Building A Weather CLI Using Python
Last Updated :
27 Nov, 2023
Trying to know the weather is something that we all do, every day. But have you ever dreamed of making a tool on your own that can do the same? If yes, then this article is for you. In this article, we will look at a step-by-step guide on how to build a Weather CLI using OpenWeather API.
What is OpenWeather API?
OpenWeather API is a weather API provided by the OpenWeather Ltd. The API provides access to weather conditions at various locations around the globe. From details about temperature to humidity to real files, the API has its call covered, making it a prime choice for most developers. Additionally, it also has a free-to-use tier and some paid options that offer better up-time and features. The best thing is that this API can be used using a simple HTTP request, meaning it can be easily used in any language.
Modules Required:
When it comes to developing a weather CLI in python we need python requests module. Python requests module allows us to make HTTP requests directly from the language. Since we will be sending requests to OpenWeather API for this project, we need to make sure requests module is installed. We also need an OS module, but that is installed by default, so we do not need to install it again.
pip install requests
Building A Weather CLI Using Python
Below are the steps to implement a weather CLI:
- First we imported all the required modules, these modules are json, requests and os
- After this we stored our API_KEY and designed a base URL to make requests
- Verified if the city exists or not, and handled it accordingly
- Store all the details received from the OpenWeather API
- Printed all these details on the console
- Runs the shell command to get weather information from wttr.in
Example
In this example, we have created a python file named weather.py
Python3
import requests
import json
import os
key = "OPENWEATHER_API_KEY"
loc = input ( "Enter city/state name : " )
url = baseUrl + "appid=" + key + "&q=" + loc
response = requests.get(url)
res = response.json()
if (res[ "cod" ] = = "404" ):
print ( " Location Not Found " )
else :
tempK = res[ "main" ][ "temp" ]
feelK = res[ "main" ][ "feels_like" ]
pres = res[ "main" ][ "pressure" ]
humi = res[ "main" ][ "humidity" ]
desc = res[ "weather" ][ 0 ][ "description" ]
tempC = tempK - 273.15
feelC = feelK - 273.15
pres * = 0.0009869233
print ( " Temperature = " + str ( round (tempC, 2 )) + " °C" )
print ( " Feels Like = " + str ( round (feelC, 2 )) + " °C" )
print ( " Pressure = " + str ( round (pres, 2 )) + " atm" )
print ( " Humidity = " + str (humi) + " %" )
print ( " Description = " + str (desc))
print (os.popen(f "curl wttr.in/{loc}" ).read())
|
Output
Now, run the code and look at the output or use the terminal and run the following command
python3 weather.py
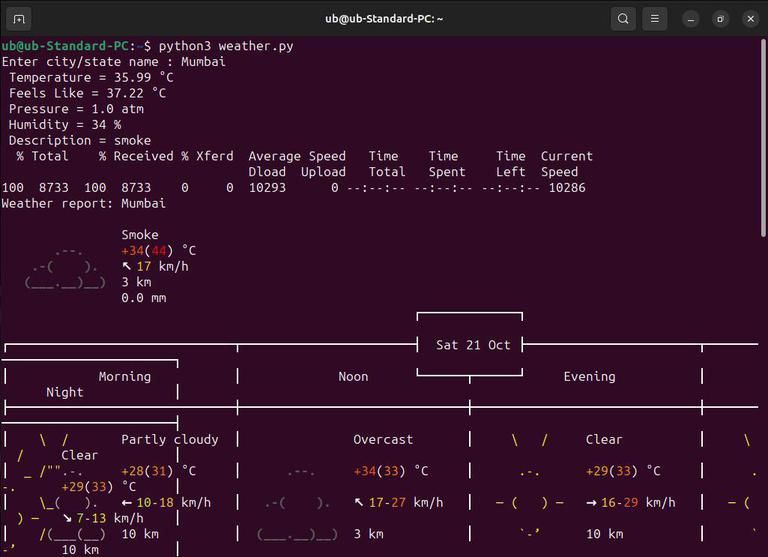
Weather CLI in action
Conclusion
So, at the end we have successfully managed to make a CLI based weather app in Python that can tell us about the weather conditions, temperature, humidity and a plethora of other details. With use of OpenWeather API’s and some Python modules we were able to make this project happen. But this is not the end, you can also go one step ahead, and extend this project even further and add more features or use this knowledge to learn more about other amazing APIs and python modules. At the end, the only limit is your imagination. So keep learning.
Share your thoughts in the comments
Please Login to comment...