Count the number of Nodes in a Binary Tree in Constant Space
Last Updated :
29 Nov, 2023
Given a binary tree having N nodes, count the number of nodes using constant O(1) space. This can be done by simple traversals like- Preorder, InOrder, PostOrder, and LevelOrder but these traversals require an extra space which is equal to the height of the tree.
Examples:
Input:
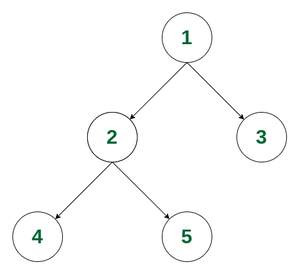
Output: 5
Explanation: In the above binary tree, there are total 5 nodes: 1, 2, 3, 4 and 5
Input:
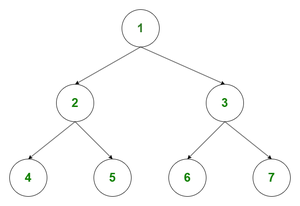
Output: 7
Explanation: In the above binary tree, there are total 7 nodes: 1, 2, 3, 4, 5, 6 and 7
Approach: To solve the problem follow the below idea:
We can solve this problem using the Morris Traversal—a Threaded Binary Tree traversal technique that enables us traverse all the nodes in any binary tree in constant space. Morris traversal does not rely on any additional data structure. The process operates by altering the arrangement of the tree as we navigate through it and subsequently restoring it back to the original state.
Steps to count nodes in a tree using Morris Traversal:
- Start by initializing two variables, cnt as 0 to keep track of the number of nodes counted and current as root to traverse the array.
- Repeat the following steps until we have traversed the tree:
- If the current node does not have a left child increase the count. Move to its right child.
- If the current node has a left child find its inorder predecessor (the rightmost node, in its subtree).
- If the predecessors right child is null set it to be the node and move to its child.
- If the predecessors right child is already set as the node (indicating that we have visited its subtree) reset it to null increment the count and move to its right child.
- Finally return the count variable which will contain the number of nodes in this tree.
Below is the implementation for the above approach:
C++
#include <iostream>
class TreeNode {
public :
int val;
TreeNode* left;
TreeNode* right;
TreeNode( int value)
{
val = value;
left = nullptr;
right = nullptr;
}
};
int countNodes(TreeNode* root)
{
int count = 0;
TreeNode* current = root;
while (current != nullptr) {
if (current->left == nullptr) {
count++;
current = current->right;
}
else {
TreeNode* predecessor = current->left;
while (predecessor->right != nullptr
&& predecessor->right != current) {
predecessor = predecessor->right;
}
if (predecessor->right == nullptr) {
predecessor->right = current;
current = current->left;
}
else {
predecessor->right = nullptr;
count++;
current = current->right;
}
}
}
return count;
}
int main()
{
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
int nodeCount = countNodes(root);
std::cout << "Number of nodes in the binary tree: "
<< nodeCount << std::endl;
return 0;
}
|
Java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode( int value) {
val = value;
}
}
public class CountNodesInBinaryTree {
public static int countNodes(TreeNode root) {
int count = 0 ;
TreeNode current = root;
while (current != null ) {
if (current.left == null ) {
count++;
current = current.right;
} else {
TreeNode predecessor = current.left;
while (predecessor.right != null && predecessor.right != current) {
predecessor = predecessor.right;
}
if (predecessor.right == null ) {
predecessor.right = current;
current = current.left;
} else {
predecessor.right = null ;
count++;
current = current.right;
}
}
}
return count;
}
public static void main(String[] args) {
TreeNode root = new TreeNode( 1 );
root.left = new TreeNode( 2 );
root.right = new TreeNode( 3 );
root.left.left = new TreeNode( 4 );
root.left.right = new TreeNode( 5 );
int nodeCount = countNodes(root);
System.out.println( "Number of nodes in the binary tree: " + nodeCount);
}
}
|
Python
class TreeNode:
def __init__( self , value):
self .val = value
self .left = None
self .right = None
def countNodes(root):
cnt = 0
current = root
while current is not None :
if current.left is None :
cnt + = 1
current = current.right
else :
pre = current.left
while pre.right is not None and pre.right ! = current:
pre = pre.right
if pre.right is None :
pre.right = current
current = current.left
else :
pre.right = None
cnt + = 1
current = current.right
return cnt
root = TreeNode( 1 )
root.left = TreeNode( 2 )
root.right = TreeNode( 3 )
root.left.left = TreeNode( 4 )
root.left.right = TreeNode( 5 )
nodeCount = countNodes(root)
print ( 'Number of nodes in the binary tree:' , nodeCount)
|
C#
using System;
public class TreeNode
{
public int val;
public TreeNode left;
public TreeNode right;
public TreeNode( int value)
{
val = value;
left = null ;
right = null ;
}
}
public class BinaryTree
{
public int CountNodes(TreeNode root)
{
int count = 0;
TreeNode current = root;
while (current != null )
{
if (current.left == null )
{
count++;
current = current.right;
}
else
{
TreeNode predecessor = current.left;
while (predecessor.right != null &&
predecessor.right != current)
{
predecessor = predecessor.right;
}
if (predecessor.right == null )
{
predecessor.right = current;
current = current.left;
}
else
{
predecessor.right = null ;
count++;
current = current.right;
}
}
}
return count;
}
public static void Main( string [] args)
{
TreeNode root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
BinaryTree binaryTree = new BinaryTree();
int nodeCount = binaryTree.CountNodes(root);
Console.WriteLine( "Number of nodes in the binary tree: " + nodeCount);
}
}
|
Javascript
class TreeNode {
constructor(value) {
this .val = value;
this .left = null ;
this .right = null ;
}
}
function countNodes(root) {
let count = 0;
let current = root;
while (current !== null ) {
if (current.left === null ) {
count++;
current = current.right;
}
else {
let predecessor = current.left;
while (predecessor.right !== null && predecessor.right !== current) {
predecessor = predecessor.right;
}
if (predecessor.right === null ) {
predecessor.right = current;
current = current.left;
}
else {
predecessor.right = null ;
count++;
current = current.right;
}
}
}
return count;
}
const root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
const nodeCount = countNodes(root);
console.log( "Number of nodes in the binary tree: " + nodeCount);
|
Output
('Number of nodes in the binary tree:', 5)
Time Complexity: O(N), where N is the number of nodes in the binary tree
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...