Deleting all files from a folder using PHP
Last Updated :
08 Jan, 2019
In PHP, files from a folder can be deleted using various approaches and inbuilt methods such as unlink, DirectoryIterator and DirectoryRecursiveIterator.
Some of these approaches are explained below:
Approach 1:
- Generate a list of files using glob() method
- Iterate over the list of files.
- Check whether the name of files is valid.
- Delete the file using unlink() method.
Example:
<?php
$folder_path = "myGeeks" ;
$files = glob ( $folder_path . '/*' );
foreach ( $files as $file ) {
if ( is_file ( $file ))
unlink( $file );
}
?>
|
Output:
Before Running the code:
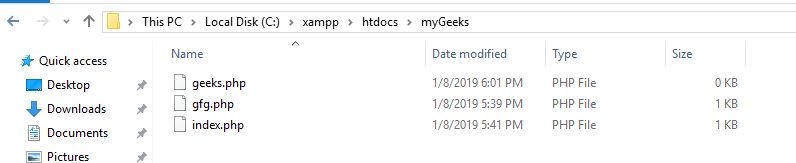
After Running the code:

Note: Hidden files can be included in the file removal operation by addition of the below code:
$hidden_files = glob ( $folder_path . '/{, .}*' , GLOB_BRACE);
|
Approach 2:
Example:
<?php
array_map ( 'unlink' , array_filter (
( array ) array_merge ( glob ( "myGeeks/*" ))));
?>
|
Approach 3:
- Generate list of files using DirectoryIterator.
- Iterate over the list of files.
- Validate the file while checking if the file directory has a dot or not.
- Using the getPathName method reference, delete the file using unlink() method.
Example:
<?php
$folder_path = 'myGeeks/' ;
$dir = new DirectoryIterator(dirname( $folder_path ));
foreach ( $dir as $fileinfo ) {
if (! $fileinfo ->isDot()) {
unlink( $fileinfo ->getPathname());
}
}
?>
|
Approach 4:
Example:
<?php
$dir = "myGeeks/" ;
$dir = new RecursiveDirectoryIterator(
$dir , FilesystemIterator::SKIP_DOTS);
$dir = new RecursiveIteratorIterator(
$dir ,RecursiveIteratorIterator::CHILD_FIRST);
foreach ( $dir as $file ) {
$file ->isDir() ? rmdir ( $file ) : unlink( $file );
}
?>
|
Share your thoughts in the comments
Please Login to comment...