedList[v];
for ( int i = 0 ; i < v; ++i)
adj[i] = new LinkedList();
}
void addEdge( int v, int w)
{
adj[v].add(w);
}
void DFSUtil( int v, boolean visited[])
{
visited[v] = true ;
System.out.print(v + " " );
Iterator<Integer> i = adj[v].listIterator();
while (i.hasNext()) {
int n = i.next();
if (!visited[n])
DFSUtil(n, visited);
}
}
void DFS( int v)
{
boolean visited[] = new boolean [V];
DFSUtil(v, visited);
}
public static void main(String args[])
{
Graph g = new Graph( 4 );
g.addEdge( 0 , 1 );
g.addEdge( 0 , 2 );
g.addEdge( 1 , 2 );
g.addEdge( 2 , 0 );
g.addEdge( 2 , 3 );
g.addEdge( 3 , 3 );
System.out.println(
"Following is Depth First Traversal "
+ "(starting from vertex 2)" );
g.DFS( 2 );
}
}
|
Python3
from collections import defaultdict
class Graph:
def __init__( self ):
self .graph = defaultdict( list )
def addEdge( self , u, v):
self .graph[u].append(v)
def DFSUtil( self , v, visited):
visited.add(v)
print (v, end = ' ' )
for neighbour in self .graph[v]:
if neighbour not in visited:
self .DFSUtil(neighbour, visited)
def DFS( self , v):
visited = set ()
self .DFSUtil(v, visited)
if __name__ = = "__main__" :
g = Graph()
g.addEdge( 0 , 1 )
g.addEdge( 0 , 2 )
g.addEdge( 1 , 2 )
g.addEdge( 2 , 0 )
g.addEdge( 2 , 3 )
g.addEdge( 3 , 3 )
print ( "Following is Depth First Traversal (starting from vertex 2)" )
g.DFS( 2 )
|
C#
using System;
using System.Collections.Generic;
class Graph {
private int V;
private List< int >[] adj;
Graph( int v)
{
V = v;
adj = new List< int >[ v ];
for ( int i = 0; i < v; ++i)
adj[i] = new List< int >();
}
void AddEdge( int v, int w)
{
adj[v].Add(w);
}
void DFSUtil( int v, bool [] visited)
{
visited[v] = true ;
Console.Write(v + " " );
List< int > vList = adj[v];
foreach ( var n in vList)
{
if (!visited[n])
DFSUtil(n, visited);
}
}
void DFS( int v)
{
bool [] visited = new bool [V];
DFSUtil(v, visited);
}
public static void Main(String[] args)
{
Graph g = new Graph(4);
g.AddEdge(0, 1);
g.AddEdge(0, 2);
g.AddEdge(1, 2);
g.AddEdge(2, 0);
g.AddEdge(2, 3);
g.AddEdge(3, 3);
Console.WriteLine(
"Following is Depth First Traversal "
+ "(starting from vertex 2)" );
g.DFS(2);
Console.ReadKey();
}
}
|
Javascript
class Graph
{
constructor(v)
{
this .V = v;
this .adj = new Array(v);
for (let i = 0; i < v; i++)
this .adj[i] = [];
}
addEdge(v, w)
{
this .adj[v].push(w);
}
DFSUtil(v, visited)
{
visited[v] = true ;
console.log(v + " " );
for (let i of this .adj[v].values())
{
let n = i
if (!visited[n])
this .DFSUtil(n, visited);
}
}
DFS(v)
{
let visited = new Array( this .V);
for (let i = 0; i < this .V; i++)
visited[i] = false ;
this .DFSUtil(v, visited);
}
}
g = new Graph(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
console.log( "Following is Depth First Traversal " +
"(starting from vertex 2)" );
g.DFS(2);
|
Output
Following is Depth First Traversal (starting from vertex 2)
2 0 1 3
- Time complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
- Auxiliary Space: O(V + E), since an extra visited array of size V is required, And stack size for iterative call to DFS function.
Share your thoughts in the comments
Please Login to comment...