D3.js line() method
Last Updated :
19 Aug, 2020
The d3.line() method is used to constructs a new line generator with the default settings. The line generator is then used to make a line.
Syntax:
d3.line();
Parameters: This method takes no parameters.
Return Value: This method returns a line Generator.
Example 1: Making a simple line using this method.
<!DOCTYPE html>
< html >
< meta charset = "utf-8" >
< head >
< title >Line in D3.js</ title >
</ head >
< script src =
</ script >
< style >
path {
fill: none;
stroke: green;
}
</ style >
< body >
< h1 style="text-align: center;
color: green;">GeeksforGeeks</ h1 >
< center >
< svg width = "500" height = "500" >
< path ></ path >
</ svg >
</ center >
< script >
// Making a line Generator
var Gen = d3.line();
var points = [
[0, 100],
[500, 100]
];
var pathOfLine = Gen(points);
d3.select('path')
.attr('d', pathOfLine);
</ script >
</ body >
</ html >
|
Output:
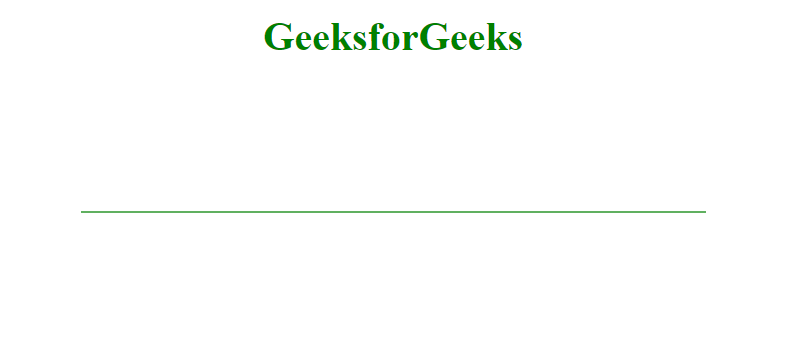
Example 2: Making a Multiconnected line.
<!DOCTYPE html>
< html >
< meta charset = "utf-8" >
< head >
< title >Line in D3.js</ title >
</ head >
< script src =
</ script >
< style >
path {
fill: none;
stroke: green;
}
</ style >
< body >
< h1 style="text-align: center;
color: green;">GeeksforGeeks</ h1 >
< center >
< svg width = "500" height = "500" >
< path ></ path >
</ svg >
</ center >
< script >
// Making a line Generator
var Gen = d3.line();
var points = [
[0, 100],
[500, 100],
[200, 200],
[500, 200]
];
var pathOfLine = Gen(points);
d3.select('path')
.attr('d', pathOfLine);
</ script >
</ body >
</ html >
|
Output:
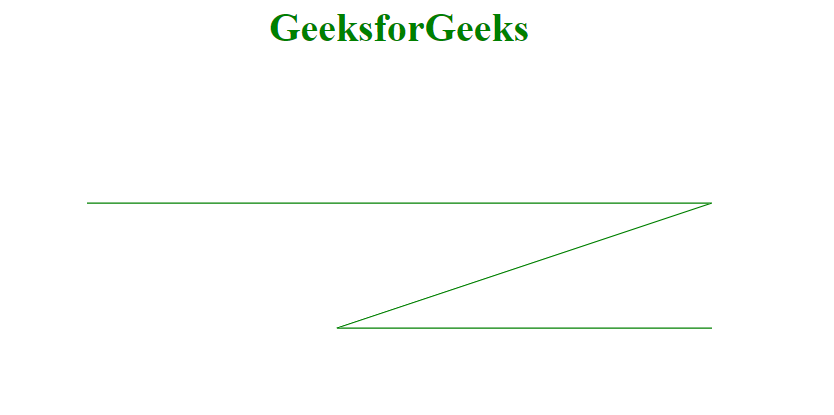
Share your thoughts in the comments
Please Login to comment...