Create A Real Estate Website Using React and Tailwind CSS
Last Updated :
12 Mar, 2024
A Real Estate Website built with React JS and Tailwind CSS is a modern and responsive web application that is designed to showcase real estate listings. It typically includes features such as OnSale property, a Features section, and a Contact Us section.
The website is created using React JS, which is a popular JavaScript library used for building user interfaces, and Tailwind CSS, which is a utility-first CSS framework that ensures a responsive and visually appealing design.
Output Preview: Let us have a look at how the final output will look like.
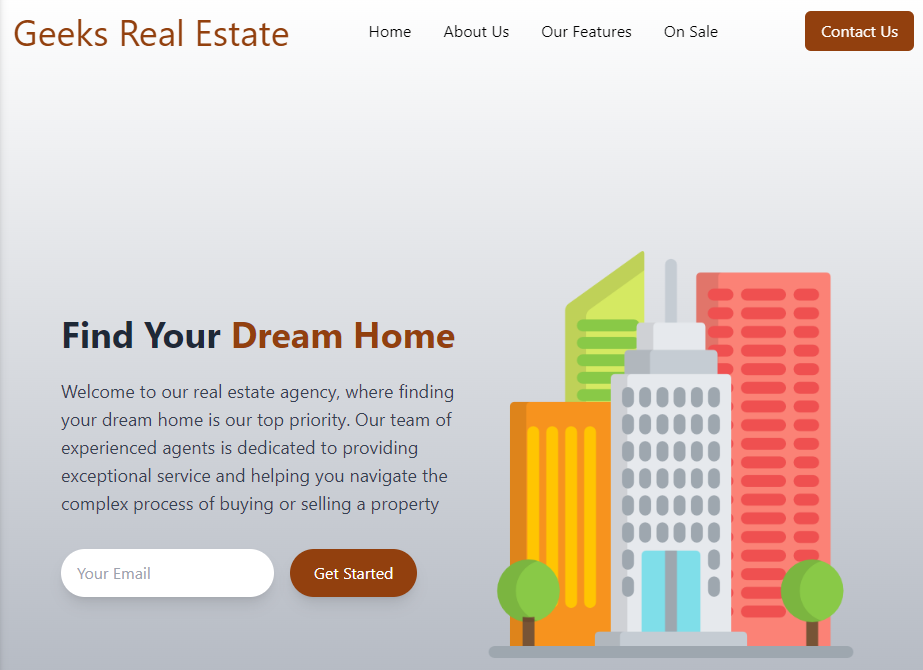
Prerequisites:
Steps to Create React Application & Install required modules:
Step 1: Set up the project using the command
npx create-react-app my-app
Step 2: After creating the application move to the folder using the command
cd my-app
Step 3: Install the required dependencies using the command
npm install -D tailwindcss react-scroll
Step 4: Create the tailwind config file using the command
npx tailwindcss init
Step 5: Rewrite the tailwind.config.js file as folllows
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Project Structure:
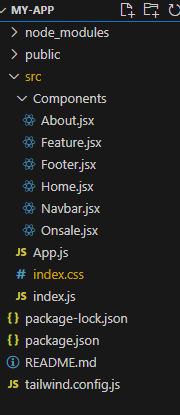
The updated dependencies in package.json file will look like:
"dependencies": {
"@headlessui/react": "^1.7.15",
"@heroicons/react": "^2.0.18",
"@testing-library/jest-dom": "^5.16.5",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"react-scroll": "^1.8.9",
"web-vitals": "^2.1.4"
}
Example: Implementation to design a real estate website.
CSS// index.css
@tailwind base;
@tailwind components;
@tailwind utilities;
@import url('https://fonts.googleapis.com/css2?family=Raleway:wght@300;400;500;600;700;800;900&display=swap');
@layer base {
body {
@apply font-[Raleway];
}
li {
@apply px-4;
@apply cursor-pointer
}
}
Javascript// App.js
import Navbar from "./Components/Navbar"
import Footer from "./Components/Footer";
import Home from "./Components/Home";
import Features from "./Components/Feature";
import Sales from "./Components/Onsale";
import About from "./Components/About";
import "./index.css";
function App() {
return (
<div>
<Navbar />
<Home />
<About />
<Features />
<Sales />
<Footer />
</div>
);
}
export default App;
Javascript// Navbar.js
import React, { useState, useEffect } from "react";
import { Link } from "react-scroll";
import "../index.css";
const Navbar = () => {
const [showMenu, setShowMenu] = useState(false);
const [isScrolled, setIsScrolled] = useState(false);
const handleMenuClick = () => {
setShowMenu(!showMenu);
};
useEffect(() => {
const handleScroll = () => {
if (window.scrollY >= 33) {
setIsScrolled(true);
} else {
setIsScrolled(false);
}
};
window.addEventListener("scroll", handleScroll);
return () => {
window.removeEventListener("scroll", handleScroll);
};
}, []);
return (
<div
className={`fixed top-0 z-50 w-full h-[70px]
flex justify-between items-center px-4
text-black ${isScrolled ? "bg-white" : "bg-transparent"
}`}
>
<div className="text-4xl cursor-pointer
inline-flex items-center text-amber-800">
<Link to="home" smooth={true} duration={500}>
Geeks Real Estate
</Link>
</div>
<div className="md:hidden" onClick={handleMenuClick}>
{showMenu ? (
<svg
className="h-6 w-6 text-black cursor-pointer"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 20 20"
fill="currentColor"
>
<path
fillRule="evenodd"
d="M14.95 5.879l-1.414-1.414L10 8.586 6.464 5.05
5.05 6.464 8.586 10l-3.536 3.536 1.414 1.414L10
11.414l3.536 3.536 1.414-1.414L11.414 10l3.536-3.536z"
clipRule="evenodd"
/>
</svg>
) : (
<svg
className="h-6 w-6 text-black cursor-pointer"
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 20 20"
fill="currentColor"
>
<path
fillRule="evenodd"
d="M3 4a1 1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1zM3
10a1 1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1zM3 16a1
1 0 011-1h12a1 1 0 110 2H4a1 1 0 01-1-1z"
clipRule="evenodd"
/>
</svg>
)}
</div>
<ul className="hidden md:flex">
<li>
<Link to="home" smooth={true} duration={500}>
Home
</Link>
</li>
<li>
<Link to="about" smooth={true} duration={500}>
About Us
</Link>
</li>
<li>
<Link to="feature" smooth={true} duration={500}>
Our Features
</Link>
</li>
<li>
<Link to="sale" smooth={true} duration={500}>
On Sale
</Link>
</li>
</ul>
<div className="hidden md:flex">
<button className="px-4 py-2 bg-amber-800 text-white
rounded-md hover:bg-amber-900
hover:text-white mx-2">
<Link to="contact" smooth={true} duration={500}>
Contact Us
</Link>
</button>
</div>
<div
className={`${showMenu ? "flex" : "hidden"
} md:hidden flex flex-col bg-amber-800 text-white
w-full absolute top-16 left-0 z-10`} >
<Link
to="home"
smooth={true}
duration={500}
className="p-4 hover:bg-gray-700 cursor-pointer"
onClick={handleMenuClick} >
Home
</Link>
<Link
to="about"
smooth={true}
duration={500}
className="p-4 hover:bg-gray-700 cursor-pointer"
onClick={handleMenuClick} >
About Us
</Link>
<Link
to="feature"
smooth={true}
duration={500}
className="p-4 hover:bg-gray-700 cursor-pointer"
onClick={handleMenuClick} >
Our Features
</Link>
<Link
to="sale"
smooth={true}
duration={500}
className="p-4 hover:bg-gray-700 cursor-pointer"
onClick={handleMenuClick} >
On Sale
</Link>
<Link
to="contact"
smooth={true}
duration={500}
className="p-4 hover:bg-gray-700 cursor-pointer"
onClick={handleMenuClick} >
Contact Us
</Link>
</div>
</div>
);
};
export default Navbar;
Javascript// Home.js
import React from "react";
const HomePage = () => {
return (
<div id="home"
className="w-full min-h-screen p-8 flex
items-center bg-gradient-to-b
from-white to-gray-400">
<div className="max-w-7xl mx-auto md:flex
md:flex-row-reverse md:items-center">
<div className="md:w-1/2 md:pr-8 my-11">
<img
src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301104957/skyscraper.png"
alt="Home"
className="w-full h-auto object-cover"
/>
</div>
<div className="md:w-1/2 md:pl-8">
<div className="text-center md:text-left">
<h1 className="text-4xl font-bold text-gray-800 mb-6">
Find Your <span className="text-amber-800">
Dream Home
</span>
</h1>
<p className="text-lg text-gray-700 mb-8">
Welcome to our real estate agency,
where finding your dream home is
our top priority. Our team of experienced
agents is dedicated to providing exceptional
service and helping you navigate the complex
process of buying or selling a property
</p>
<div className="text-center md:text-left">
<form className="flex flex-col md:flex-row gap-4">
<input
id="email"
name="email"
type="email"
autoComplete="email"
placeholder="Your Email"
className="px-4 py-2 bg-white
rounded-full shadow-lg
focus:outline-none"
/>
<button className="px-6 py-3 bg-amber-800
text-white rounded-full
shadow-lg
hover:bg-amber-900">
Get Started
</button>
</form>
</div>
</div>
</div>
</div>
</div>
);
};
export default HomePage;
Javascript// About.js
import React from "react";
const AboutUs = () => {
return (
<div id="about"
className="flex flex-col md:flex-row
w-full h-screen bg-amber-800">
<div className="w-full md:w-1/2 p-0 md:p-8
flex items-center justify-center ">
<img
src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301104749/mansion-(1).png"
alt="About Us"
className="w-full h-auto object-cover"
/>
</div>
<div className="w-full md:w-1/2 p-4 md:p-8
bg-gray-100 flex items-center justify-center">
<div className="text-center md:text-left">
<h2 className="text-3xl md:text-5xl
font-bold text-amber-800 mb-4">
About Us
</h2>
<p className="text-lg md:text-xl text-gray-700 mb-8">
Welcome to my premier real estate agency,
where our mission is to provide exceptional
service and expertise to help our clients
achieve their real estate goals. With years
of experience in the industry, our team of
dedicated professionals has built a
reputation for excellence and integrity.
<br />
<br />
We are committed to staying up-to-date
with the latest trends and technologies
in the real estate market, and we use our
knowledge and expertise to guide our clients
through the buying and selling
process with confidence.
</p>
</div>
</div>
</div>
);
};
export default AboutUs;
Javascript// Feature.js
import React from "react";
const Features = () => {
return (
<div id="feature"
className="w-full min-h-screen p-2 flex
items-center bg-gradient-to-b
from-white to-gray-400">
<div className="max-w-7xl mx-auto py-12
px-4 sm:px-6 lg:py-16 lg:px-8">
<h2 className="text-4xl font-bold text-gray-800 mb-6">Feature
<span className="text-amber-800">Properties</span>
</h2>
<p className="text-lg text-gray-700 mb-8">
Here are some of our featured properties:
</p>
<div className="grid grid-cols-1 sm:grid-cols-2
md:grid-cols-3 gap-8">
<div className='shadow-md shadow-[#040c16]
hover:scale-110 duration-500'>
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301103810/h3.png"
alt="Property"
className="w-full max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium
text-gray-900">
123 Main St</h3>
<p className="text-sm text-gray-500">
3 bd | 2 ba | 1,500 sqft
</p>
<p className="text-lg font-bold
text-gray-700 mt-4">
$500,000
</p>
<button className="mt-6 px-4 py-2 bg-amber-800
text-white rounded-md
hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
<div className='shadow-md shadow-[#040c16]
hover:scale-110 duration-500'>
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301103810/h2.png"
alt="Property"
className="w-full max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium
text-gray-900">
456 Oak St
</h3>
<p className="text-sm text-gray-500">
4 bd | 3 ba | 2,000 sqft
</p>
<p className="text-lg font-bold
text-gray-700 mt-4">
$750,000
</p>
<button className="mt-6 px-4 py-2
bg-amber-800 text-white
rounded-md
hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
<div className='shadow-md shadow-[#040c16]
hover:scale-110 duration-500'>
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301103811/h1.png"
alt="Property"
className="max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium
text-gray-900">
789 Maple Ave
</h3>
<p className="text-sm text-gray-500">
2 bd | 1 ba | 1,000 sqft
</p>
<p className="text-lg font-bold
text-gray-700 mt-4">
$300,000
</p>
<button className="mt-6 px-4 py-2
bg-amber-800 text-white
rounded-md
hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
</div>
</div>
</div>
);
};
export default Features;
Javascript// Onsale.js
import React from "react";
const SaleProperties = () => {
return (
<div id="sale"
className="w-full min-h-screen p-2
flex items-center bg-gray-100">
<div className="max-w-7xl mx-auto py-12
px-4 sm:px-6 lg:py-16 lg:px-8">
<h2 className="text-4xl font-bold text-gray-800 mb-6">
On Sale <span className="text-amber-800">Properties</span>
</h2>
<p className="text-lg text-gray-700 mb-8">
We offer a wide selection of on-sale
properties that cater to different
preferences and budgets. Here are
some of our featured properties:
</p>
<div className="grid grid-cols-1 sm:grid-cols-2
md:grid-cols-3 gap-8">
<div className="hover:shadow-md hover:shadow-[#040c16]">
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301104543/house-(1).png"
alt="Property"
className="w-full max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium text-gray-900">
123 Main St
</h3>
<p className="text-sm text-gray-500">
3 bd | 2 ba | 1,500 sqft
</p>
<p class="text-lg font-bold text-gray-700 mt-4">
<del>$500,000</del> On Sale Now: $250,000
</p>
<button className="mt-6 px-4 py-2 bg-amber-800
text-white rounded-md hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
<div className="hover:shadow-md hover:shadow-[#040c16]">
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301104545/mansion.png" alt="Property"
className="w-full max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium
text-gray-900">456 Oak St</h3>
<p className="text-sm text-gray-500">
4 bd | 3 ba | 2,000 sqft
</p>
<p class="text-lg font-bold text-gray-700 mt-4">
<del>$750,000</del> On Sale Now: $500,000
</p>
<button className="mt-6 px-4 py-2 bg-amber-800
text-white rounded-md hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
<div className="hover:shadow-md hover:shadow-[#040c16]">
<div className="bg-white rounded-lg shadow-lg">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240301104546/house.png" alt="Property"
className="w-full max-h-64 object-cover
rounded-t-lg h-1/3 md:h-64" />
<div className="py-6 px-4">
<h3 className="text-lg font-medium
text-gray-900">789 Maple Ave</h3>
<p className="text-sm text-gray-500">
2 bd | 1 ba | 1,000 sqft
</p>
<p class="text-lg font-bold text-gray-700 mt-4">
<del>$300,000</del> On Sale Now: $200,000
</p>
<button className="mt-6 px-4 py-2 bg-amber-800
text-white rounded-md hover:bg-amber-700">
View Details
</button>
</div>
</div>
</div>
</div>
</div>
</div>
);
};
export default SaleProperties;
Javascript// Footer.js
import React from "react";
import { Link } from 'react-scroll';
const Footer = () => {
return (
<footer className="bg-amber-800">
<div className="max-w-7xl mx-auto
py-8 px-4 sm:px-6 lg:px-8">
<nav className="-mx-5 -my-2 flex flex-wrap
justify-center" aria-label="Footer">
<div className="px-5 py-2">
<div className="text-xl text-white font-bold
hover:text-gray-300 cursor-pointer">
Geeks Real Estate
</div>
</div>
<div className="px-5 py-2 cursor-pointer">
<Link to="home"
className="text-white hover:text-gray-300">
Home
</Link>
</div>
<div className="px-5 py-2 cursor-pointer">
<Link to="feature"
className="text-white hover:text-gray-300">
Features
</Link>
</div>
<div className="px-5 py-2 cursor-pointer">
<Link to="client"
className="text-white hover:text-gray-300">
Clients
</Link>
</div>
<div className="px-5 py-2 cursor-pointer">
<Link to="about"
className="text-white hover:text-gray-300">
About Us
</Link>
</div>
<div className="px-5 py-2 cursor-pointer">
<Link to="contact"
className="text-white hover:text-gray-300">
Contact Us
</Link>
</div>
</nav>
<div className="grid grid-cols-1 md:grid-cols-3 gap-8 mt-8">
<div className="col-span-1">
<h3 className="text-white text-lg font-medium mb-4">
About Us
</h3>
<p className="text-white mb-4">
Real Estate Company is a leading
provider of real estate services,
with a focus on delivering exceptional
value to our clients. Our team of experts
has deep industry knowledge and a proven
track record of success.
</p>
</div>
<div className="col-span-1">
<h3 className="text-white text-lg font-medium mb-4">
Services
</h3>
<ul className="text-white">
<li className="mb-2">Residential Real Estate</li>
<li className="mb-2">Commercial Real Estate</li>
<li className="mb-2">Property Management</li>
<li className="mb-2">Real Estate Investing</li>
</ul>
</div>
<div className="col-span-1">
<h3 className="text-white text-lg font-medium mb-4">
Social links
</h3>
<ul className="text-white">
<li className="mb-2">Facebook</li>
<li className="mb-2">Instagram</li>
<li className="mb-2">LinkedIn</li>
</ul>
</div>
</div>
<div className="mt-8 flex justify-center">
<p className="text-base text-white">
© {new Date().getFullYear()}
Geeks Real Estate. All rights reserved.
</p>
</div>
</div>
</footer>
);
};
export default Footer;
Steps to run the application:
Step 1:Â Type the following command in terminal
npm run start
Output:
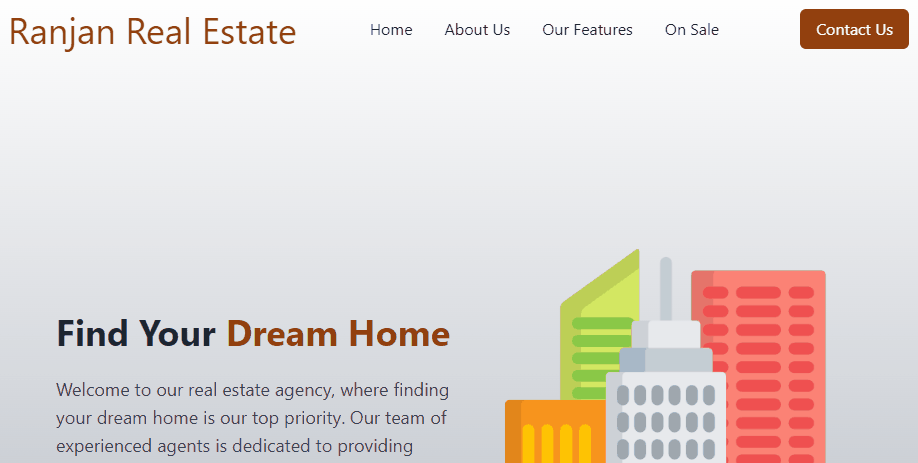
Share your thoughts in the comments
Please Login to comment...