Create a To-Do List App using React Redux
Last Updated :
23 Apr, 2024
A To-Do list allows users to manage their tasks by adding, removing, and toggling the completion status for each added item. It emphasizes a clean architecture, with Redux state management and React interface rendering.
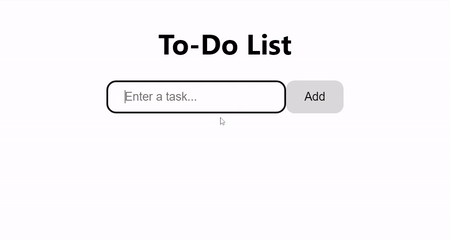
Todo App
Prerequisites
Approach
- Create a new React project using Create React App and install Redux and React Redux to manage application state.
- Create a store for State management. Here, we use createStore to create store and combine all reducers. Define reducer ( adding, removing, and toggling to-do ) here.
- Create a functional component (App.js) that represents the main application and connect the component to the Redux store using connect from React Redux.
- Create a CSS file(App.css) for styling the input field, buttons and to-do list.
Steps to Create Application
Step 1: Create React Application named todo-list-app and navigate to it using this command.
npx create-react-app todo-list-app
cd todo-list-app
Step 2: Install required packages and dependencies.
npm install react-redux redux
Step 3: Check package.json file for updated and installed dependencies, it will look like the below file.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.1",
"redux": "^5.0.1",
},
Step 4: Run the application, start your application by navigating to todo-list-app and use the below command.
npm start
Project Structure:
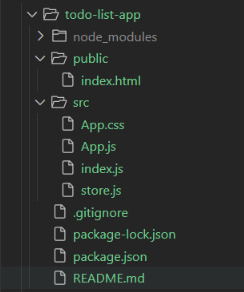
File Structure
Example: The below code will explain the use of react-redux to create todo list in react.
CSS
/* App.css */
#app {
display: flex;
flex-direction: column;
text-align: center;
}
input {
padding: 10px 20px;
border: 1px solid black;
border-radius: 10px;
}
button {
padding: 10px 20px;
border: 1px solid transparent;
border-radius: 10px;
background-color: rgba(204, 204, 204, 0.713);
cursor: pointer;
}
button:hover {
background-color: rgba(7, 162, 7, 0.568);
}
ul li {
list-style: none;
}
.todo button {
margin: 5px;
}
JavaScript
// App.js
import "./App.css";
import React, { useState } from "react";
import { connect } from "react-redux";
const App =
({ todos, addTodo, removeTodo, toggleTodo }) => {
const [text, setText] = useState("");
const handleAddTodo = () => {
if (text.trim() !== "") {
addTodo({
id: new Date().getTime(),
text,
completed: false,
});
setText("");
}
};
const handleRemoveTodo = (id) => {
removeTodo(id);
};
const handleToggleTodo = (id) => {
toggleTodo(id);
};
return (
<div id="app">
<div>
<h1>To-Do List</h1>
<input
type="text"
value={text}
onChange={(e) =>
setText(e.target.value)}
placeholder="Enter a task..."
/>
<button onClick={handleAddTodo}>
Add
</button>
<ul>
{todos.map((todo) => (
<li
className="todo"
key={todo.id}
style={{
textDecoration: todo.completed ?
"line-through" : "none",
color: todo.completed ?
"red" : "black",
}}
onClick={() => handleToggleTodo(todo.id)}
>
{todo.text}
<button onClick=
{() => handleRemoveTodo(todo.id)}>
Remove
</button>
</li>
))}
</ul>
</div>
</div>
);
};
const mapStateToProps = (state) => ({
todos: state.todos,
});
const mapDispatchToProps = (dispatch) => ({
addTodo: (todo) =>
dispatch({
type: "ADD_TODO",
payload: todo
}),
removeTodo: (id) =>
dispatch({
type: "REMOVE_TODO",
payload: id
}),
toggleTodo: (id) =>
dispatch({
type: "TOGGLE_TODO",
payload: id
}),
});
export default connect
(mapStateToProps, mapDispatchToProps)(App);
JavaScript
// index.js
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import store from "./store";
import { Provider } from "react-redux";
const root = ReactDOM.createRoot
(document.getElementById("root"));
root.render(
<Provider store={store}>
<App />
</Provider>
);
JavaScript
// store.js
import { createStore } from "redux";
const initialState = {
todos: [],
};
const rootReducer =
(state = initialState, action) => {
switch (action.type) {
case "ADD_TODO":
return {
...state,
todos: [
...state.todos,
action.payload
],
};
case "REMOVE_TODO":
return {
...state,
todos: state.todos.filter(
(todo) => todo.id !==
action.payload),
};
case "TOGGLE_TODO":
return {
...state,
todos: state.todos.map((todo) =>
todo.id === action.payload
? {
...todo,
completed:
!todo.completed
}
: todo
),
};
default:
return state;
}
};
const store = createStore(rootReducer);
export default store;
Output:
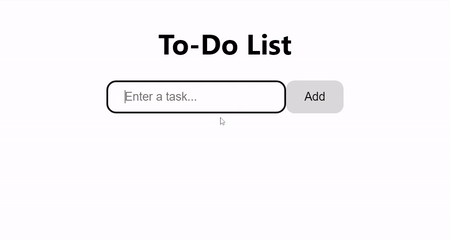
Todo App
Share your thoughts in the comments
Please Login to comment...