Java Program to Create a File in a Specified Directory
Last Updated :
13 Nov, 2023
Creating a file in a specific directory using Java can be done in various ways. This is done using predefined packages and Classes. In this article, we will learn about how to create a file in a specified directory.
Methods to Create a File in a Specified Directory
Three such methods to create a file in a specified directory are mentioned below:
- File.createNewFile() method
- FileOutputStream class
- File.createFile() method
While doing development it is essential to create files, read the files, and perform many other operations. Therefore it becomes very crucial to learn how files can be created in a specific directory.
1. File.createNewFile() Method
This is a very simple method to create a file in a specific directory using Java. On creating a file successfully it returns true and if the file is not created due to an error it will return false. For exception handling (To avoid IOException) we will use try-catch block in the program.
Below is the implementation of the mentioned method:
Java
import java.io.File;
import java.io.IOException;
public class GFG {
public static void main(String[] args) {
String directoryPath = "C:/GFG/Java createfile/" ;
String fileName = "newfile.txt" ;
File file = new File(directoryPath + fileName);
try {
boolean isFileCreated = file.createNewFile();
if (isFileCreated) {
System.out.println( "File created successfully." );
}
else {
System.out.println( "File already exists or an error occurred." );
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
File created successfully.
After successfully running the code the file will be created in specified directory.
Screenshot Showing the File Created:
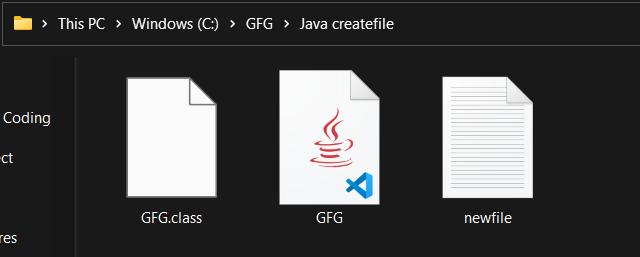
2. FileOutputStream Class
The second method to create a file is using FileOutputStream Class. Using this method we get more control over the file in terms of content.
Below is the implementation of the mentioned method:
Java
import java.io.FileOutputStream;
import java.io.IOException;
public class GFG {
public static void main(String[] args) {
String directoryPath = "C:/GFG/Java createfile/" ;
String fileName = "newfile2.txt" ;
try {
FileOutputStream fileOutputStream = new FileOutputStream(directoryPath + fileName);
fileOutputStream.close();
System.out.println( "File created successfully." );
}
catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
File created successfully.
Screenshot Showing the File Created:
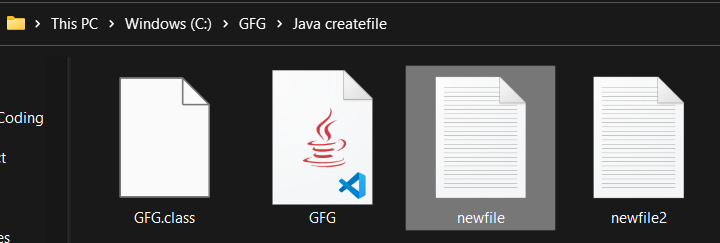
3. File.createFile() Method
Using java.nio.file package this method is alternative to create a file. More file handling capabilities are provided by this method.
Below is the implementation of the mentioned method:
Java
import java.io.IOException;
import java.nio.file.FileAlreadyExistsException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class GFG {
public static void main(String[] args) {
String directoryPath = "C:/GFG/Java createfile/" ;
String fileName = "newfile3.txt" ;
Path filePath = Paths.get(directoryPath, fileName);
try {
Files.createFile(filePath);
System.out.println( "File created successfully." );
}
catch (FileAlreadyExistsException e) {
System.out.println( "File already exists." );
}
catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
File created successfully.
Screenshot Showing the File Created:
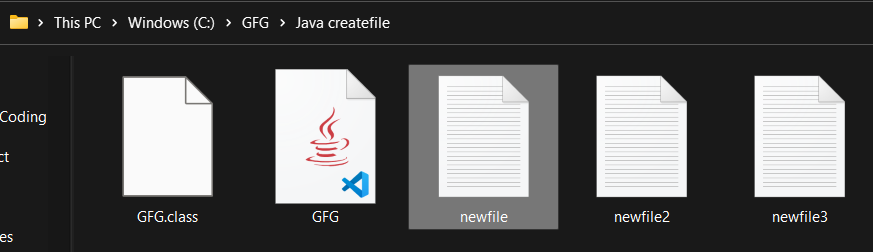
Conclusion
In Java programming creating file in specified directory is very easy and common operation. It can be done in three ways as discussed earlier. This methods can be selected on various factors like requirements and all. The above discussed techniques can be used to create file in specified directory.
Share your thoughts in the comments
Please Login to comment...