FastAPI – Crud Operations
Last Updated :
06 Dec, 2023
We will explore how to implement CRUD operations with FastAPI. CRUD operations are essential in any web application, including creating new records, retrieving existing records, updating existing records, and deleting records from a database.
What is CRUD in FastAPI?
CRUD refers to the basic operations that can be performed on data in a database. It stands for Create, Read, Update, and Delete, and it represents the fundamental functionalities that are essential for managing data in most applications.
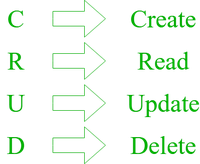
CRUD
Create: It involves adding new data to the database. In FastAPI, we can create data by sending a POST request to the appropriate endpoint. For example, to add a new entry to the database, we would send a POST request to the creation endpoint with the relevant details in the request body.
Read: It involves retrieving existing data from the database. In FastAPI, we can perform Read operations using GET requests. For example, if we want to retrieve any entry from a database, we would send a GET request to the respective endpoint.
Update: It involves updating existing data in the database. In FastAPI we can perform an Update operation using PUT/PATCH, PUT allows us to update the entire database whereas PATCH allows us to modify specific fields in the database. For example, if we want to update any attribute of the user we would send a PUT/PATCH request to the endpoint with new details.
Delete: It involves deleting existing data from the database. In FastAPI we can perform a Delete Operation using DELETE requests. For example, if we want to delete any entry of a user we would send a DELETE request to the endpoint.
Requirements
To implement the CRUD operations in FastAPI we need the following :
- SQLAlchemy: SQLAlchemy is a popular SQL toolkit and Object Relational Mapper. It is written in Python and gives full power and flexibility of SQL to an application developer.
- Uvicorn: Uvicorn is an ASGI (Asynchronous Server Gateway Interface) web server implementation for Python used to manually run our server. As FastAPI doesn’t have it’s own development server we use this high-performance and asyncio-friendly web server to run our API on localhost.
- SwaggerUI: SwaggerUI is an Open-Source tool to visualize or test RESTful APIs. It uses an existing JSON or YAML document and makes it interactive. It creates a platform that arranges each of our methods (GET, PUT, POST, DELETE) and categorizes our operations. Each method is expandable, and within them we can find a full list of parameters with their corresponding examples.
Implementing CRUD operations with FastAPI
Step 1: Make sure your system has Python installed if not follow this article.
Step 2: Install FastAPI, Uvicorn and SQLalchemy using following command.
pip install fastapi uvicorn sqlalchemy
Step 3: Create new file, name main.py
main.py: This code creates a FastAPI web app with SQLAlchemy for CRUD operations on an “items” table in a SQLite database. It provides four endpoints for Create, Read, Update, and Delete operations. The app can be run using uvicorn
.
Python3
import uvicorn
from fastapi import FastAPI
from sqlalchemy import Column, Integer, String, create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
engine = create_engine(SQLALCHEMY_DATABASE_URL)
SessionLocal = sessionmaker(autocommit = False , autoflush = False , bind = engine)
Base = declarative_base()
class Item(Base):
__tablename__ = "items"
id = Column(Integer, primary_key = True , index = True )
name = Column(String, index = True )
description = Column(String, index = True )
Base.metadata.create_all(bind = engine)
app = FastAPI()
@app .post( "/items/" )
async def create_item(name: str , description: str ):
db = SessionLocal()
db_item = Item(name = name, description = description)
db.add(db_item)
db.commit()
db.refresh(db_item)
return db_item
@app .get( "/items/{item_id}" )
async def read_item(item_id: int ):
db = SessionLocal()
item = db.query(Item). filter (Item. id = = item_id).first()
return item
@app .put( "/items/{item_id}" )
async def update_item(item_id: int , name: str , description: str ):
db = SessionLocal()
db_item = db.query(Item). filter (Item. id = = item_id).first()
db_item.name = name
db_item.description = description
db.commit()
return db_item
@app .delete( "/items/{item_id}" )
async def delete_item(item_id: int ):
db = SessionLocal()
db_item = db.query(Item). filter (Item. id = = item_id).first()
db.delete(db_item)
db.commit()
return { "message" : "Item deleted successfully" }
if __name__ = = "__main__" :
uvicorn.run(app)
|
Testing
To run the application using uvicorn use the command:
uvicorn test:app Â
Output:
.png)
Now let’s start with testing of our API using SwaggerUI. Open new tab in your browser and type http://127.0.0.1:8000/docs
-768.png)
POST Request
Click on POST which will allows us to add items, then click on ‘Try it out‘ top right corner. In request body enter data then click on execute.
.png)
Create
GET Request:
On the same page goto GET to retrieve data, then click of ‘Try it out‘. Then click on execute, below in server response you will get all retrieved data. We can also check our users from our 1st tab i.e http://127.0.0.1:8000/items/1. If no changes are reflected try to reload the tab.
.png)
Read
PUT Request:
To update your existing user details go to PUT then, enter the index of user present in the list and update details in request body. To check if again use GET method or just go to your path.
.png)
Update
DELETE Request:
To delete your existing user, go to DELETE then, enter the index of user present in the list and click execute. Again check if user got deleted or not. You can also in Reponse Body where you will get message like “Item deleted successfully”
.png)
Delete
Conclusion
Therefore now we can conclude that we can perform CRUD operations using FastAPI which is micro-web-framerwork of python for API development with SQLalchemy for database in which we used Uvicorn for running the API and SwaggerUI for testing our API.
Share your thoughts in the comments
Please Login to comment...