How to Read a File Using ifstream in C++?
Last Updated :
06 Feb, 2024
In C++, we can read the contents of the file by using the ifstream object to that file. ifstream stands for input file stream which is a class that provides the facility to create an input from to some particular file. In this article, we will learn how to read a file line by line through the ifstream class in C++.
Read File Using ifstream in C++
To read a file line by line using the ifstream class, we can use the std::getline() function. The getline() function can take input from any available stream so we can use the ifstream to the file with the getline() function.
Algorithm
- We will first create an ifstream object associated with the given file.
- We then use the getline() function and pass the previously created ifstream object to it along with the buffer.
In the following example, we will read a text file abc.txt line by line using the getline function of the input stream.
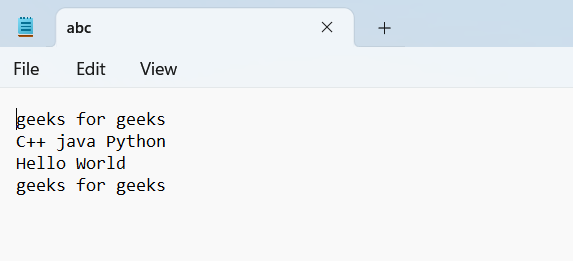
abc.txt
C++ Program to Read File using ifstream in C++
C++
#include <fstream>
#include <iostream>
#include <string>
using namespace std;
int main()
{
ifstream inputFile( "abc.txt" );
string line;
while (getline(inputFile, line)) {
cout << line << endl;
}
inputFile.close();
return 0;
}
|
Output

Output after reading the text file abc.txt
Time Complexity: O(N), where N is the number of characters in the file
Auxilary space: O(M), where M is the length of the longest line in the file.
Share your thoughts in the comments
Please Login to comment...