C++ Program to Read and Print All Files From a Zip File
Last Updated :
09 Oct, 2023
In this article, we will explore how to create a C++ program to read and print all files contained within a zip archive.
What are zip files?
Zip files are a popular way to compress and store multiple files in a single container. They use an algorithm to decrease the size of the data they store. They also contain information about file type, name, signature, and compression method.
C++ language doesn’t have built-in support for zip files but there are some third-party libraries that allow the programmer to perform operations on zip files. One such library is libzip which we are going to use in our program.
Prerequisites: Basic C++, libzip installed on the system.
Approach
We will follow these steps to read and print all files from a zip file:
1. Include Necessary Headers and Open Zip Archive
- In this step, we include the neccesary header files:
- After that, we create a zip pointer and open the zip archive sample.zip using zip_open() function.
- Finally, we check if the zip archive is successfully opened or not.
2. Iterate through Each File and Print its Content
- After the zip archive is opened, we first get the number of files inside the archive using zip_get_num_files()
- Then we start parsing each file.
- For each file,
- We obtain its info using zip_stat_info() function,
- Print the filename.
- Open the file for reading using zip_fopen_index().
- Read the file using zip_fread() and printi ts content.
- Close the file using zip_fclose().
- We will repeat the steps till no file is left.
- After that, we will close the archive too using zip_close().
C++ Program to Read and Print Files from a Zip Archive
Zip File Content
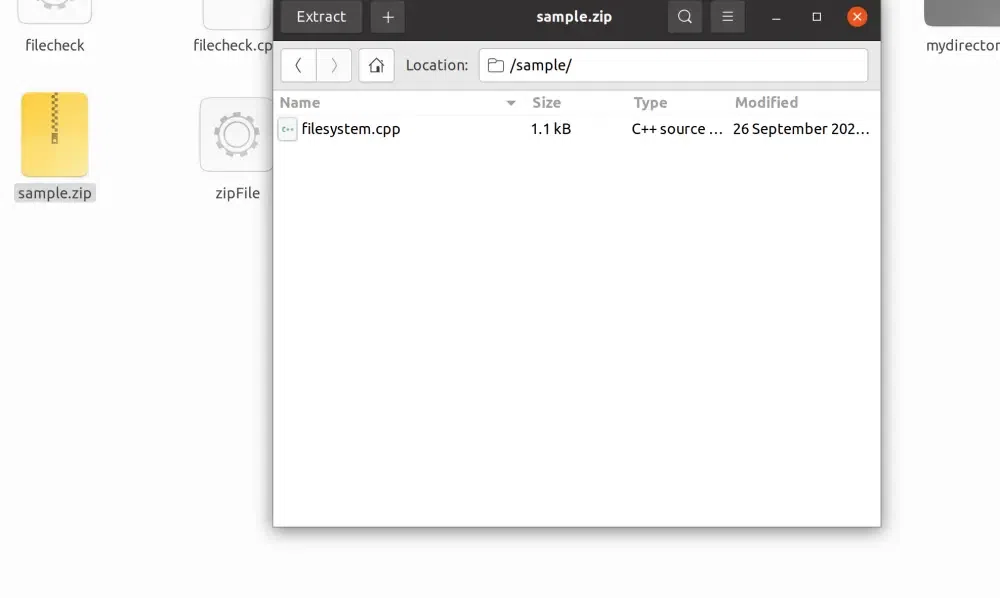
Zip File Contents
C++
#include <iostream>
#include <zip.h>
int main()
{
const char * zipFileName = "sample.zip" ;
zip* archive = zip_open(zipFileName, 0, NULL);
if (!archive) {
std::cerr << "Failed to open the zip file."
<< std::endl;
return 1;
}
int numFiles = zip_get_num_files(archive);
for ( int i = 0; i < numFiles; ++i) {
struct zip_stat fileInfo;
zip_stat_init(&fileInfo);
if (zip_stat_index(archive, i, 0, &fileInfo) == 0) {
std::cout << "File Name: " << fileInfo.name
<< std::endl;
zip_file* file = zip_fopen_index(archive, i, 0);
if (file) {
char buffer[1024];
while (
zip_fread(file, buffer, sizeof (buffer))
> 0) {
std::cout.write(
buffer, zip_fread(file, buffer,
sizeof (buffer)));
}
zip_fclose(file);
}
}
}
zip_close(archive);
return 0;
}
|
Output
Compliexity Analysis
Time Complexity
For both approaches, the time complexity is mainly determined by the number of files in the zip archive and the size of the largest file. Therefore, the time complexity is O(n * file_size), where ‘n’ is the number of files, and ‘file_size’ is the size of the largest file. This is because the program needs to loop through each file and read/print its contents.
Space Complexity
The space complexity primarily depends on the size of the largest file being read and printed at any given time. The space complexity can be considered O(file_size) for the buffer used to read and print file contents.
Share your thoughts in the comments
Please Login to comment...