Creating a Travel Journal App using React
Last Updated :
29 Feb, 2024
The Travel Journal App project is a web application developed using React. This travel journal app will allow users to record their travel experiences and manage their entries efficiently. By leveraging the power of React for the frontend and Bootstrap for styling, we’ll create an interactive and visually appealing user interface.
Preview of Final Output: Let us have a look at how the final application will look like:
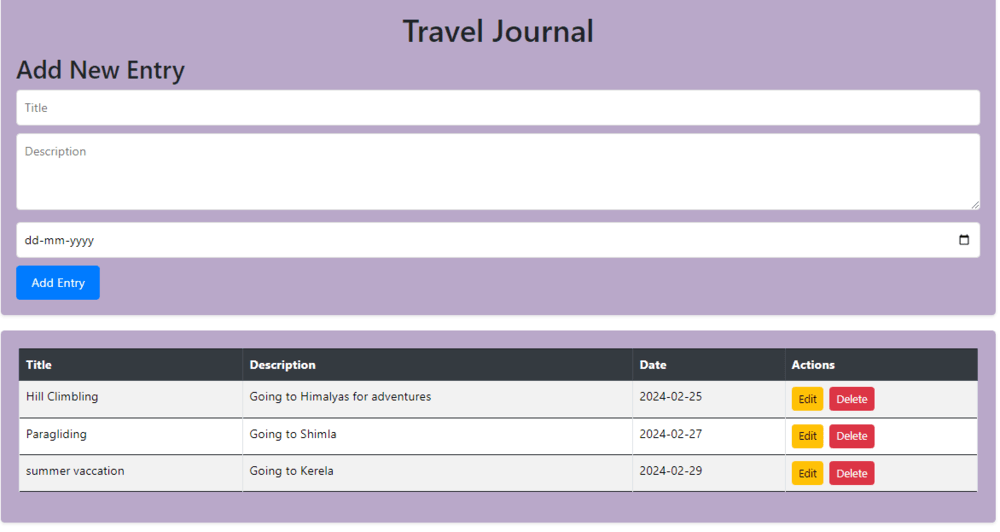
Approach/Functionality of Travel Journal App:
The development approach for our travel journal app involves setting up a React environment, designing components for adding and displaying entries, styling with Bootstrap, managing data using React’s state, implementing user interaction features, testing, and deploying the app for user access. This comprehensive process ensures a seamless and visually appealing experience for recording and managing travel entries.
Steps to Create Travel Journal App using React:
Step 1: Create a new React JS project using the following command.
npx create-react-app <<Project_Name>>
Step 2: Change to the project directory.
cd <<Project_Name>>
Step 3: Install the requires modules.
npm install bootstrap
Step 4: Create a folder called components in src directory and create the following files inside it AddEntry.js, AddList.js etc.
Project Structure:
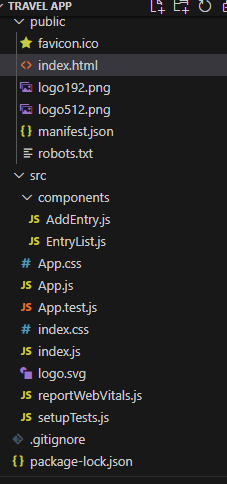
The updated dependencies in package.json will look like this:
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
}
Example: Write the following code in respective files.
- App.js: This component is responsible, for rendering the layout of the application.
- EntryLIst.js: The EntryList component displays a list of travel entries in a table format. Each entry includes details such as title, description, date, and options to edit or delete the entry.
- AddEntry.js: The AddEntry component allows users to add new entries to their travel journal. It consists of input fields for title, description, and date, along with a submit button.
CSS
body {
font-family : Arial , sans-serif ;
background-color : #f4f4f4 ;
}
h 1 {
color : #333 ;
}
.form-container {
margin-bottom : 20px ;
padding : 20px ;
background-color : #b9a8c9 ;
border-radius: 5px ;
box-shadow: 0 2px 4px rgba( 0 , 0 , 0 , 0.1 );
}
form input[type= "text" ],
form textarea,
form input[type= "date" ] {
width : 100% ;
padding : 10px ;
margin-bottom : 10px ;
border : 1px solid #ddd ;
border-radius: 5px ;
font-size : 16px ;
}
form textarea {
height : 100px ;
}
form button {
padding : 10px 20px ;
background-color : #007bff ;
color : #fff ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
font-size : 16px ;
}
form button:hover {
background-color : #0056b3 ;
}
.table-container {
background-color : #b9a8c9 ;
border-radius: 5px ;
box-shadow: 0 2px 4px rgba( 0 , 0 , 0 , 0.1 );
}
.actions button {
padding : 5px 10px ;
margin-right : 5px ;
cursor : pointer ;
border-radius: 5px ;
}
.actions button.edit {
background-color : #ffc107 ;
border : 1px solid #ffc107 ;
}
.actions button.delete {
background-color : #dc3545 ;
border : 1px solid #dc3545 ;
}
.actions button:hover {
opacity: 0.8 ;
}
|
Javascript
import React, { useState } from 'react' ;
import AddEntry from './components/AddEntry' ;
import EntryList from './components/EntryList' ;
import './App.css' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
function App() {
const [entries, setEntries] = useState([]);
const [editIndex, setEditIndex] = useState( null );
const addEntry = (entry) => {
if (editIndex !== null ) {
const newEntries = [...entries];
newEntries[editIndex] = entry;
setEntries(newEntries);
setEditIndex( null );
} else {
setEntries([...entries, entry]);
}
};
const handleDelete = (index) => {
const newEntries = [...entries];
newEntries.splice(index, 1);
setEntries(newEntries);
};
const handleEdit = (index) => {
setEditIndex(index);
};
return (
<div className= "container" >
<div className= "form-container" >
<h1 className= "text-center" >
Travel Journal
</h1>
<AddEntry onAdd={addEntry}
editIndex={editIndex}
entries={entries} />
</div>
<div className= "table-container p-4" >
<EntryList entries={entries}
onDelete={handleDelete}
onEdit={handleEdit} />
</div>
</div>
);
}
export default App;
|
Javascript
import React from 'react' ;
const EntryList = ({ entries, onDelete, onEdit }) => {
return (
<div className= "table-responsive" >
<table className= "table table-striped
table-bordered" >
<thead className= "thead-dark" >
<tr>
<th>Title</th>
<th>Description</th>
<th>Date</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{
entries.map((entry, index) => (
<tr key={index}>
<td>{entry.title}</td>
<td>{entry.description}</td>
<td>{entry.date}</td>
<td>
<button className= "btn btn-sm
btn-warning mr-2"
onClick={
() =>
onEdit(index)
}>
Edit
</button>
<button className= "btn btn-sm btn-danger"
onClick={
() =>
onDelete(index)
}>
Delete
</button>
</td>
</tr>
))
}
</tbody>
</table>
</div>
);
};
export default EntryList;
|
Javascript
import React,
{
useState,
useEffect
} from 'react' ;
const AddEntry = ({ onAdd, editIndex, entries }) => {
const [title, setTitle] = useState( '' );
const [description, setDescription] = useState( '' );
const [date, setDate] = useState( '' );
useEffect(() => {
if (editIndex !== null ) {
const entryToEdit = entries[editIndex];
setTitle(entryToEdit.title);
setDescription(entryToEdit.description);
setDate(entryToEdit.date);
}
}, [editIndex, entries]);
const handleSubmit = (e) => {
e.preventDefault();
if (!title || !description || !date) return ;
onAdd({ title, description, date });
setTitle( '' );
setDescription( '' );
setDate( '' );
};
return (
<div>
<h2>
{
editIndex !== null ?
'Edit Entry' :
'Add New Entry'
}
</h2>
<form onSubmit={handleSubmit}>
<input
type= "text"
placeholder= "Title"
value={title}
onChange={
(e) =>
setTitle(e.target.value)
} />
<textarea
placeholder= "Description"
value={description}
onChange={
(e) =>
setDescription(e.target.value)
}>
</textarea>
<input
type= "date"
value={date}
onChange={
(e) =>
setDate(e.target.value)} />
<button type= "submit" >
{
editIndex !== null ?
'Edit Entry' :
'Add Entry'
}
</button>
</form>
</div>
);
};
export default AddEntry;
|
Step to run the App:
npm start
Output:
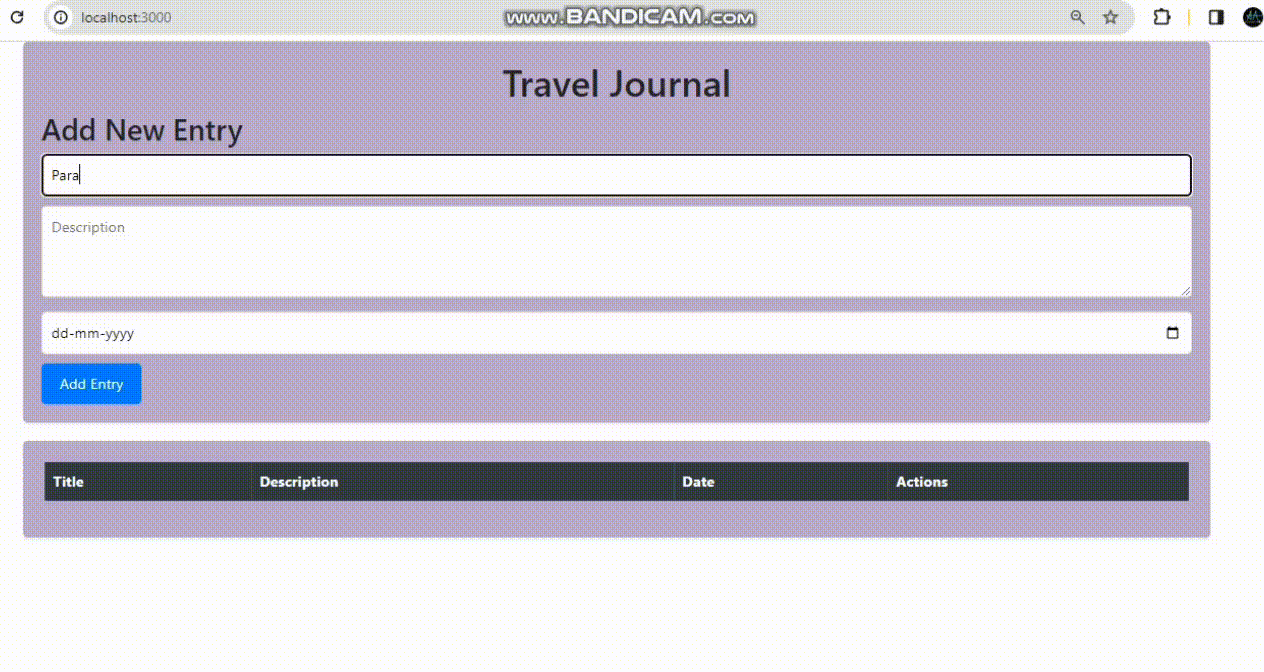
Output
Share your thoughts in the comments
Please Login to comment...